Interquartile Range Quartiles are used in statistics to classify data. Per their name, they divide data into quarters. Given a set of data: [1, 2, 3, 4, 5, 6, 7] The lower quartile (Q1) would be the value that separates the lowest quarter of the data from the rest of the data set. So, in this instance, Q1 = 2. The middle quartile (also known as the median or Q2) separates the lowest 2 quarters of the data from the rest of the data set. In this case, Q2 = 4. The upper quartile (Q3) separates the lowest 3 quarters of the data from the rest of the data set. In this case, Q3 = 6. The interquartile range (IQR) is the difference between the third quartile and the first quartile: Q3 - Q1. In case the number of values in the list are odd, the central element is a unique element. Example, if the list has size = 9. The fifth element in the list will be the median. In case the number of values in the list are even, the central element is a average of two elements. Example, if the list has size = 10. The average of fifth and sixth element in the list will be the median. Q1 is the median of the beginning and the element preceding median, and Q3 is the median of the element succeeding median and the end. Another example, if the data were [1, 2, 3, 4] • Q2 = Average of 2 and 3 = 2.5 • Q1 = List consisting of elements: 1, 2 (everything before median) = Average of 1 and 2 = 1.5 • Q3 = List consisting of elements: 3, 4 (everything after median) = Average of 3 and 4 = 3.5 • IQR = 3.5-1.5 = 2.00 Problem Statement Given a sorted singly linked list without a tail (e.g, head -> 1-> 2 -> 3 -> 4), return the interquartile range of the data set using the slow and fast pointer approach OR using a methodology that does not iterate over the linked list twice. You must not iterate over the entire linked list more than once and you cannot use arrays, vectors, lists or an STL implementation of List ADT in this problem. If you prohibit the above requirements, you will incur a 20% penalty on your score. The following Node class is already defined for you and we have already implemented the insert() and main() function: class Node { public: }; int value; Node* next nullptr;
Interquartile Range Quartiles are used in statistics to classify data. Per their name, they divide data into quarters. Given a set of data: [1, 2, 3, 4, 5, 6, 7] The lower quartile (Q1) would be the value that separates the lowest quarter of the data from the rest of the data set. So, in this instance, Q1 = 2. The middle quartile (also known as the median or Q2) separates the lowest 2 quarters of the data from the rest of the data set. In this case, Q2 = 4. The upper quartile (Q3) separates the lowest 3 quarters of the data from the rest of the data set. In this case, Q3 = 6. The interquartile range (IQR) is the difference between the third quartile and the first quartile: Q3 - Q1. In case the number of values in the list are odd, the central element is a unique element. Example, if the list has size = 9. The fifth element in the list will be the median. In case the number of values in the list are even, the central element is a average of two elements. Example, if the list has size = 10. The average of fifth and sixth element in the list will be the median. Q1 is the median of the beginning and the element preceding median, and Q3 is the median of the element succeeding median and the end. Another example, if the data were [1, 2, 3, 4] • Q2 = Average of 2 and 3 = 2.5 • Q1 = List consisting of elements: 1, 2 (everything before median) = Average of 1 and 2 = 1.5 • Q3 = List consisting of elements: 3, 4 (everything after median) = Average of 3 and 4 = 3.5 • IQR = 3.5-1.5 = 2.00 Problem Statement Given a sorted singly linked list without a tail (e.g, head -> 1-> 2 -> 3 -> 4), return the interquartile range of the data set using the slow and fast pointer approach OR using a methodology that does not iterate over the linked list twice. You must not iterate over the entire linked list more than once and you cannot use arrays, vectors, lists or an STL implementation of List ADT in this problem. If you prohibit the above requirements, you will incur a 20% penalty on your score. The following Node class is already defined for you and we have already implemented the insert() and main() function: class Node { public: }; int value; Node* next nullptr;
Chapter3: Performing Calculations With Formulas And Functions
Section: Chapter Questions
Problem 3.9CP
Related questions
Question
Need help with a c++ problem, have provided all instructions and code. I have also included the constraints, and you are allowed to use as many if statements and pointers as you want. However, you are only allowed to use one loop. Please write the code in the interQuartile method thank you.
