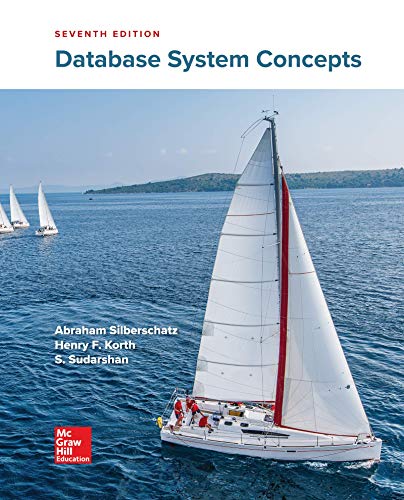
Please explain a bit more in the way of footnotes. From the given text it's not clear what are we reading about.\n and there needs to be nothing after that.
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class MorseCodeConverter {
public static void main(String[] args) {
Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt");
Scanner scanner = new Scanner(System.in);
System.out.print("Please enter the file name or type QUIT to exit:\n");
do{
String fileName = scanner.nextLine().trim();
if (fileName.equalsIgnoreCase("QUIT")) {
break;
}
try {
String text = convertMorseCodeToText(fileName, morseCodeMap);
System.out.println(text);
break;
} catch (IOException e) {
System.out.println("File '" + fileName + "' is not found.");
System.out.print("Please re-enter the file name or type QUIT to exit:\n");
}
}while(true);
scanner.close();
}
private static Map<String, String> readMorseCodeTable(String fileName) {
Map<String, String> morseCodeMap = new HashMap<>();
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split("\\s+");
if (parts.length == 2) {
morseCodeMap.put(parts[1], parts[0]);
}
}
} catch (IOException e) {
e.printStackTrace();
}
return morseCodeMap;
}
private static String convertMorseCodeToText(String fileName, Map<String, String> morseCodeMap) throws IOException {
StringBuilder result = new StringBuilder();
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
String[] morseWords = line.split("\\s{3,}");
for (String morseWord : morseWords) {
String[] morseLetters = morseWord.split("\\s+");
for (String morseLetter : morseLetters) {
if (morseCodeMap.containsKey(morseLetter)) {
result.append(morseCodeMap.get(morseLetter));
}
}
}
result.append("\n");
}
}
return result.toString().trim();
}
}
PleaseExplainABitMoreInTheWayOfFootnotes.FromTheGivenTextIt'sNotClearWhatAreWeReadingAbout.
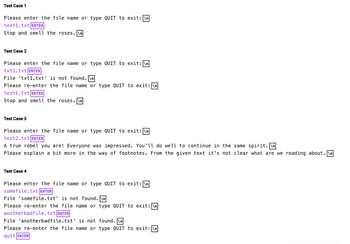

Step by stepSolved in 4 steps with 5 images

- *The following needs to be in python For this application, you will need to create a Python application that reads the sales for 12 months from a file and calculates the total yearly sales as well as the average monthly sales. In addition, this program should let the user edit the sales for any month. Jan 14317 Feb 3903 Mar 1073 Apr 3463 May 2429 Jun 4324 Jul 9762 Aug 15578 Sep 2437 Oct 6735 Nov 88 Dec 2497 Step 1 Define a display_title() function and have it use the print() to show the text: [FirstName] [LastName]'s Monthly Sales Replace the placeholders with your own first and last name add a space using the print() Define a display_menu() function and have it Use the print() function several times to show the following text with a space after: COMMAND MENUmonthly - View monthly salesyearly - View yearly summaryedit - Edit sales for a monthexit - Exit program Step 2 Define a main() function Call the display_title() function and the…arrow_forwardThe local t-shirt shop sells shirts that retail for $14.99. Quantity discounts are given as follow: Number of Shirts Discount < 10 0% 11-20 10% 21-50 15% 51 or more 20% Write a program that can run within a .cpp file that prompts the user for the number of shirts required and then computes the total price. Output the cost per shirt, discount percentage, and total cost. Make sure the program accepts only nonnegative input. You must use a constant to represent price of the shirt You must use the correct data types for your constants and variables You must validate your input. Do not allow negative number of shirts. This should display a warning You must use one or more if/else branches for your decisions You must also use a logical operator at least once to check the ranges. Your output should be formatted correctly Prices should have two decimal places Percents should have one decimal place Sample Run: How many shirts would you like?25 The cost per…arrow_forwardUsing a Counter-Controlled whileLoop Summary In this lab, you use a counter-controlled while loop in a Java program provided for you. When completed, the program should print the numbers 0 through 10, along with their values multiplied by 2 and by 10. The data file contains the necessary variable declarations and some output statements. Instructions Ensure the file named Multiply.java is open. Write a counter-controlled while loop that uses the loop control variable to take on the values 0 through 10. Remember to initialize the loop control variable before the program enters the loop. In the body of the loop, multiply the value of the loop control variable by 2 and by 10. Remember to change the value of the loop control variable in the body of the loop. Execute the program by clicking Run. Record the output of this program.arrow_forward
- -all-occurrences-of-a-substring-in-a-string/.arrow_forwardJava Program ASAP ************This program must work in hypergrade and pass all the test cases.********** Please give me a new program becausse when I upload the program to hypergrade it does not pass the test cases. It says 1 out of 4 passed. Thank you! For Test Case 1 first print out Please enter the file name or type QUIT to exit:\ then you type text1.txtENTER and it displays Stop And Smell The Roses./n there needs to be nothing after that. For test case 2 first print out Please enter the file name or type QUIT to exit: then you type txt1.txtENTER then it reads out File 'txt1.txt' is not found.\n Then it didplays Please re-enter the file name or type QUIT to exit:\n after the test file is not found. then you type in text1.txt and it displays stop and smell the roses.\n. For test case 3 first print out Please enter the file name or type QUIT to exit: then you type text2.txtENTER and it displays A true rebel you are! Everyone was impressed. You'll do well to continue in…arrow_forwardi would like some help in java using eclipsearrow_forward
