Kindly help check for correction in the attached. Benchmarking Sorting Algorithms The same task can take vastly different amounts of time, depending on the algorithm that is used to perform the task. You are familiar with simple sorting algorithms such as insertion sort and selection sort. While these methods work fine for small arrays, for larger arrays they can take an unreasonable amount of time. The question is whether we can do any better. Java has some built-in sorting methods. They can be found in the class named Arrays in the package java.util. The one that you will use in this lab is Arrays.sort(A), which sorts the entire array A into increasing order. (Actually, there are different methods for different array base types, but all the methods have the same name and are used in the same way. You will be using an array of ints in this lab.) You should write a program that does the following: Create two arrays of type int[]. Both arrays should be the same size, and the size should be given by a constant in the program so that you can change it easily. Fill the arrays with random integers. The arrays should have identical contents, with the same random numbers in both arrays. To generate random integers with a wide range of sizes, you could use (int)(Integer.MAX_VALUE * Math.random()). Sort the first array using either Selection Sort or Insertion Sort. You should add the sorting method to your program; you can copy it from (http://math.hws.edu/javanotes/ ; Section 7.4) if you want. (It is a good idea to check that you got the sorting method correct by using it to sort a short array and printing out the result.) Time how long it takes to sort the array and print out the time. Now, sort the second (identical) array using Arrays.sort(). Again, time how long it takes, and print out the time. You should run your program using array sizes of 1,000, 10,000, and 100,000. Record the sort times. Add a comment to the top of the program that reports the times. (You might be interested in applying Arrays.sort() to a million-element array, but don't try that with Selection Sort or Insertion Sort!) Note: The general method for getting the run time of a code segment is: long startTime = System.currentTimeMillis(); doSomething(); long runTime = System.currentTimeMillis() - startTime; This gives the run time in milliseconds. If you want the time in seconds, you can use runTime/1000.0.
Kindly help check for correction in the attached.
Benchmarking Sorting Algorithms
The same task can take vastly different amounts of time, depending on the
Java has some built-in sorting methods. They can be found in the class named Arrays in the package java.util. The one that you will use in this lab is Arrays.sort(A), which sorts the entire array A into increasing order. (Actually, there are different methods for different array base types, but all the methods have the same name and are used in the same way. You will be using an array of ints in this lab.)
You should write a
- Create two arrays of type int[]. Both arrays should be the same size, and the size should be given by a constant in the program so that you can change it easily.
- Fill the arrays with random integers. The arrays should have identical contents, with the same random numbers in both arrays. To generate random integers with a wide range of sizes, you could use (int)(Integer.MAX_VALUE * Math.random()).
- Sort the first array using either Selection Sort or Insertion Sort. You should add the sorting method to your program; you can copy it from (http://math.hws.edu/javanotes/ ; Section 7.4) if you want. (It is a good idea to check that you got the sorting method correct by using it to sort a short array and printing out the result.)
- Time how long it takes to sort the array and print out the time.
- Now, sort the second (identical) array using Arrays.sort(). Again, time how long it takes, and print out the time.
You should run your program using array sizes of 1,000, 10,000, and 100,000. Record the sort times. Add a comment to the top of the program that reports the times. (You might be interested in applying Arrays.sort() to a million-element array, but don't try that with Selection Sort or Insertion Sort!)
Note: The general method for getting the run time of a code segment is:
long startTime = System.currentTimeMillis();
doSomething();
long runTime = System.currentTimeMillis() - startTime;
This gives the run time in milliseconds. If you want the time in seconds, you can use runTime/1000.0.
![E eclipse-workspace - Lab1/src/BenchmarkingSortingAlgorithms.java - Eclipse IDE
File Edit Source Refactor Navigate Search Project Run Window Help
台▼圖
E Package Explorer 3 E Project Explorer
D MathQuizjava
D TextlO.java
D AdditionProblem.java
A BenchmarkingSortingAlgorithms.java X
E Outline X
vS Lab1
EA JRE System Library [JavaSE-15]
System.out.println("Arrays Sort time(sec):"+runTimeArray2/1000.0); // Print result
}
27
9 BenchmarkingSortingAlgorithms
A maxArraySize : int
sortingArray1: int[)
A sortingArray2 : int[]
° BenchmarkingSortingAlgorithms
AS selectionSort(int[]) : void
28
vA src
29
30
v E (default package)
> D AdditionProblem.java
> D BenchmarkingSortingAlgorithms.java
> D MathQuiz.java
> D Textl0.java
A
310
static void selectionSort(int[] A) {
// Sort A in increasing order, using selection sort
for (int lastPlace = A.lengthfi-1; lastPlace > 0; lastPlace fifi)) k // Find t
// A[lastPlace], and move it into position lastPlace
// by swapping it with the number that is currently
// in position lastPlace.
int maxLoc = 0; // Location of largest item seen so far.
for (int j = 1; j <= lastPlace; j++) {
if (A[j] > A[maxLoc]) {
// Since A[il is bigger than the maximum we have seen
// so far, i is the new location of the maximum value
// we have seen so far.
maxLoc = j;
}
}
int temp = A[maxLoc]; // Swap largest item with A[lastPlace].
