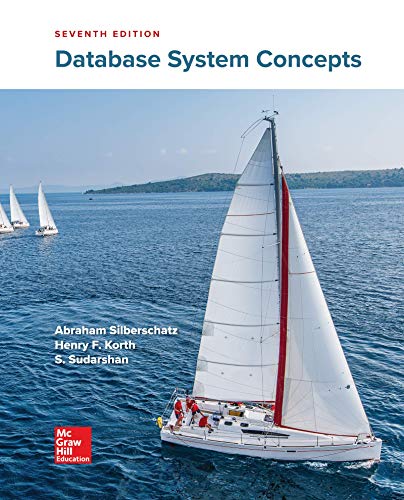
Write Java statements to add the integers 1001, 1100, and 1110 to the resulting ArrayList
import java.util.*;
public class test {
public static void main(String[] args)
{
//Set n =25
int n = 25;
//ArrayList of Integer type with size,n=25
ArrayList<Integer> list = new ArrayList<Integer>(n);
for(int i = 0;i<n;i++){
//Assign -1 to all the objects of the array list,list
list.add(-1);
}
//Add 1001 to the list
list.add(1001);
//Add 1100 to the list
list.add(1100);
//Add 1110 to the list
list.add(1110);
//Reset the number of elements in the list becomes 28
//Use size() method to get the size of the list.
n=list.size();
//for loop to print the list
for(int i = 0;i<n;i++){
System.out.print(list.get(i) + " ");
}
}
}
1. Write the Java statement to display the size of the resulting ArrayList from above.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- java Create a static method that: is called repeatAll returns ArrayList of Booleans takes in a single parameter - an ArrayList of Booleans This method should modify its ArrayList parameter by repeating its ArrayList values. For example, if the parameter is (true, false, false) The modified ArrayList should be (true, false, false, true, false, false) public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); ArrayList<Boolean> list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.nextBoolean()); } System.out.println(repeatAll(list)); } }arrow_forwardjava Create a method that: is called timesTwo returns an ArrayList of Integers takes in a single parameter - an ArrayList of Integers called nums This method should take the ArrayList parameter and multiply every value by two. public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); ArrayList<Integer> list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.nextInt()); } System.out.println(timesTwo(list)); } }arrow_forwardFor JAVA.arrow_forward
- Please use the template provided below and make sure the output matches exactly. import java.util.Scanner;import java.util.ArrayList; public class PhotoLineups { // TODO: Write method to create and output all permutations of the list of names. public static void printAllPermutations(ArrayList<String> permList, ArrayList<String> nameList) { } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); ArrayList<String> nameList = new ArrayList<String>(); ArrayList<String> permList = new ArrayList<String>(); String name; // TODO: Read in a list of names; stop when -1 is read. Then call recursive method. }}arrow_forwardCorrect my codes in java // Arraysimport java.util.Scanner;public class Assignment1 {public static void main (String args[]){Scanner sc=new Scanner (System.in);System.out.println("Enter mark of student");int n=sc.nextInt();int a[]=new int [n];int i;for(i=0;i<n;i++){System.out.println("Total marks of student in smster");a[i]=sc.nextInt();}int sum=0;for(i=0;i<n;i++){sum=sum+a[i];}System.out.println("Total marks is :");for (i=0;i<n;i++);{System.out.println(a[i]);}System.out.println();}}arrow_forwardpublic class arrayOutput ( public static void main (String [] args) { final int NUM ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; } Type the program's output userVals [0] = 2; userVals [1] = 6; userVals [2] = 8; for (i = userVals.length - 1; i >= 0; −−1) { System.out.println(userVals [1]); } C.C. ? ? ??arrow_forward
