In this question, we will bake a class. Create a class Cake, with the following instance attributes: • name (a string) • ingreds (a list of strings) • price (a float) The Cake class has also the following methods: • Write a constructor that takes as input a string indicating the cake name, and a list of strings indicating the cake ingredients. (No docstring needed.) The constructor uses these values to initialize the attributes accordingly. Note that each cake should be assigned a random price between $10.00 (inclusive) and $15.00 (exclusive). You may import random. Moreover, a cake must contain at least three or more ingredients. If this is not the case, the constructor should raise a ValueError. For this constructor, do not worry about making a copy of the input array. You can simply copy the reference received as input into the corresponding attribute. 1.
In this question, we will bake a class. Create a class Cake, with the following instance attributes: • name (a string) • ingreds (a list of strings) • price (a float) The Cake class has also the following methods: • Write a constructor that takes as input a string indicating the cake name, and a list of strings indicating the cake ingredients. (No docstring needed.) The constructor uses these values to initialize the attributes accordingly. Note that each cake should be assigned a random price between $10.00 (inclusive) and $15.00 (exclusive). You may import random. Moreover, a cake must contain at least three or more ingredients. If this is not the case, the constructor should raise a ValueError. For this constructor, do not worry about making a copy of the input array. You can simply copy the reference received as input into the corresponding attribute. 1.
Chapter9: Using Classes And Objects
Section: Chapter Questions
Problem 11E: a. Write a FractionDemo program that instantiates several Fraction objects and demonstrates that...
Related questions
Question
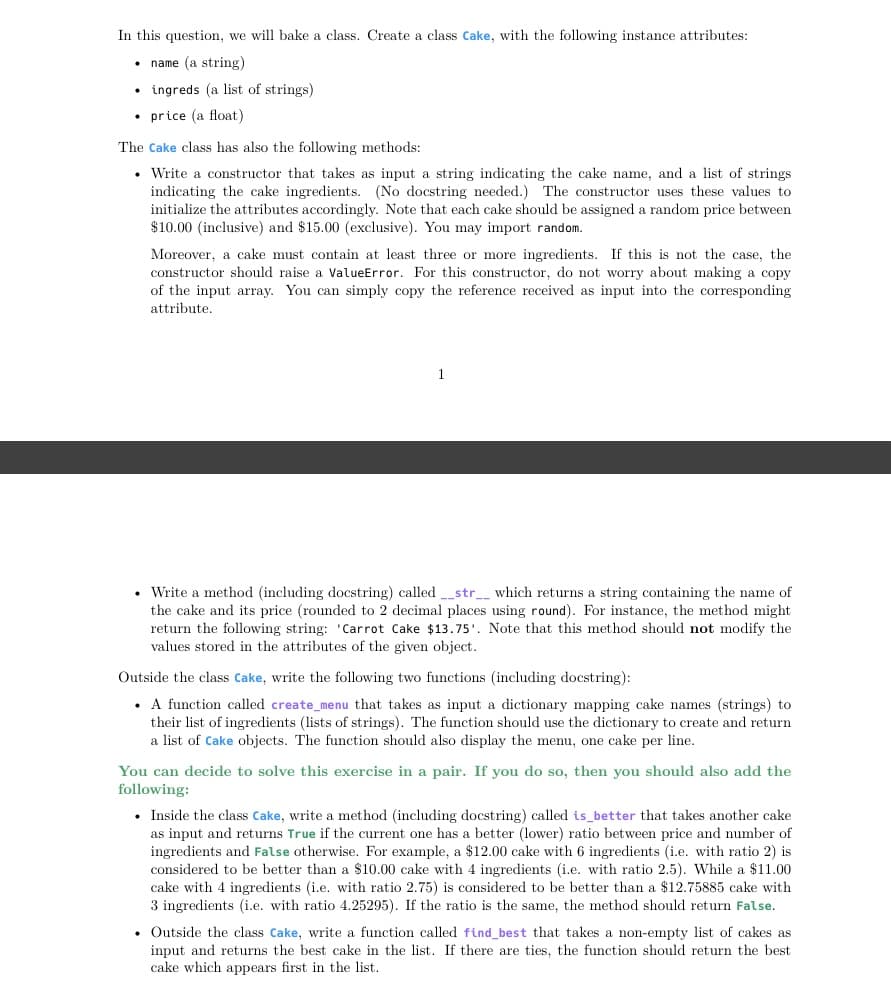
Transcribed Image Text:In this question, we will bake a class. Create a class Cake, with the following instance attributes:
• name (a string)
• ingreds (a list of strings)
• price (a float)
The Cake class has also the following methods:
• Write a constructor that takes as input a string indicating the cake name, and a list of strings
indicating the cake ingredients. (No docstring needed.) The constructor uses these values to
initialize the attributes accordingly. Note that each cake should be assigned a random price between
$10.00 (inclusive) and $15.00 (exclusive). You may import random.
Moreover, a cake must contain at least three or more ingredients. If this is not the case, the
constructor should raise a ValueError. For this constructor, do not worry about making a copy
of the input array. You can simply copy the reference received as input into the corresponding
attribute.
1
• Write a method (including docstring) called _str__ which returns a string containing the name of
the cake and its price (rounded to 2 decimal places using round). For instance, the method might
return the following string: 'Carrot Cake $13.75'. Note that this method should not modify the
values stored in the attributes of the given object.
Outside the class Cake, write the following two functions (including docstring):
• A function called create_menu that takes as input a dictionary mapping cake names (strings) to
their list of ingredients (lists of strings). The function should use the dictionary to create and return
a list of Cake objects. The function should also display the menu, one cake per line.
You can decide to solve this exercise in a pair. If you do so, then you should also add the
following:
• Inside the class Cake, write a method (including docstring) called is_better that takes another cake
as input and returns True if the current one has a better (lower) ratio between price and number of
ingredients and False otherwise. For example, a $12.00 cake with 6 ingredients (i.e. with ratio 2) is
considered to be better than a $10.00 cake with 4 ingredients (i.e. with ratio 2.5). While a $11.00
cake with 4 ingredients (i.e. with ratio 2.75) is considered to be better than a $12.75885 cake with
3 ingredients (i.e. with ratio 4.25295). If the ratio is the same, the method should return False.
• Outside the class Cake, write a function called find_best that takes a non-empty list of cakes as
input and returns the best cake in the list. If there are ties, the function should return the best
cake which appears first in the list.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
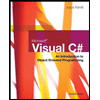
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
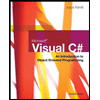
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,