Given the IntNode class, define the getCount() method in the CustomLinkedList class that returns the number of items in the list not including the head node. Ex: If the list contains: head -> 14 -> 19 -> 4 getCount(headObj) returns 3. Ex: If the list contains: head -> getCount(headObj) returns 0. public class IntNode { private int dataVal; // Node data private IntNode nextNodePtr; // Reference to the next node // Default constructor public IntNode() { dataVal = 0; nextNodePtr = null; } // Constructor public IntNode(int dataInit) { this.dataVal = dataInit; this.nextNodePtr = null; } // Constructor public IntNode(int dataInit, IntNode nextLoc) { this.dataVal = dataInit; this.nextNodePtr = nextLoc; } /* Insert node after this node. Before: this -- next After: this -- node -- next */ public void insertAfter(IntNode nodeLoc) { IntNode tmpNext; tmpNext = this.nextNodePtr; this.nextNodePtr = nodeLoc; nodeLoc.nextNodePtr = tmpNext; } // Get location pointed by nextNodePtr public IntNode getNext() { return this.nextNodePtr; } // Get node value public int getNodeData() { return this.dataVal; } // Print node value public void printNodeData() { System.out.println(this.dataVal); } } -------------------------------------------------------------------------------------------------------------------------------------------------------- public class CustomLinkedList { // TODO: Return number of nodes in list public static int getCount(IntNode headObj) { /* insert your code here */ return } public static void main(String[] args) { IntNode headObj; IntNode currObj; IntNode lastObj; int i; int count; // Create head node headObj = new IntNode(-1); lastObj = headObj; // Add nodes to the list for (i = 0; i < 20; ++i) { currObj = new IntNode(i); lastObj.insertAfter(currObj); lastObj = currObj; } count = getCount(headObj); System.out.println(count); } }
Given the IntNode class, define the getCount() method in the CustomLinkedList class that returns the number of items in the list not including the head node.
Ex: If the list contains:
head -> 14 -> 19 -> 4
getCount(headObj) returns 3.
Ex: If the list contains:
head ->
getCount(headObj) returns 0.
public class IntNode {
private int dataVal; // Node data
private IntNode nextNodePtr; // Reference to the next node
// Default constructor
public IntNode() {
dataVal = 0;
nextNodePtr = null;
}
// Constructor
public IntNode(int dataInit) {
this.dataVal = dataInit;
this.nextNodePtr = null;
}
// Constructor
public IntNode(int dataInit, IntNode nextLoc) {
this.dataVal = dataInit;
this.nextNodePtr = nextLoc;
}
/* Insert node after this node.
Before: this -- next
After: this -- node -- next
*/
public void insertAfter(IntNode nodeLoc) {
IntNode tmpNext;
tmpNext = this.nextNodePtr;
this.nextNodePtr = nodeLoc;
nodeLoc.nextNodePtr = tmpNext;
}
// Get location pointed by nextNodePtr
public IntNode getNext() {
return this.nextNodePtr;
}
// Get node value
public int getNodeData() {
return this.dataVal;
}
// Print node value
public void printNodeData() {
System.out.println(this.dataVal);
}
}
--------------------------------------------------------------------------------------------------------------------------------------------------------
public class CustomLinkedList {
// TODO: Return number of nodes in list
public static int getCount(IntNode headObj) {
/* insert your code here */
return
}
public static void main(String[] args) {
IntNode headObj;
IntNode currObj;
IntNode lastObj;
int i;
int count;
// Create head node
headObj = new IntNode(-1);
lastObj = headObj;
// Add nodes to the list
for (i = 0; i < 20; ++i) {
currObj = new IntNode(i);
lastObj.insertAfter(currObj);
lastObj = currObj;
}
count = getCount(headObj);
System.out.println(count);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

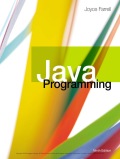
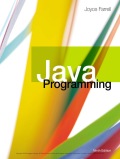