- Need help with menu loop pleasearrow_forwardWrite in Java What is this program's exact outputarrow_forwardstarter code import java.util.ArrayList; public class SortsTracing { //Note: Style is not required for this file //for reference public ArrayList<int[]> SelectionSortExampleList() { ArrayList<int[]> answer = new ArrayList<int[]>(); answer.add(new int[]{-1, 20, 18, 17, 9, 4, 2, 0, 40}); answer.add(new int[]{-1, 0, 18, 17, 9, 4, 2, 20, 40}); answer.add(new int[]{-1, 0, 2, 17, 9, 4, 18, 20, 40}); answer.add(new int[]{-1, 0, 2, 4, 9, 17, 18, 20, 40}); answer.add(new int[]{-1, 0, 2, 4, 9, 17, 18, 20, 40}); answer.add(new int[]{-1, 0, 2, 4, 9, 17, 18, 20, 40}); // etc... (the rest of the iterations) return answer; } public ArrayList<int[]> InsertionSortRandomList() { ArrayList<int[]> answer = new ArrayList<int[]>(); answer.add(new int[]{30, 62, 5, 109, 66, 17, 51, 18}); // TODO return answer; } public ArrayList<int[]>…arrow_forward
- Java language Use arrays in creating your class. Stack Interface (LIFO) void push(Object) Object pop() String toString() boolean isEmpty() boolean equals(Object) getIndexOf get remove private static void arrayListTests() { System.out.println("ArrayList Tests"); // todo: make more tests here ArrayList a = new ArrayList(); System.out.println("Check empty array isEmpty:" + a.isEmpty()); a.insert('B', 0); a.insert('a', 0); a.insert('t', 1); System.out.println("Check non-empty array isEmpty:" + a.isEmpty()); System.out.println(a.toString()); while (a.isEmpty() == false) { System.out.println(a.remove(0)); } // Fill over initial capacity and check that it grows for (int i = 0; i < 110; i++) { a.append(new Integer(i)); } System.out.println("Size of array after 110 adds: "+ a.size()); System.out.println("Value of last element: "+ a.get(a.size()-1)); System.out.println("Insert past end of list"); a.insert('z', 200); System.out.println("Insert negative index"); a.insert('z', -3);…arrow_forwardJavaTimer.java: import java.util.Arrays;import java.util.Random; public class JavaTimer { // Please expand method main() to meet the requirements.// You have the following sorting methods available:// insertionSort(int[] a);// selectionSort(int[] a);// mergeSort(int[] a);// quickSort(int[] a);// The array will be in sorted order after the routines are called!// Be sure to re-randomize the array after each sort.public static void main(String[] args) {// Create and initialize arraysint[] a = {1, 3, 5}, b, c, d;// Check the time to sort array along startTime = System.nanoTime();quickSort(a);long endTime = System.nanoTime();long duration = (endTime - startTime) / 1000l;// Output resultsSystem.out.println("Working on an array of length " + a.length + ".");System.out.println("Quick sort: " + duration + "us.");}// Thanks to https://www.javatpoint.com/insertion-sort-in-javapublic static void insertionSort(int array[]) {int n = array.length;for (int j = 1; j < n; j++) {int key = array[j];int…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
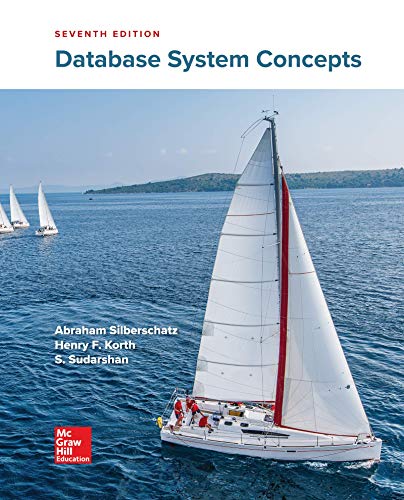
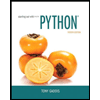
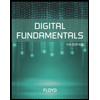
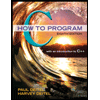
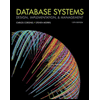
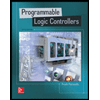