What data would you put together into a struct so that it would make that program easier to manage and make the data on it easier to manage. C++
Addition of Two Numbers
Adding two numbers in programming is essentially the same as adding two numbers in general arithmetic. A significant difference is that in programming, you need to pay attention to the data type of the variable that will hold the sum of two numbers.
C++
C++ is a general-purpose hybrid language, which supports both OOPs and procedural language designed and developed by Bjarne Stroustrup. It began in 1979 as “C with Classes” at Bell Labs and first appeared in the year 1985 as C++. It is the superset of C programming language, because it uses most of the C code syntax. Due to its hybrid functionality, it used to develop embedded systems, operating systems, web browser, GUI and video games.
What data would you put together into a struct so that it would make that program easier to manage and make the data on it easier to manage. C++
#include<iostream>
#include<cmath>
#include<limits>
//Define constants Pi
#define PI 3.14
using namespace std;
//Define a function to calculate the radius
double calculateRadius(int x1,int y1,int x2,int y2)
{
//Compute radius
double radius = sqrt(pow(x2-x1, 2) + pow((y2-y1), 2));
//Return radius to calling function
return radius;
}
//Define a function to calculate Area
double calculateArea(double radius)
{
//Return area to calling function
return PI * radius * radius;
}
//Define a function to calculate perimeter
double calculatePeriemeter(double radius)
{
//Return perimeter to calling function
return 2*PI*radius;
}
int main()
{
//Variables declaration
int x1, y1, x2, y2;
double radius, area, perimeter;
cout<<"\nPlease enter the center point in the form x y: ";
cin>>x1>>y1;
while(1)
{
if(cin.fail())
{
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(),'\n');
cout<<"You entered something that is not a number. Please try again"<<endl;
cout<<"\nPlease enter the center point in the form x y: ";
cin>>x1>>y1;
}
if(!cin.fail())
break;
}
//Prompt the user to enter the point on the circle in the form x y
cout<<"Please enter the point on the circle in the form x y: ";
cin>>x2>>y2;
while(1)
{
if(cin.fail())
{
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(),'\n');
cout<<"You entered something that is not a number. Please try again"<<endl;
cout<<"\nPlease enter the point on the circle in the form x y: ";
cin>>x2>>y2;
}
if(!cin.fail())
break;
}
//call to functions and store the return values to variables
radius = calculateRadius(x1, y1, x2, y2);
area = calculateArea(radius);
perimeter = calculatePeriemeter(radius);
//display the outputs
cout<<"Here is the information for the circle formed from ("<<x1<<","<<y1<<") and ("<<x2<<","<<y2<<")"<<endl;
cout<<"Radius: "<<radius<<endl;
cout<<"Area: "<<area<<endl;
cout<<"Perimeter: "<<perimeter<<endl;
return 0;
}

Step by step
Solved in 3 steps with 1 images

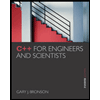
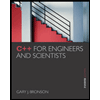