JAVA PROGRAM ASAP The program down below does now work in hypergrade as shown in the screenashot. For test case 1 after ALANTURING\n there need to be nothing. Fro test case 2 after File 'input2.txt' is not found.\n i t wants only Please enter the file name or type QUIT to exit:\n quitENTER. Please modify it or create a new program ASAP BECAUSE it does not pass all the test cases when I upload it to hypergrade. The program must pass the test case when uploaded to Hypergrade. Thank you Chapter 9. PC #16. Morse Code Translator (modified *** Read carefully ***) Morse code is a code where each letter of the English alphabet, each digit, and various punctuation characters are represented by a series of dots and dashes. Write a program that asks the user to enter a file name containing morse code, and then converts that code to text and prints it on the screen. The Morse code table is given in a text file morse.txt. When printing resulting text, display one sentence on each line. There should be no extra spaces at the beginning and at the end of the output. import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.util.HashMap; import java.util.Map; import java.util.Scanner; public class MorseCodeConverter { public static void main(String[] args) { Map morseCodeMap = readMorseCodeTable("Morse.txt"); Scanner scanner = new Scanner(System.in); while (true) { System.out.print("Please enter the file name or type QUIT to exit:\n"); String fileName = scanner.nextLine().trim(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { String text = convertMorseCodeToText(fileName, morseCodeMap); System.out.println(text); } catch (IOException e) { System.out.println("File '" + fileName + "' is not found."); } } scanner.close(); } private static Map readMorseCodeTable(String fileName) { Map morseCodeMap = new HashMap<>(); try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) { String line; while ((line = reader.readLine()) != null) { String[] parts = line.split("\\s+"); if (parts.length == 2) { morseCodeMap.put(parts[1], parts[0]); } } } catch (IOException e) { e.printStackTrace(); } return morseCodeMap; } private static String convertMorseCodeToText(String fileName, Map morseCodeMap) throws IOException { StringBuilder result = new StringBuilder(); try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) { String line; while ((line = reader.readLine()) != null) { String[] morseWords = line.split("\\s{3,}"); for (String morseWord : morseWords) { String[] morseLetters = morseWord.split("\\s+"); for (String morseLetter : morseLetters) { if (morseCodeMap.containsKey(morseLetter)) { result.append(morseCodeMap.get(morseLetter)); } } } result.append("\n"); } } return result.toString().trim(); } } File data down below: Morse.txt 0 ----- 1 .---- 2 ..--- 3 ...-- 4 ....- 5 ..... 6 -.... 7 --... 8 ---.. 9 ----. , --..-- . .-.-.- ? ..--.. A .- B -... C -.-. D -.. E . F ..-. G --. H .... I .. J .--- K -.- L .-.. M -- N -. O --- P .--. Q --.- R .-. S ... T - U ..- V ...- W .-- X -..- Y -.-- Z --.. input1.txt - .... . --- .-. .. --. .. -. .- .-.. --.- ..- . ... - .. --- -. --..-- -.-. .- -. -- .- -.-. .... .. -. . ... - .... .. -. -.- ..--.. .. -... . .-.. .. . ...- . - --- -... . - --- --- -- . .- -. .. -. --. .-.. . ... ... - --- -.. . ... . .-. ...- . -.. .. ... -.-. ..- ... ... .. --- -. .-.-.- .- .-.. .- -. - ..- .-. .. -. --. Test Case 1 Please enter the file name or type QUIT to exit:\n input1.txtENTER THEORIGINALQUESTION,\n CANMACHINESTHINK?\n IBELIEVETOBETOOMEANINGLESSTODESERVEDISCUSSION.\n ALANTURING\n Test Case 2 Please enter the file name or type QUIT to exit:\n input2.txtENTER File 'input2.txt' is not found.\n Please re-enter the file name or type QUIT to exit:\n quitENTER
JAVA PROGRAM ASAP
The program down below does now work in hypergrade as shown in the screenashot. For test case 1 after ALANTURING\n there need to be nothing. Fro test case 2 after File 'input2.txt' is not found.\n i t wants only Please enter the file name or type QUIT to exit:\n
quitENTER.
