wroting a Java program with a single-dimension array that holds 10 integer numbers and identify the maximum value of the 10 numbers. Next sort the array using a bubble sort and display the array before and after sorting.
wroting a Java program with a single-dimension array that holds 10 integer numbers and identify the maximum value of the 10 numbers. Next sort the array using a bubble sort and display the array before and after sorting.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter12: Points, Classes, Virtual Functions And Abstract Classes
Section: Chapter Questions
Problem 29SA
Related questions
Question
I need help with wroting a Java
10 integer numbers and identify the maximum value of the 10
numbers. Next sort the array using a bubble sort and display the
array before and after sorting.
![11:15
LTE O
Chapter 7 Project - new Summer 19 3...
Chapter 7 Project
Project Name: Chpt7_Project
Class Name: Chpt7_Project
Write a Java program with a single-dimension array that holds
10 integer numbers and identify the maximum value of the 10
numbers. Next sort the array using a bubble sort and display the
array before and after sorting.
Step 1. Create an algorithm (either flowchart or pseudocode)
will use to write the program. Place the algorithm in a
that
you
Word document.
Step 2. Code the program in Eclipse and ensure the following
steps are accomplished.
1. Generate 10 random integer numbers between 1 and 100, and
place each random number in a different element of a single-
dimension array starting with the first number generated.
2. Locate the largest of the 10 numbers and display its value.
3. Display the array's contents in the order the numbers are
initially inserted. This is called an unsorted list.
4. Using the bubble sort, now sort the array from smallest
integer to the largest. The bubble sort must be in its own
method; it cannot be in the main method. Here is the code for
the bubble sort method. Use this code, and not some code you
find online.
Bubble Sort Code:
public static void bubbleSort(int[] list)
{
int temp;
for (int i = list.length - 1; i> 0; i--)
{
for (int j= 0; j<i; j++)
{
if (list[j] > list[j+ 1])
{
temp = list[j];
list[j] = list[j + 1];
list[j + 1] = temp;
}
}
}
(7
94
Dashboard
Calendar
Notifications
Inbox](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F42ffe971-9975-4e79-958e-ae533ea5e503%2Fe938fa17-f9e6-4c06-b374-09dc5de224f0%2Fk4hnmxw_processed.png&w=3840&q=75)
Transcribed Image Text:11:15
LTE O
Chapter 7 Project - new Summer 19 3...
Chapter 7 Project
Project Name: Chpt7_Project
Class Name: Chpt7_Project
Write a Java program with a single-dimension array that holds
10 integer numbers and identify the maximum value of the 10
numbers. Next sort the array using a bubble sort and display the
array before and after sorting.
Step 1. Create an algorithm (either flowchart or pseudocode)
will use to write the program. Place the algorithm in a
that
you
Word document.
Step 2. Code the program in Eclipse and ensure the following
steps are accomplished.
1. Generate 10 random integer numbers between 1 and 100, and
place each random number in a different element of a single-
dimension array starting with the first number generated.
2. Locate the largest of the 10 numbers and display its value.
3. Display the array's contents in the order the numbers are
initially inserted. This is called an unsorted list.
4. Using the bubble sort, now sort the array from smallest
integer to the largest. The bubble sort must be in its own
method; it cannot be in the main method. Here is the code for
the bubble sort method. Use this code, and not some code you
find online.
Bubble Sort Code:
public static void bubbleSort(int[] list)
{
int temp;
for (int i = list.length - 1; i> 0; i--)
{
for (int j= 0; j<i; j++)
{
if (list[j] > list[j+ 1])
{
temp = list[j];
list[j] = list[j + 1];
list[j + 1] = temp;
}
}
}
(7
94
Dashboard
Calendar
Notifications
Inbox
![11:15
LTE O
Chapter 7 Project - new Summer 19 3...
Z. LOCat tHC largest Of tlNe TV IIUIIIUCIS alng dispray its valut.
3. Display the array's contents in the order the numbers are
initially inserted. This is called an unsorted list.
4. Using the bubble sort, now sort the array from smallest
integer to the largest. The bubble sort must be in its own
method; it cannot be in the main method. Here is the code for
the bubble sort method. Use this code, and not some code you
find online.
Bubble Sort Code:
public static void bubbleSort(int[] list)
{
int temp;
for (int i = list.length - 1; i> 0; i--)
{
for (int j = 0; j <i; j++)
{
if (list[j] > list[j + 1])
{
temp = list[j];
list[j] = list[j + 1];
list[j + 1] = temp;
}
}
}
}
||
5. Display the array's contents after the bubble sort is completed.
6. Add comments at the top of the class with your First and Last
name, and comment the code to explain the various steps.
Step 3. Test your program. Below is an example of what the
program output should look like. Your output will be different
because the 10 random numbers will not be the same as the
example.
Use the Snip It tool in Windows or a similar tool on the Mac to
cut and paste the Eclipse Console output window into the same
Word document as the algorithm in Step 1.
<terminated> C7_Project (2) [Java Application] C:\Program Files\Java\jre1.8.0_131\bin\javaw.exe (Mar 26, 2019, 11:24:20 AM)
The largest value is 100
The unsorted list is: 64 24 25
48 71 1ee
81
3
The sorted list is: 3 5 24
47 48
64 71 81
100
SAMBLE
(7
94
Dashboard
Calendar
Notifications
Inbox](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F42ffe971-9975-4e79-958e-ae533ea5e503%2Fe938fa17-f9e6-4c06-b374-09dc5de224f0%2Fnwjhg5_processed.png&w=3840&q=75)
Transcribed Image Text:11:15
LTE O
Chapter 7 Project - new Summer 19 3...
Z. LOCat tHC largest Of tlNe TV IIUIIIUCIS alng dispray its valut.
3. Display the array's contents in the order the numbers are
initially inserted. This is called an unsorted list.
4. Using the bubble sort, now sort the array from smallest
integer to the largest. The bubble sort must be in its own
method; it cannot be in the main method. Here is the code for
the bubble sort method. Use this code, and not some code you
find online.
Bubble Sort Code:
public static void bubbleSort(int[] list)
{
int temp;
for (int i = list.length - 1; i> 0; i--)
{
for (int j = 0; j <i; j++)
{
if (list[j] > list[j + 1])
{
temp = list[j];
list[j] = list[j + 1];
list[j + 1] = temp;
}
}
}
}
||
5. Display the array's contents after the bubble sort is completed.
6. Add comments at the top of the class with your First and Last
name, and comment the code to explain the various steps.
Step 3. Test your program. Below is an example of what the
program output should look like. Your output will be different
because the 10 random numbers will not be the same as the
example.
Use the Snip It tool in Windows or a similar tool on the Mac to
cut and paste the Eclipse Console output window into the same
Word document as the algorithm in Step 1.
<terminated> C7_Project (2) [Java Application] C:\Program Files\Java\jre1.8.0_131\bin\javaw.exe (Mar 26, 2019, 11:24:20 AM)
The largest value is 100
The unsorted list is: 64 24 25
48 71 1ee
81
3
The sorted list is: 3 5 24
47 48
64 71 81
100
SAMBLE
(7
94
Dashboard
Calendar
Notifications
Inbox
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
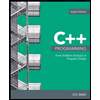
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
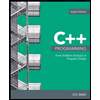
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning