Please help me identify which is polymorphism and inheritance in the program, if none, please add. Other comments on other code blocks will be appreciated. Lastly, display the information of the vehicle/s. Thanks lot. ————- public class Vehicle { public Vehicle() { } protected string _type; protected int_door; protected double _Fuelcapacity; protected double _speed; protected void SetType(string type) { // such as car, bus, motorcycle. _type = type; } protected void SetEngineType(string engineType) { // such as diesel, regular gas, unleaded gas _engineType = engineType; } public void Setdoor(int door) { _door = door; } } public class Car : Vehicle { public double GetKilometrage() { return _speed / 0.62137; } } public class Bus : Vehicle { private int capacity; public int Capacity { get { return capacity; } set { Capacity = value; } } public double Getspeed() { return _speed; // comes from the base class } public int GetCapacity() { return Capacity; } } static void Main(string[] args) { Car myCar = new Car(); myCar.setspeed(25000); Console.WriteLine(myCar.GetKilometrage().ToString()); Console.ReadKey(); }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Please help me identify which is polymorphism and inheritance in the program, if none, please add. Other comments on other code blocks will be appreciated. Lastly, display the information of the vehicle/s. Thanks lot.
————-
public class Vehicle
{
public Vehicle()
{ }
protected string _type;
protected int_door;
protected double _Fuelcapacity;
protected double _speed;
protected void SetType(string type)
{
// such as car, bus, motorcycle.
_type = type;
}
protected void SetEngineType(string engineType)
{
// such as diesel, regular gas, unleaded gas
_engineType = engineType;
}
public void Setdoor(int door)
{
_door = door;
}
}
public class Car : Vehicle
{
public double GetKilometrage()
{
return _speed / 0.62137;
}
}
public class Bus : Vehicle
{
private int capacity;
public int Capacity
{
get
{
return capacity;
}
set
{
Capacity = value;
}
}
public double Getspeed()
{
return _speed; // comes from the base class
}
public int GetCapacity()
{
return Capacity;
}
}
static void Main(string[] args)
{
Car myCar = new Car();
myCar.setspeed(25000);
Console.WriteLine(myCar.GetKilometrage().ToString());
Console.ReadKey();
}

Step by step
Solved in 3 steps with 1 images

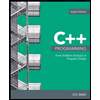
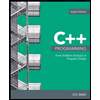