Please add comments to my program. Thank you in advance! :) package examples; import java.util.Scanner; public class ascendingOrder { public static void main(String[] args) { int count, temp; //User inputs the array size Scanner scan = new Scanner(System.in); System.out.print("\nEnter number of elements you want in the array: "); count = scan.nextInt(); int num[] = new int[count]; System.out.println("\nEnter array elements:"); for (int i = 0; i < count; i++) { num[i] = scan.nextInt(); } scan.close(); for (int i = 0; i < count; i++) { for (int j = i + 1; j < count; j++) { if (num[i] > num[j]) { temp = num[i]; num[i] = num[j]; num[j] = temp; } } } System.out.print("Array Elements in Ascending Order: "); for (int i = 0; i < count - 1; i++) { System.out.print(num[i] + ", "); } System.out.print(num[count - 1]); System.out.print("\n"); }
Please add comments to my
package examples;
import java.util.Scanner;
public class ascendingOrder
{
public static void main(String[] args)
{
int count, temp;
//User inputs the array size
Scanner scan = new Scanner(System.in);
System.out.print("\nEnter number of elements you want in the array: ");
count = scan.nextInt();
int num[] = new int[count];
System.out.println("\nEnter array elements:");
for (int i = 0; i < count; i++)
{
num[i] = scan.nextInt();
}
scan.close();
for (int i = 0; i < count; i++)
{
for (int j = i + 1; j < count; j++) {
if (num[i] > num[j])
{
temp = num[i];
num[i] = num[j];
num[j] = temp;
}
}
}
System.out.print("Array Elements in Ascending Order: ");
for (int i = 0; i < count - 1; i++)
{
System.out.print(num[i] + ", ");
}
System.out.print(num[count - 1]);
System.out.print("\n");
}
}

Step by step
Solved in 2 steps

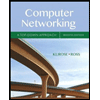
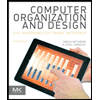
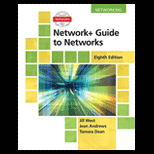
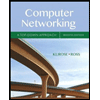
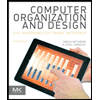
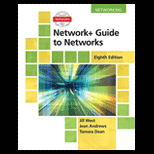
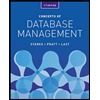
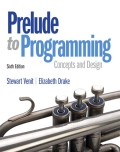
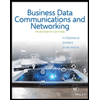