In this lab, you complete a partially written Java program that includes methods that require multiple parameters (arguments). The program prompts the user for two numeric values. Both values should be passed to methods named calculateSum(), calculateDifference(), and calculateProduct(). The methods compute the sum of the two values, the difference between the two values, and the product of the two values. Each method should perform the appropriate computation and display the results. The source code file provided for this lab includes the variable declarations and the input statements. Comments are included in the file to help you write the remainder of the program. My code: import java.util.Scanner; public class Computation { public static void main(String args[]) { double value1; String value1String; double value2; String value2String; Scanner input = new Scanner(System.in); System.out.println("Enter the first numeric value: "); value1String = input.nextLine(); value1 = Double.parseDouble(value1String); System.out.println("Enter second numeric value: "); value2String = input.nextLine(); value2 = Double.parseDouble(value2String); // Call calculateSum() here System.out.println(calculateSum(value1,value2)); // Call calculateDifference() here System.out.println(calculateDifference(value1,value2)); // Call calculateProduct() here System.out.println(calculateProduct(value1,value2)); System.exit(0); } // End of main() method. // Write calculateSum() method here. public static double calculateSum(double a,double b){ return a + b; } // Write calculateDifference() method here. public static double calculateDifference(double a,double b){ return a - b; } // Write calculateProduct() method here. public static double calculateSum(double a,double b){ return a*b; } } // End of Computation class.
I already written a code but this error keeps on showing up, I don't know what's wrong with it. Programming language used is Java. If my code is messy, please debug it. Thank you.
Problem:
In this lab, you complete a partially written Java program that includes methods that require multiple parameters (arguments). The program prompts the user for two numeric values. Both values should be passed to methods named calculateSum(), calculateDifference(), and calculateProduct(). The methods compute the sum of the two values, the difference between the two values, and the product of the two values. Each method should perform the appropriate computation and display the results. The source code file provided for this lab includes the variable declarations and the input statements. Comments are included in the file to help you write the remainder of the program.
My code:
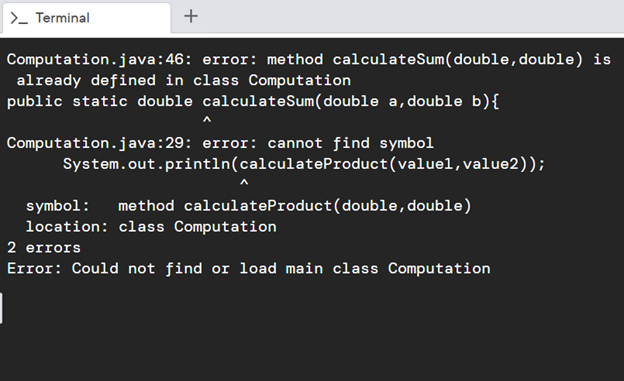

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

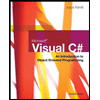
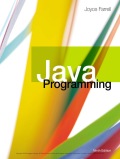
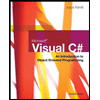
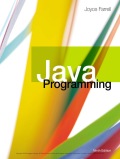