this is the template to be user. Add the code here according to the question in image. in java language. public class MergeSort { ArrayList getInput(ArrayList al) { // } void getOutput(ArrayList al) { // } void merge(ArrayList al, int beg, int mid, int end) { // } void sort(ArrayList al, int beg, int end) { // } public static void main(String[] args) { // TODO Auto-generated method stub } }
this is the template to be user. Add the code here according to the question in image. in java language. public class MergeSort { ArrayList getInput(ArrayList al) { // } void getOutput(ArrayList al) { // } void merge(ArrayList al, int beg, int mid, int end) { // } void sort(ArrayList al, int beg, int end) { // } public static void main(String[] args) { // TODO Auto-generated method stub } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
this is the template to be user. Add the code here according to the question in image. in java language.
public class MergeSort {
ArrayList getInput(ArrayList<Integer> al) { // }
void getOutput(ArrayList<Integer> al) { // }
void merge(ArrayList<Integer> al, int beg, int mid, int end) { // }
void sort(ArrayList<Integer> al, int beg, int end) { // }
public static void main(String[] args) { // TODO Auto-generated method stub }
}
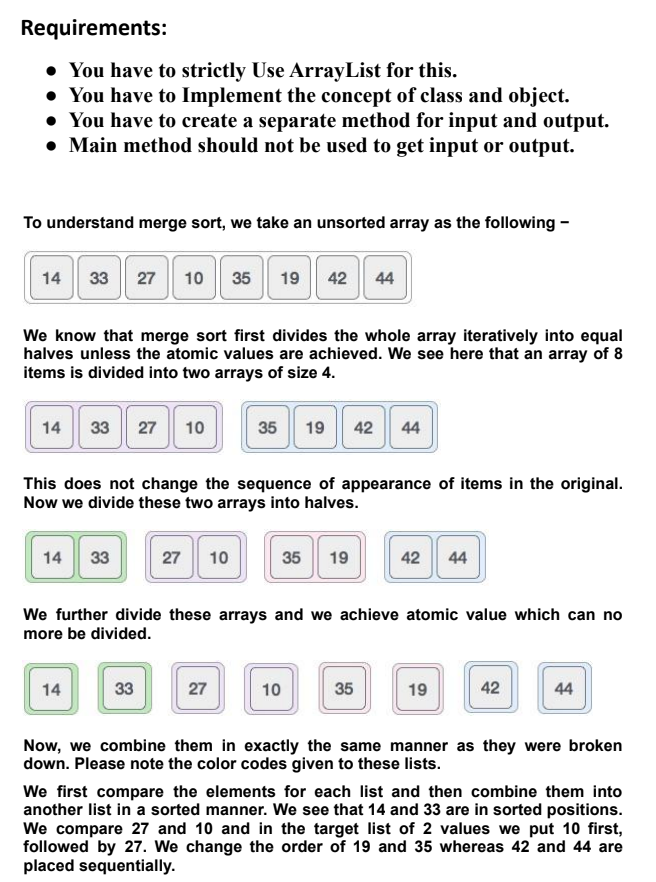
Transcribed Image Text:Requirements:
• You have to strictly Use ArrayList for this.
• You have to Implement the concept of class and object.
• You have to create a separate method for input and output.
• Main method should not be used to get input or output.
To understand merge sort, we take an unsorted array as the following -
14
33 27
10 35 19 | 42 44
We know that merge sort first divides the whole array iteratively into equal
halves unless the atomic values are achieved. We see here that an array of 8
items is divided into two arrays of size 4.
14
33 27 10
35
19 42 44
This does not change the sequence of appearance of items in the original.
Now we divide these two arrays into halves.
14
33
27
10
35
19
42 44
We further divide these arrays and we achieve atomic value which can no
more be divided.
14
33
27
10
35
19
42
44
Now, we combine them in exactly the same manner as they were broken
down. Please note the color codes given to these lists.
We first compare the elements for each list and then combine them into
another list in a sorted manner. We see that 14 and 33 are in sorted positions.
We compare 27 and 10 and in the target list of 2 values we put 10 first,
followed by 27. We change the order of 19 and 35 whereas 42 and 44 are
placed sequentially.
