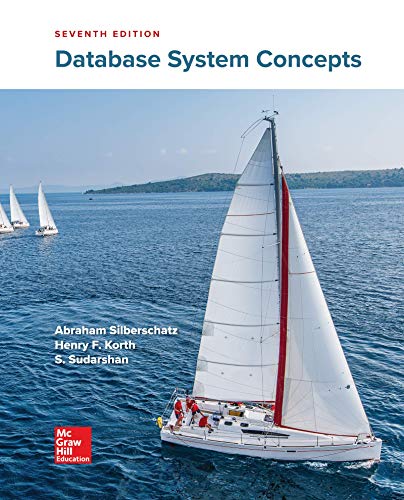
Concept explainers
I need help in Java code please.
This is the question:
Creating a JAVA program that should implement a method that verifies whether a given 4 x 4 square is a Magic Square with a given magicValue. Also, adding code to generate values for a Magic Square based on a magicValue.
And below is the code. This code is run but it is just qualified the requirement which is to verify whether a given 4 x 4 square (containing 16 values) is a Magic Square with a given magic value. The other requirement is to generate values for a Magic Square based on a magicValue, when I check the executed magic square, the sum of the numbers in rows and columns is not equal to the magic value
import java.util.*;
import java.awt.*;
public class MagicSquare{
static int checkMag(int mat[][],int M)
{
// Function for checking Magic square
int i, j,n=4; int sum=0; // filling matrix with its count value // starting from 1;
for ( i = 0; i < n; i++) {
for ( j = 0; j < n; j++)
sum=sum+mat[i][j];
if(sum!=M)
{
return 0;
}
sum=0;
}
for ( i = 0; i < n; i++) {
for ( j = 0; j < n; j++)
sum=sum+mat[j][i];
if(sum!=M)
{
return 0;
}
sum=0;
}
int sumR=0,
sumL=0;
for ( i = 0; i < n; i++) {
for ( j = 0; j < n; j++) {
if(i==j) sumL=sumL+mat[i][j];
if((i+j)==(n-1))
sumR=sumR+mat[i][j]; } }
if(sumR!=M )
{
return 0;
}
if(sumL!=M)
{
return 0;
}
return 1;
}
static int[][] builtMagic(int mat[][],int M,int Mnew) {
int i,j,n=4;
Mnew=Mnew-M;
int rem=Mnew%4;
int quo =Mnew/4;
if(rem==0){ for ( i = 0; i < n; i++) {
for ( j = 0; j < n; j++)
mat[i][j] =mat[i][j]+quo; }}
else{
for ( i = 0; i < n; i++) {
for ( j = 0; j < n; j++) {
mat[i][j] =mat[i][j]+quo;
if(mat[i][j]==13||mat[i][j]==14||mat[i][j]==15||mat[i][j]==16) {
mat[i][j] =mat[i][j]+rem;
}
}
}
}
return mat;
}
public static void main (String[] args) {
//Scanner object
Scanner sc = new Scanner(System.in);
DrawingPanel panel = new DrawingPanel(500, 500);
Graphics g = panel.getGraphics();
// creating a 2d array of magic square numbers
int numbers[][] = { { 8, 11, 14, 1 }, { 13, 2, 7, 12 }, { 3, 16, 9, 6 }, { 10, 5, 4, 15 } };
// drawing grid of cell width = 80 centered at panel center
drawGrid(g, panel.getWidth() / 2, panel.getHeight() / 2,numbers.length, 80);
// drawing numbers
//drawNumbers(g, panel.getWidth() / 2, panel.getHeight() / 2, 80, numbers);
// drawing title string centered at y=50
drawString(g, "CSC 142 Magic Square", panel.getWidth() / 2, 50);
int n = 4;
int magMat[][]= new int[n][n];
int M;
int baseMat[][]={{12,6,15,1},{13,3,10,8},{2,16,5,11},{7,9,4,14}};
for (int k=0;k<10;k++){
System.out.println("Enter magic value");
M=sc.nextInt();
System.out.println( "Enter " + ( n*n ) + " values" );
for(int i=0;i<n;i++) {
for(int j=0;j<n;j++)
{
magMat[i][j]= sc.nextInt();
}
}
if(checkMag(magMat,M)==1) {
System.out.println("It is a magic square");
panel.clear();
makeMagicSquareGrid(panel,magMat,k+1); // insert magic m=numbers on grid
}
else {
System.out.println("It is not a magic square"); }
System.out.println("Enter another magic value greater than 34");
int Mnew=sc.nextInt();
magMat=builtMagic(magMat,34,Mnew);
panel.clear();
makeMagicSquareGrid(panel,magMat,k+1); // insert magic m=numbers on grid
}
}
static void makeMagicSquareGrid(DrawingPanel dp,int[][] magMat, int k){
Graphics g = dp.getGraphics();
drawGrid(g, dp.getWidth() / 2, dp.getHeight() / 2,magMat.length, 80);
// drawing numbers
drawNumbers(g, dp.getWidth() / 2, dp.getHeight() / 2, 80, magMat);
// drawing title string centered at y=50
drawString(g, "Magic Square "+k, dp.getWidth() / 2, 50);
String filename="MagicMatrix"+k+".jpeg";
try{
dp.save(filename);
}
catch(Exception e)
{
System.out.println("File coudn't be saved");
}
}
public static void drawGrid(Graphics g, int centerX, int centerY, int row, int cell) { // cell width
int x = centerX - (row / 2) * cell;
int y = centerY - (row / 2) * cell;
// setting black color
g.setColor(Color.BLACK);
// looping for each row and column
for (int i = 0; i < row; i++) {
for (int j = 0; j < row; j++) {
// drawing a square with equal sides
g.drawRect(x + (cell * j), y + (cell * i),cell, cell);
}
}
}
// method to draw the numbers (given in a 2d array) inside a grid (assuming
// grid is previously drawn)
public static void drawNumbers(Graphics g, int centerX, int centerY,int cell, int numbers[][]) {