Please modify it or create a new program ASAP BECAUSE it does not pass all the test cases when I upload it to hypergrade. The program must pass the test case when uploaded to Hypergrade. Thank you
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class MorseCodeConverter {
public static void main(String[] args) {
Map<String, String> morseCodeMap = readMorseCodeTable("Morse.txt");
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Please enter the file name or type QUIT to exit:\n");
String fileName = scanner.nextLine().trim();
if (fileName.equalsIgnoreCase("QUIT")) {
break;
}
try {
String text = convertMorseCodeToText(fileName, morseCodeMap);
System.out.println(text);
} catch (IOException e) {
System.out.println("File '" + fileName + "' is not found.");
}
}
scanner.close();
}
private static Map<String, String> readMorseCodeTable(String fileName) {
Map<String, String> morseCodeMap = new HashMap<>();
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split("\\s+");
if (parts.length == 2) {
morseCodeMap.put(parts[1], parts[0]);
}
}
} catch (IOException e) {
e.printStackTrace();
}
return morseCodeMap;
}
private static String convertMorseCodeToText(String fileName, Map<String, String> morseCodeMap) throws IOException {
StringBuilder result = new StringBuilder();
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
String[] morseWords = line.split("\\s{3,}");
for (String morseWord : morseWords) {
String[] morseLetters = morseWord.split("\\s+");
for (String morseLetter : morseLetters) {
