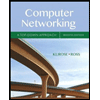
PYTHON
In order to parse data to start a simulation, users must enter data correctly.
Suppose that the simulation requires 2 information from the user, which is called 'soil_key' and 'soil_data.' The user will enter 'soil_key' first, following with 'soil_data.' We need to check if the user enters the 'soil_data' data correctly. All elements in soil data ('soil_data') must be characters defined in the soil keys ('soil_key'). Both soil_key and soil_data are lists. Consider an example:
INPUT:
soil_key = ['C', 'Clay', ' B', 'Bedrock', ' V', 'Void']
soil_data1 = [ ['V', 'C', 'V'], ['C', 'V', ' '], ['B', 'M', 'B'] ]
soil_data2 = [ ['B', 'C', 'V'], ['C', 'V', ' B'], ['B', 'C', 'B'] ]
OUTPUT:
soil_data1: check data again. Parsing failed!
soil_data2: Parsing successfully.
Write a python function that checks if the soil data is being entered correctly. Return True and print 'Parsing successfully' for correct data. Otherwise, return False and tells the user 'check data again. Parsing failed!'

Step by stepSolved in 2 steps with 1 images

- # Create Custom Transformer Create a custom transformer, just as we did in the lecture video entitled "Custom Transformers", that performs two computations: 1. Adds an attribute to the end of the numerical data (i.e. new last column) that is equal to $\frac{x_1^3}{x_5}$ for each observation. In other words, for each instance, you will cube the $x_1$ column and then divide by the $x_5$ column. 2. Drops the entire $x_4$ feature column if the passed function argument `drop_x4` is `True` and doesn't drop the column if `drop_x4` is `False`. (See further instructions below.) You must name your custom transformer class `Assignment4Transformer`. Your class should include an input parameter called `drop_x4` with a default value of `True` that deletes the $x_4$ feature column when its value is `True`, but preserves the $x_4$ feature column when you pass a value of `False`. This transformer will be used in a pipeline. In that pipeline, an imputer will be run *before* this transformer. Keep…arrow_forwardPart 3. arange Method Define a method in simpy named arange. Its purpose is to fill in the values attribute with range of values, like the range built-in function, but in terms of floats. It has three parameters in addition to self, the last being optional: 1. start - a float indicating the first value in the range 2. stop - a float that is not included in the produced range values 3. step - a float whose default value is 1.0 that indicates how to increase (or decrease) each subsequent item in the generated range. Unlike the built- in range function, this can be a fractional, float value. Step cannot be 0.0. Think carefully about what the value of step tells you about how to design your loop(s) for this method. Before any looping, you should assert step != 0.0 to be sure you avoid an infinite loop with an invalid argument. positive = Simpy([]) positive.arange(1.0, 5.0) print("Actual: ", positive, " - Expected: Simpy([1.0, 2.0, 3.0, 4.0])") fractional = Simpy([]) positive.arange (0.0,…arrow_forwardPlease submit a flowchart of your program for your project below. Need a class which will contain: Student Name Student Id Student Grades (an array of 3 grades) A constructor that clears the student data (use -1 for unset grades) Get functions for items a, b, and c, average, and letter grade Set functions for items a, n, and c Note that the get and set functions for Student grades need an argument for the grade index. Need another class which will contain: An Array of Students (1 above) A count of number of students in use You need to create a menu interface that allows you to: Add new students Enter test grades Display all the students with their names, ids, test grades, average, and letter grade Exit the program Add comments and use proper indentation. Nice Features: I would like that system to accept a student with no grades, then later add one or more grades, and when all grades are entered, calculate the final average or grade. I would like the system to display the…arrow_forward
- ✓ Exercises Exercise: Write a method _ and _ that computes the region representing the intersection of two regions. Exercise: Write a method _contains__, which checks whether a n-dimensional point belongs to the region. Remember, a point belongs to the region if it belongs to one of the rectangles in the region. ✓ Membership of a point in a region #@title Membership of a point in a region def region_contains (self, p): ### YOUR SOLUTION HERE Region._contains = region_contains [ ] # Tests 10 points. assert (2, 1) in Region (Rectangle((0, 2), (0, 3)), Rectangle((4, 6), (5,8))) assert (2, 1) not in Region (Rectangle((0, 1), (0, 3)), Rectangle((4, 6), (5, 8))) Exercise: Write a method _le_ for regions such that R <= S if the region R is contained in the region S. You can test this by checking that the difference between R and S is empty.arrow_forward@Build ER diagramarrow_forwardTic Tac Toe SimulatorCreate a GUI application that simulates a game of tic tac toe. Figure 13-35 shows an example of the application’s window. The window shown in the figure uses nine large JLabelcomponents to display the Xs and Os.One approach in designing this application is to use a two-dimensional int array to simulatethe game board in memory. When the user clicks the New Game button, the applicationshould step through the array, storing a random number in the range of 0 through 1 in eachelement. The number 0 represents the letter O, and the number 1 represents the letter X. TheJLabel components should then be updated to display the game board. The application shoulddisplay a message indicating whether player X won, player Y won, or the game was a tie.arrow_forward
- Opengl Help Programming Language: c++ I need help setting coordinate boundries for this program so the shape can't leave the Opengl window. The shape needs to stay visiable.arrow_forwardCodeWorkout Gym Course Q Search kola shreya@ columbusstate.edu Search exercises... X274: Recursion Programming Exercise: Cannonballs X274: Recursion Programming Exercise: Cannonballs Spherical objects, such as cannonballs, can be stacked to form a pyramid with one cannonball at the top, sitting on top of a square composed of four cannonballs, sitting on top of a square composed of nine. cannonballs, and so forth. Given the following recursive function signature, write a recursive function that takes as its argument the height of a pyramid of cannonballs and returns the number of cannonballs it contains. Examples: cannonball(2) -> 5 Your Answwer: 1 public int cannonball(int height) { 3. 4} Check my answer! Reset Next exercise Feedbackarrow_forwardthe only data that should b epresented as numerical values are those that represent numbers. data that represent categories should never be presented as numerical values. TRUE or FALSEarrow_forward
- Computer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forwardYou have the following workspace in MATLAB: Workspace Name Value Beccentricity Egrav_const Binclination Emass_Earth Horbit_period Bradius_Earth E semi_major E spacecraft_loc 6918900 E spacecraft_vel 7.5883e+03 2.8300e-04 6.6730e-11 28.4700 5.9720e+24 95.4320 6378000 6917100 In the box below, fill in the exact command necessary to delete the variable spacecraft_vel from the workspace without deleting any other variables clear spacecraft_vel Question 2 In MATLAB, you are calculating friction forces on five rail materials for a comparison study. One variable you must define is the time traveled on the aluminum rail. Which of the following are appropriate, descriptive variable names that could be used for this variable? Select all that apply. timeAl alum time Alt U aluminum timearrow_forwardPYTHON PLEASE EXPLAIN STEP BY STEPS AND THE OUTPUT School_sum calls the class_sum function: def class_sum(num): return num * 3 def school_sum(m): return class_sum(m) + 3 print(school_sum(5)) print(school_sum(8)) print(school_sum(15)arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
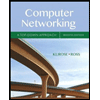
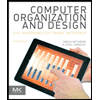
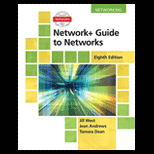
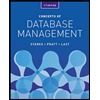
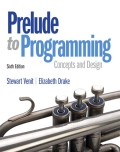
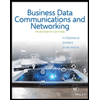