- pythonarrow_forwardWhat does the following python code do? f = open("sample.txt", "w") Choose all that apply. Select 2 correct answer(s) Question 14 options: If the file called sample.txt exists, it writes at the bottom of the file writes "w" to the file called sample.txt closes a file called sample.txt opens a file called sample.txt for appending opens a file called sample.txt for reading reads the file called sample.txt starting from the top if the file sample.txt exists, deletes everything in it if the file sample.txt does not exist, it creates the file and opens it for writingarrow_forward The fields below repeat for each customer: o Customer name (String)o Customer ID (numeric integer) o Bill balance (numeric)o EmailAddress (String)o Tax liability (numeric or String) The customers served by the office supply store are of two types: tax-exempt or non-tax- exempt. For a tax-exempt customer, the tax liability field on the file is the reason for the tax exemptions: education, non-profit, government, other (String). For a non-tax exempt customer, the tax liability field is the percent of tax that the customer will pay (numeric) based on the state where the customer’s business resides. Program requirements: From the information provided, write a solution that includes the following: A suitable inheritance hierarchy which represents the customers serviced by the office supply company. It is up to you how to design the inheritance hierarchy. I suggest a Customer class and appropriate subclasses.. For all classes include the following: o Instance variables o…arrow_forward
- *From now and on yourLastName will be changed to your last name. *Your program should change White to your last name. *Your program should change McKINLEY WHITE to your name. *Change Mary Lane to the name of user who is using the Investment Application entered from the keyboard. *Write the file name as the first comment line at the top of the program. *After running your program, get the picture of the output window from your program with your name on to paste at the bottom of the pseudo-code to turn in *Step1: Read the requirement of each part; write the pseudo-code in a word document by listing the step by step what you suppose to do in main() and then save it with the name as Lab2_pseudoCode_yourLastName. *Step2: start editor (for example eClipse) create the project with the following project name: Part 1: SU2022_LAB2PART1_yourLastName -add a driver class (file with extension .java) that contain main()…arrow_forwardprofile-image Time remaining: 00 : 09 : 42 Computer Science C++. Need help writing a program that plays the game of Hangman. The program should pick a word (which is either coded directly into the program or read from a text file) and display the following: Guess the word: XXXXXX Each X represents a letter. The user tries to guess the letters in the word. The appropriate response yes or no should be displayed after each guess. After each incorrect guess, display the diagram with another body part filled. After seven incorrect guesses, the user should be hanged. The display should look as follows: O /|\ | / \ After each guess, display all user guesses. If the user guesses the word correctly, display: " Congratulations!!! You guessed my word. Play again? yes/no " Please help in making this code and if possible to include steps to learn what most of the lines and functions do in order to understand it better. Thank you.arrow_forward************This program must work in hypergrade and pass all the test cases.********** Remove the extra space \n from the program and dont take out quit from the program because it requires a space for it as shown in the screenshot. The text files are located in Hypergrade. For test case 1 first display Please enter a string to convert to Morse code:\n then you press Enter it should print out \n. Then for test case 2 it should display Please enter a string to convert to Morse code:\n then you type abc it should print out .- -... -.-. \n For test case 3 first display Please enter a string to convert to Morse code:\n then you type This is a sample string 1234.ENTER it should print put - .... .. ... .. ... .- ... .- -- .--. .-.. . ... - .-. .. -. --. .---- ..--- ...-- ....- .-.-.- \n. This program down below does not pass the test cases as shown in the screenshot I have provided the correct test case as a screenshot too. Please modify it or create a new program so it…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
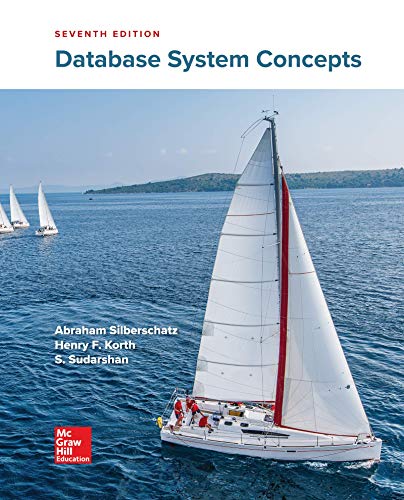
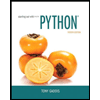
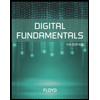
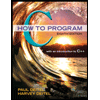
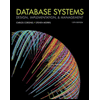
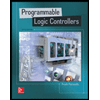