if (morseCodeMap.containsKey(morseLetter)) {
result.append(morseCodeMap.get(morseLetter));
}
}
}
result.append("\n");
}
}
return result.toString().trim();
}
}
File data down below:
Morse.txt
0 -----
1 .----
2 ..---
3 ...--
4 ....-
5 .....
6 -....
7 --...
8 ---..
9 ----.
, --..--
. .-.-.-
? ..--..
A .-
B -...
C -.-.
D -..
E .
F ..-.
G --.
H ....
I ..
J .---
K -.-
L .-..
M --
N -.
O ---
P .--.
Q --.-
R .-.
S ...
T -
U ..-
V ...-
W .--
X -..-
Y -.--
Z --..
input1.txt
- .... . --- .-. .. --. .. -. .- .-.. --.- ..- . ... - .. --- -. --..--
-.-. .- -. -- .- -.-. .... .. -. . ... - .... .. -. -.- ..--..
.. -... . .-.. .. . ...- . - --- -... . - --- --- -- . .- -. .. -. --. .-.. . ... ... - --- -.. . ... . .-. ...- . -.. .. ... -.-. ..- ... ... .. --- -. .-.-.-
.- .-.. .- -. - ..- .-. .. -. --.
Test Case 1
input1.txtENTER
THEORIGINALQUESTION,\n
CANMACHINESTHINK?\n
IBELIEVETOBETOOMEANINGLESSTODESERVEDISCUSSION.\n
ALANTURING\n
Test Case 2
input2.txtENTER
File 'input2.txt' is not found.\n
Please re-enter the file name or type QUIT to exit:\n
quitENTER
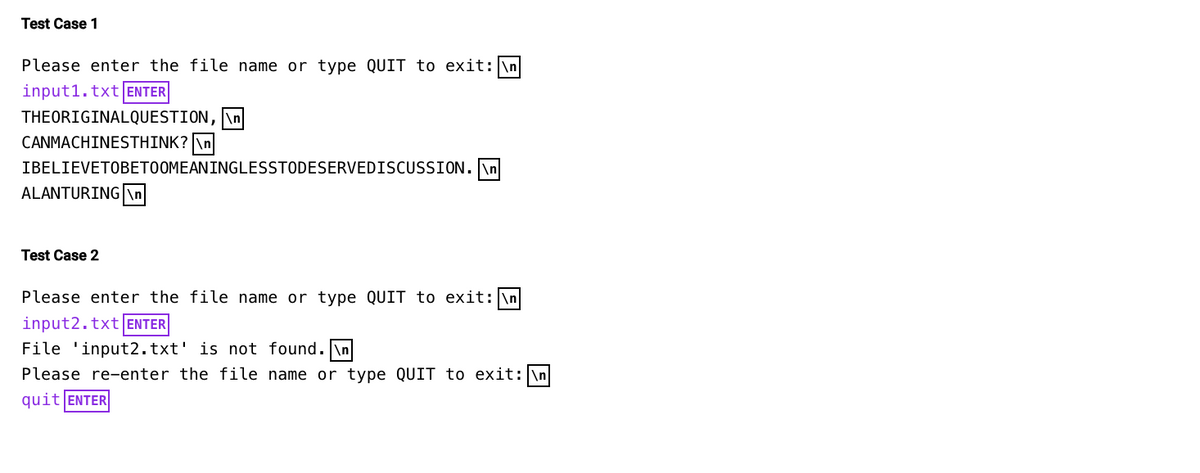
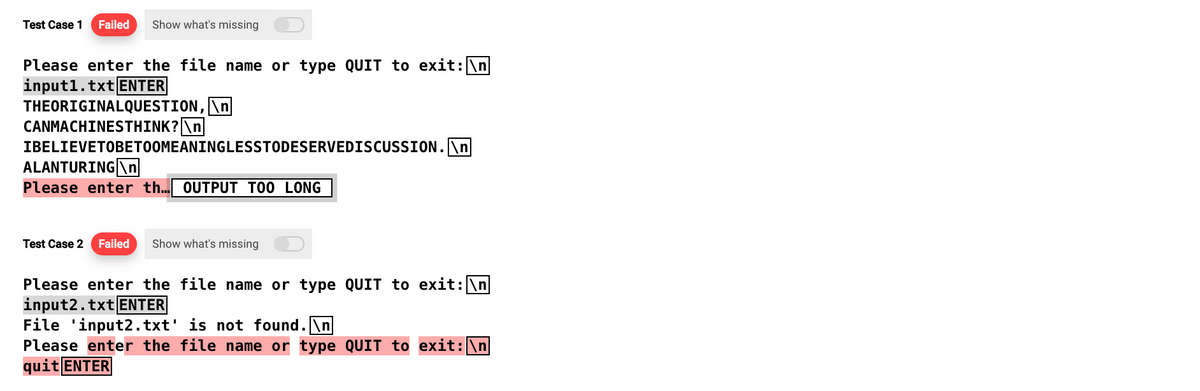

Step by step
Solved in 3 steps with 2 images

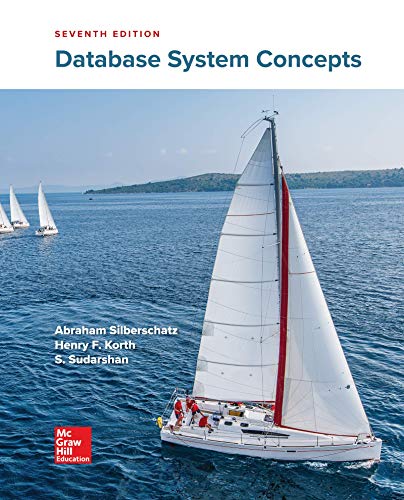
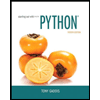
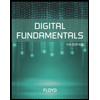
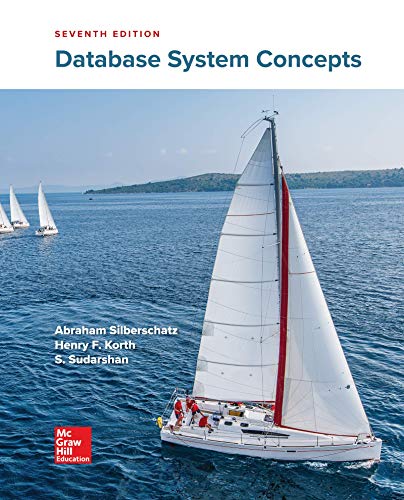
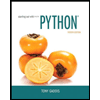
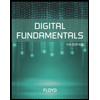
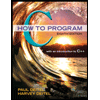
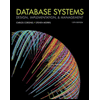
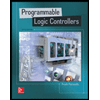