int x = centerX - (numbers.length / 2) * cell;
int y = centerY - (numbers.length / 2) * cell; //Setting red color
g.setColor(Color.RED); // Customizing font
g.setFont(new Font("Georgia", Font.BOLD, 25));
for (int i = 0; i < numbers.length; i++) {
for (int j = 0; j < numbers.length; j++) {
// Setting center cell
int xx = x + (cell * j) + cell / 2;
int yy = y + (cell * i) + cell / 2;
// Setting center text
drawString(g, String.valueOf(numbers[i][j]), xx, yy);
}
}
} public static void drawString(Graphics g, String text, int centerX, int centerY) {
// getting FontMetrics of current font
FontMetrics metrics = g.getFontMetrics(g.getFont());
// finding width of text in pixels using metrics
int width = metrics.stringWidth(text);
// drawing string center aligned
g.drawString(text, centerX - width / 2, centerY + metrics.getHeight()/ 2);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Write a program called LoopMethod (main method) that contains a method called printNums (method name) that prints the numbers from a specified start to end value. a) The main method will do the following: • asks the user for start and end number (int type). • calls the printNums methodb) The printNums method will do the following: • receives start and end number (parameters)• prints from the start to the end number using a loop Sample Run: Input start number: 3Input end number: 93 4 5 6 7 8 9Input start number: 9Input end number: 219 10 11 12 13 14 15 16 17 18 19 20 21arrow_forwardPlease use java only. In this assignment, you will implement a simple game in a class called SimpleGame. This game has 2 options for the user playing. Based on user input, the user can choose to either convert time, from seconds to hours, minutes, and seconds, or calculate the sum of all digits in an integer. At the beginning of the game, the user will be prompted to input either 1 or 2, to indicate which option of the game they want to play. 1 will indicate converting time, and 2 will indicate calculating the sum of digits in an integer. For converting time, the user will be prompted to input a number of seconds (as an int) and the program will call a method that will convert the seconds to time, in the format hours:minutes:seconds, and print the result. For example, if the user enters 6734, the program will print the time, 1:52:14. As another example, if the user enters 10,000, the program should print 2:46:39. For calculating the sum of digits in an integer, the user will be…arrow_forwardCan you do it in Javaarrow_forward
- change Summation Integers problem to Finding the minimumproblem. Make sure you properly wrote/updated all text messages, method names,and math calculations.Hint: You can use java.lang.Math.min() method.Example: System.out.printf("The min of the integers %4d and %4d and %4dis %7d\n", a, b, c, MinTest(a, b, c));arrow_forwardWrite a Java method that computes the future investment value at a given interest rate for a specified number of years. Your method should return the future investment value after calculation. The future investment is determined using the formula below: futurelnvestmentValue = investmentAmount * (1+monthlylnterestRate)numberOfYears*12 You can use the following method header: public static double futurelnvestmentValue (double investmentAmount, double monthlylnterestRate, int years);arrow_forwardDefine a method printDate, with int parameters year and day, and String parameter month, that prints using Gregorian "Month DD, YYYY" format. For example, printDate (2020, 21, "October") prints October 21, 2020. Write the code of the method in the box below.arrow_forward
- The language is Java. The methods in the pictures must be used.arrow_forwardchange Summation Integers problem to Finding the minimumproblem. Make sure you properly wrote/updated all text messages, method names,and math calculations.Hint: You can use java.lang.Math.min() method.Example: System.out.printf("The min of the integers %4d and %4d and %4dis %7d\n", a, b, c,d MinTest(a, b, c,d)); fix below code structurearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
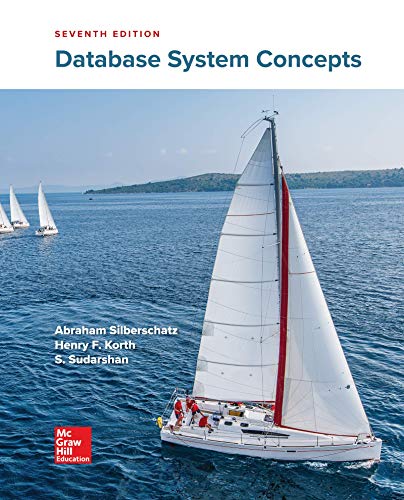
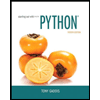
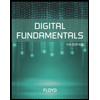
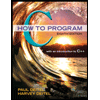
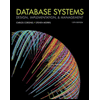
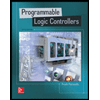