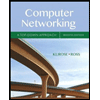
The language I am using is Java
The source code for Shortest Job First
Please help me to create a GUI for my code for CPU Scheduling: Shortest Job First. I have created the design for the GUI using windows builder in Java Eclipse.
I can't fully upload the code for my GUI because of the word limit in Bartleby. Therefore, you can do your own design of the GUI with the code in it. I need a functional and working code for the GUI where I can input the desired number I want for the burst time and arrival time. I hope you can help me with this. Thank you so much.
package test;
import java.util.*;
import javax.swing.JOptionPane;
public class SJF {
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
int x= Integer.parseInt(JOptionPane.showInputDialog("Enter number of process"));
int processID[] = new int[x]; //To display the process ID
int arTime[] = new int[x]; // arTime means arrival time
int burstTime[] = new int[x]; // burstTime means burst time
int complTime[] = new int[x]; // complTime means complete time
int TAT[] = new int[x]; // TAT means turn around time
int waitingTime[] = new int[x]; //waitingTime means waiting time
int process[] = new int[x]; // Checks if the process is completed
int ganttChart[] = new int[x]; //To display the Gantt Chart
int startTime=0, totalProcess=0; //startTime = start time and total process time to check if the process if finished to break from the while loop
float avrgWT=0, avrgTAT=0;
for(int i=0;i<x;i++) //for loop to get the arrival time and burst time
{
arTime[i] = Integer.parseInt(JOptionPane.showInputDialog("Input Arrival Time " + (i+1)));
burstTime[i] = Integer.parseInt(JOptionPane.showInputDialog("Input Burst Time " + (i+1)));
processID[i] = i+1;
process[i] = 0;
}
while(true)
{
int c=x, min=1000; //Looking for the smallest burst time
if (totalProcess == x) // total no of process = completed process loop will be terminated
break;
for (int i=0; i<x; i++)
{
if ((arTime[i] <= startTime) && (process[i] == 0) && (burstTime[i]<min))
{
min=burstTime[i];
c=i;
}
}
if (c==x)
startTime++;
else
{
complTime[c]=startTime+burstTime[c];
startTime+=burstTime[c];
TAT[c]=complTime[c]-arTime[c];
waitingTime[c]=TAT[c]-burstTime[c];
process[c]=1;
ganttChart[totalProcess] = c + 1;
totalProcess++;
}
}
for(int i=0;i<x;i++)
{
avrgWT+= waitingTime[i];
avrgTAT+= TAT[i];
System.out.println("Process ID: "+processID[i]+"\t"+"Arrival Time: "+arTime[i]+"\t"+" Burst Time: "+burstTime[i]+"\t"+"Completion Time: "+complTime[i]+"\t"+"Turn Around Time: "+TAT[i]+"\t"+"Waiting Time: "+waitingTime[i]);
}
System.out.println ("\nAverage TAT is "+ (float)(avrgTAT/x));
System.out.println ("Average Waiting Time is "+ (float)(avrgWT/x));
sc.close();
System.out.print("The Gantt Chart is ");
for(int i=0; i<x; i++)
{
System.out.print("P"+ganttChart[i]+ " ");
}
}
}
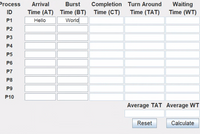

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Use the Eclipse IDE to write java code for Functionality for the “Doctor” interface: The system should allow a doctor to assign a new doctor to a patient using the list of all doctors. The system should then send confirmation messages to the patient and both doctors The appearance of the interface is not important as long as the required functionality is provided. That is, you are not expected to implement a fancy looking interface. just ensure it has a home button and a log out buttonarrow_forwardScenario: You have been tasked with building a URL file validator for a web crawler. A web crawler is an application that fetches a web page, extracts the URLs present in that page, and then recursively fetches new pages using the extracted URLs. The end goal of a web crawler is to collect text data, images, or other resources present in order to validate resource URLs or hyperlinks on a page. URL validators can be useful to validate if the extracted URL is a valid resource to fetch. In this scenario, you will build a URL validator that checks for supported protocols and file types.arrow_forwardAssume the base package for an Eclipse project is edu.westga.cs1301.drinks and that you are writing tests for the dispense method of a DrinkMachine class. Use this information to answer the following questionWhat should you name your test class?arrow_forward
- final big report project for java. 1.Problem Description Student information management system is used to input, display student information records. The GUI interface for inputing ia designed as follows : The top part are the student imformation used to input student information,and the bottom part are four buttonns, each button meaning : (2) Total : add java score and C++ score ,and display the result; (3) Save: save student record into file named student.dat; (4) Clear : set all fields on GUI to empty string; (5) Close : close the winarrow_forwardcan you help me create a simple java GUI about bus ticketing system. Please refer to the instruction below. Thank you!arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
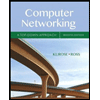
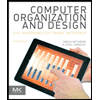
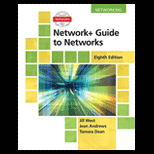
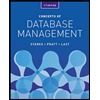
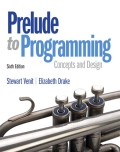
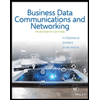