A[maxLoc] = A[1lastPlace];
A[lastPlace] = temp;
E // end of for loop
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
%3D
}
51
52
}
53
54
A Problems @ Javadoc e Declaration e Console 3
關
AdditionProblem [Java Application] C:\Users\user\.p2\pool\plugins\org.eclipse.justj.openjdk.hotspot.jre.full.win32.x86_64_15.0.2.v20210201-0955\jre\bin\javaw.exe (Jun 23, 2021, 9:37:46
The sum of : 34 + 13
Please enter your answer
Writable
Smart Insert
33: 84: 1729](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5dd53dd1-02d0-442f-939d-39f076a6afbe%2F2a006fca-626e-44c3-a961-9dfc41a9d6d5%2Fyvcemed_processed.png&w=3840&q=75)
![E eclipse-workspace - Lab1/src/BenchmarkingSortingAlgorithms.java - Eclipse IDE
File Edit Source Refactor Navigate Search Project Run Window Help
- O - Q ▼
国 T , ▼や
E Package Explorer X E Project Explorer
D MathQuizjava
D TextlO.java
D AdditionProblem.java
D BenchmarkingSortingAlgorithms.java 8
E Outline 8
v Lab1
> A JRE System Library [JavaSE-15]
v 9 BenchmarkingSortingAlgorithms
v src
v E (default package)
> D AdditionProblem.java
> D BenchmarkingSortingAlgorithms.java
> D MathQuiz.java
> D Textl0.java
import java.util.*;
public class BenchmarkingSortingAlgorithms {
// Compute benchmarks of two different sorting techniques
int maxArraysize=10000; // Array Size
int[] sortingArray1 = new int[maxArraySize]; // First Array
int[] sortingArray2 = new int[maxArraysize]; // Second Array
maxArraySize : int
sortingArray1: int[]
A sortingArray2 : int[]
C BenchmarkingSortingAlgorithms
selectionSort(int[]) : void
6.
8
9.
public BenchmarkingSortingAlgorithms (){// Class Constructor
for (int i = 0; i < sortingArray1.length; i++) {
// Filling two arrays with the same random numbers.
sortingArray1[i]=(int) (Integer.MAX_VALUE * Math.random());
sortingArray2[i]=sortingArray1[i];
100
11
12
13
14
15
16
long startTimeArray1 = System.currentTimeMillis();
// Start computing time for SelectionSort
selectionSort(sortingArray1); // Sorting Array1 with SelectionSort
long runTimeArray1 = System.currentTimeMillis() - startTimeArray1; //Time to run t
17
18
19
20
long startTimeArrav2 = System.currentTimeMillis();// Start computing time for Arra
Arrays.sort(sortingArray2); // Sorting Array2 with Arrays.sort
long runTimeArray2 = System.currentTimeMillis(); // Time to run the Arrays.sort
System.out.println("SelectionSort time(sec):"+runTimeArray1/1000.0); // Print resu
System.out.println("Arrays Sort time(sec):"+runTimeArray2/1000.0); // Print result
A Problems @ Javadoc e Declaration e Console 3
AdditionProblem [Java Application] C:\Users\user\.p2\pool\plugins\org.eclipse.justj.openjdk.hotspot.jre.full.win32.x86_64_15.0.2.v20210201-0955\jre\bin\javaw.exe (Jun 23, 2021, 9:37:46
The sum of : 34 + 13
Please enter your answer
Writable
Smart Insert
33: 84: 1729](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5dd53dd1-02d0-442f-939d-39f076a6afbe%2F2a006fca-626e-44c3-a961-9dfc41a9d6d5%2Fuqqmtju_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

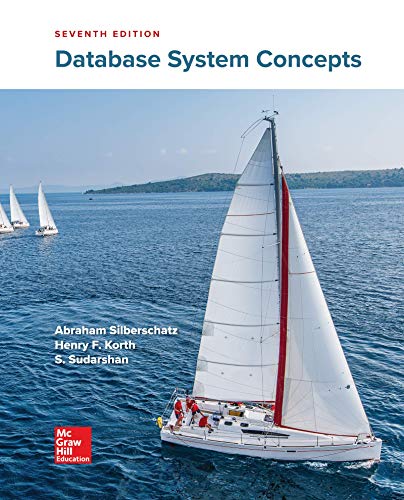
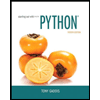
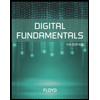
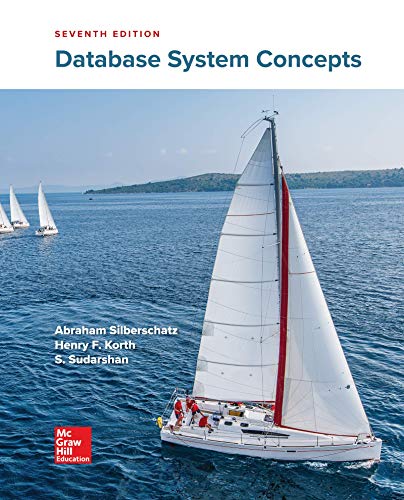
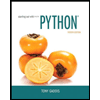
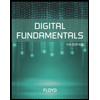
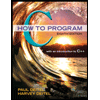
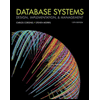
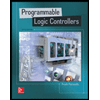