PS: Please remember, there is only one loop allowed, so if you chose to use a while loop, you can only use ONE while loop. Cannot use multiple while loops.
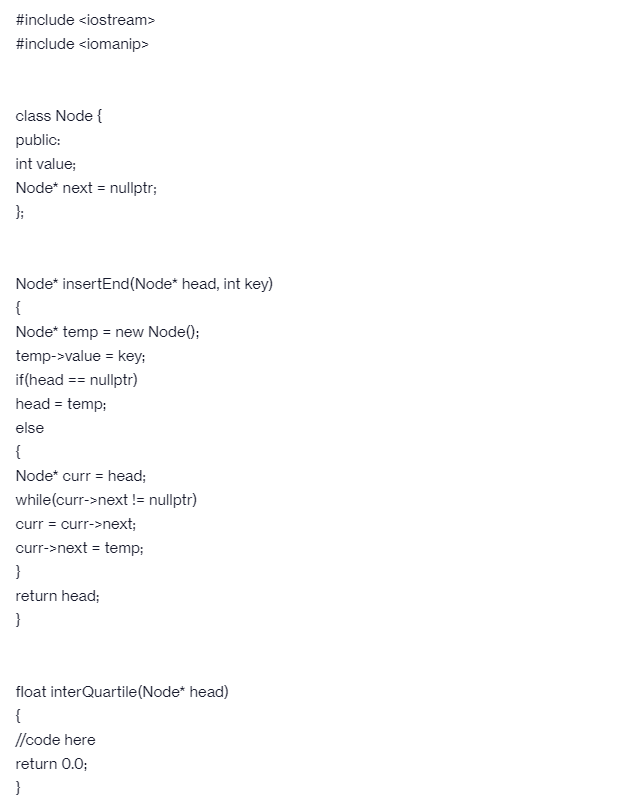
Transcribed Image Text:#include <iostream>
#include <iomanip>
class Node {
public:
int value;
Node* next = nullptr;
};
Node* insertEnd (Node* head, int key)
{
Node* temp = new Node();
temp->value = key;
if(head == nullptr)
head = temp;
else
{
Node* curr = head;
while(curr->next != nullptr)
curr = curr->next;
curr->next = temp;
}
return head;
}
float interQuartile (Node* head)
{
//code here
return 0.0;
}
![Interquartile Range
Quartiles are used in statistics to classify data. Per their name, they divide data into quarters.
Given a set of data: [1, 2, 3, 4, 5, 6, 7]
The lower quartile (Q1) would be the value that separates the lowest quarter of the data from the rest of the data set. So,
in this instance, Q1 = 2. The middle quartile (also known as the median or Q2) separates the lowest 2 quarters of the
data from the rest of the data set. In this case, Q2 = 4. The upper quartile (Q3) separates the lowest 3 quarters of the
data from the rest of the data set. In this case, Q3 = 6. The interquartile range (IQR) is the difference between the third
quartile and the first quartile: Q3 - Q1.
In case the number of values in the list are odd, the central element is a unique element. Example, if the list has size = 9.
The fifth element in the list will be the median. In case the number of values in the list are even, the central element is a
average of two elements. Example, if the list has size = 10. The average of fifth and sixth element in the list will be the
median. Q1 is the median of the beginning and the element preceding median, and Q3 is the median of the element
succeeding median and the end.
Another example, if the data were [1, 2, 3, 4]
Q2 Average of 2 and 3 = 2.5
Q1 = List consisting of elements: 1, 2 (everything before median) = Average of 1 and 2 = 1.5
Q3 = List consisting of elements: 3, 4 (everything after median) = Average of 3 and 4 = 3.5
• IQR 3.5-1.5 = 2.00
Problem Statement
Given a sorted singly linked list without a tail (e.g, head -> 1-> 2 -> 3 -> 4), return the interquartile range of the data set
using the slow and fast pointer approach OR using a methodology that does not iterate over the linked list twice. You
must not iterate over the entire linked list more than once and you cannot use arrays, vectors, lists or an STL
implementation of List ADT in this problem. If you prohibit the above requirements, you will incur a 20% penalty on
your score.