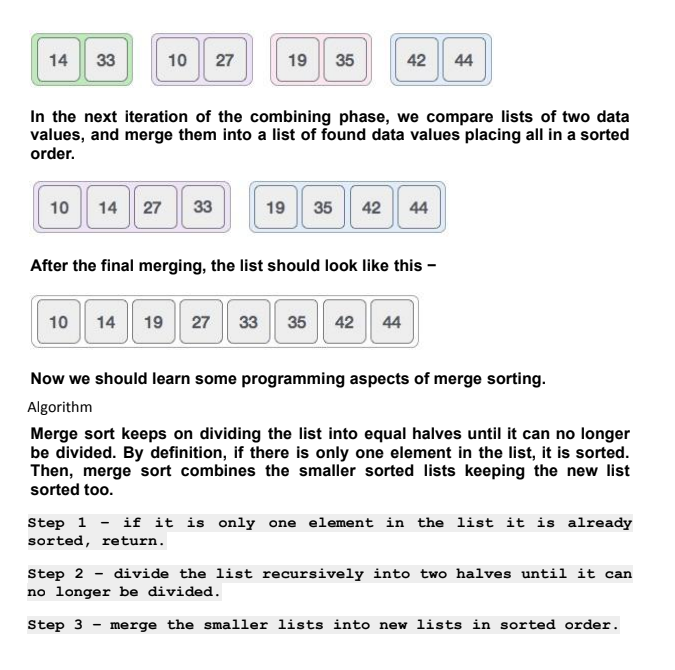
Transcribed Image Text:14
33
10
27
19
35
42
44
In the next iteration of the combining phase, we compare lists of two data
values, and merge them into a list of found data values placing all in a sorted
order.
14 27 33
42 44
10
19
35
After the final merging, the list should look like this -
10
14
19 27
33
35
42
44
Now we should learn some programming aspects of merge sorting.
Algorithm
Merge sort keeps on dividing the list into equal halves until it can no longer
be divided. By definition, if there is only one element in the list, it is sorted.
Then, merge sort combines the smaller sorted lists keeping the new list
sorted too.
Step 1 - if it is only one element in the list it is already
sorted, return.
Step 2 - divide the list recursively into two halves until it can
no longer be divided.
Step 3 - merge the smaller lists into new lists in sorted order.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
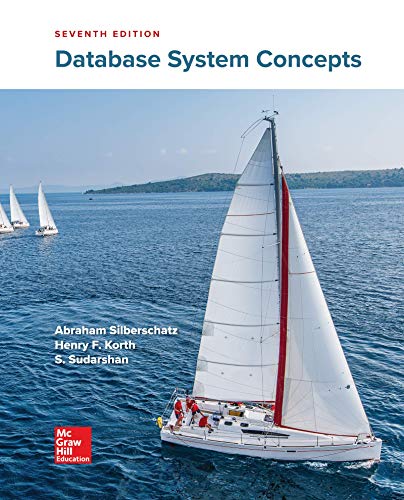
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
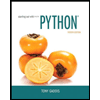
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
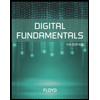
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
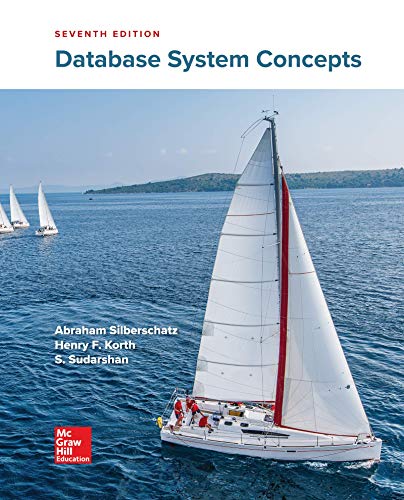
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
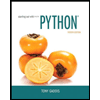
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
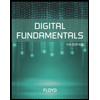
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
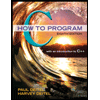
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
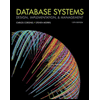
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
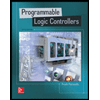
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education