The following Node class is already defined for you and we have already implemented the insert() and main() function:
class Node {
public:
};
int value;
Node* next = nullptr;
Example 1 Input:
2 4 4 5 6 7 8
Example 1 Output:
3.00
Explanation:
Input is a set of numbers inserted into a Linked List separated by spaces.
• The head of this linked list is passed into the interQuartile() function.
Output is the Interquartile Range of the list, a floating point value. IQR = Q3-Q1 = 7-4 = 3.00. We are rounding
returned values to two decimal places in main using setprecision ().
• Note you are expected to return a floating point value. Also, there are a a variety of definitions of IQR and we will
use the definition listed here: https://www.calculatorsoup.com/calculators/statistics/quartile-calculator.php
• You can use the calculator at above link to generate sample test cases.
Constraints
• The list can contain any integers.
• The list will have at least 4 values.
• The list is sorted.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb7b26484-772b-4284-af5a-fa2f3fa080bf%2F0270ea95-4ab2-43e5-9041-e2ad61199ed1%2Fmgt2yk_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Interquartile Range
Quartiles are used in statistics to classify data. Per their name, they divide data into quarters.
Given a set of data: [1, 2, 3, 4, 5, 6, 7]
The lower quartile (Q1) would be the value that separates the lowest quarter of the data from the rest of the data set. So,
in this instance, Q1 = 2. The middle quartile (also known as the median or Q2) separates the lowest 2 quarters of the
data from the rest of the data set. In this case, Q2 = 4. The upper quartile (Q3) separates the lowest 3 quarters of the
data from the rest of the data set. In this case, Q3 = 6. The interquartile range (IQR) is the difference between the third
quartile and the first quartile: Q3 - Q1.
In case the number of values in the list are odd, the central element is a unique element. Example, if the list has size = 9.
The fifth element in the list will be the median. In case the number of values in the list are even, the central element is a
average of two elements. Example, if the list has size = 10. The average of fifth and sixth element in the list will be the
median. Q1 is the median of the beginning and the element preceding median, and Q3 is the median of the element
succeeding median and the end.
Another example, if the data were [1, 2, 3, 4]
Q2 Average of 2 and 3 = 2.5
Q1 = List consisting of elements: 1, 2 (everything before median) = Average of 1 and 2 = 1.5
Q3 = List consisting of elements: 3, 4 (everything after median) = Average of 3 and 4 = 3.5
• IQR 3.5-1.5 = 2.00
Problem Statement
Given a sorted singly linked list without a tail (e.g, head -> 1-> 2 -> 3 -> 4), return the interquartile range of the data set
using the slow and fast pointer approach OR using a methodology that does not iterate over the linked list twice. You
must not iterate over the entire linked list more than once and you cannot use arrays, vectors, lists or an STL
implementation of List ADT in this problem. If you prohibit the above requirements, you will incur a 20% penalty on
your score.
The following Node class is already defined for you and we have already implemented the insert() and main() function:
class Node {
public:
};
int value;
Node* next = nullptr;
Example 1 Input:
2 4 4 5 6 7 8
Example 1 Output:
3.00
Explanation:
Input is a set of numbers inserted into a Linked List separated by spaces.
• The head of this linked list is passed into the interQuartile() function.
Output is the Interquartile Range of the list, a floating point value. IQR = Q3-Q1 = 7-4 = 3.00. We are rounding
returned values to two decimal places in main using setprecision ().
• Note you are expected to return a floating point value. Also, there are a a variety of definitions of IQR and we will
use the definition listed here: https://www.calculatorsoup.com/calculators/statistics/quartile-calculator.php
• You can use the calculator at above link to generate sample test cases.
Constraints
• The list can contain any integers.
• The list will have at least 4 values.
• The list is sorted.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:
9781337508841
Author:
Carey
Publisher:
Cengage