Loan Account Class: Create class LoanAccount. Use a static variable annuallnterestRate to store the annual interest rate for all account holders. Each object of the class contains a private instance variable principle indicating the amount the person is borrowing. Provide method: public double calculateMonthlyPayment(int numberOfPayments) to calculate the monthly payment by using the following formula: double monthlyPayment = principle * ( monthlylnterest / (1 - Math.pow(1 + monthlylnterest, - numberOfPayments)); where monthly interest = annuallnterestRate/12. Provide a static method setAnnuallnterestRate that sets the annuallnterestRate to a new value. Set the initial loan amount (Principle) for a new loan through the constructor. Write a program to test class LoanAccount. Instantiate two LoanAccount objects, loan1 and loan2, with principle loan amounts of $5000.00 and $31000.00, respectively. Set annuallnterestRate to 1%, then calculate the monthly payment for each loan for 36 months, 60 months, and 72 months and print the monthly payment amounts for both loans. Next, set the annuallnterestRate to 5%, and output the same information again. Make sure your dollar amounts are displayed to two decimal places. Put the code to test the class in the main method of the LoanAccount Class. The output of your program should look like the following: Monthly payments for loanl of $5000.00 and loan2 $31000.00 for 3, 5, and € year loans at 1 interest. Loan 3 years 5 years 6 years Loanl 141.04 85.47 71.58 Loan2 874.45 529.91 443.78 I Monthly payments for loanl of $5000.00 and loan2 $31000.00 for 3, 5, and € year loans at 54 interest. Loan 3 years 5 years 6 years Loanl 149.85 94.36 80.52 Loan2 929.10 585.01 499.25
Loan Account Class: Create class LoanAccount. Use a static variable annuallnterestRate to store the annual interest rate for all account holders. Each object of the class contains a private instance variable principle indicating the amount the person is borrowing. Provide method: public double calculateMonthlyPayment(int numberOfPayments) to calculate the monthly payment by using the following formula: double monthlyPayment = principle * ( monthlylnterest / (1 - Math.pow(1 + monthlylnterest, - numberOfPayments)); where monthly interest = annuallnterestRate/12. Provide a static method setAnnuallnterestRate that sets the annuallnterestRate to a new value. Set the initial loan amount (Principle) for a new loan through the constructor. Write a program to test class LoanAccount. Instantiate two LoanAccount objects, loan1 and loan2, with principle loan amounts of $5000.00 and $31000.00, respectively. Set annuallnterestRate to 1%, then calculate the monthly payment for each loan for 36 months, 60 months, and 72 months and print the monthly payment amounts for both loans. Next, set the annuallnterestRate to 5%, and output the same information again. Make sure your dollar amounts are displayed to two decimal places. Put the code to test the class in the main method of the LoanAccount Class. The output of your program should look like the following: Monthly payments for loanl of $5000.00 and loan2 $31000.00 for 3, 5, and € year loans at 1 interest. Loan 3 years 5 years 6 years Loanl 141.04 85.47 71.58 Loan2 874.45 529.91 443.78 I Monthly payments for loanl of $5000.00 and loan2 $31000.00 for 3, 5, and € year loans at 54 interest. Loan 3 years 5 years 6 years Loanl 149.85 94.36 80.52 Loan2 929.10 585.01 499.25
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter11: More Object-oriented Programming Concepts
Section: Chapter Questions
Problem 1GZ
Related questions
Question
I don't know what to do to dispay the output shown.
I have this far right now.
![Click nbf://nbhost/SystemFileSystem/Templates/Licenses/license-default.txt to change this license
Click nbfs://nbhost/SystemFileSystem/Templates/Classes/Class.java to edit this template
*/
package programmingassignmentone;
/**
@author sl
public class LoanAccount {
private static float annualInterestRate;
private double principle;
public LoanAccount (double principle) {
this.principle
principle;
public static void setAnnualInterestRate (double annualInterestRate)
{
LoanAccount.annualInterestRate = (float) annualInterestRate;
public double calculateMonthlyPayment (int numberOfPayments)
{
double monthlyInterest
annualInterestRate / 12;
double monthlyPayment
principle *
(monthlyInterest / (1 - Math.pow (1 + monthlyInterest, -number0fPayments))) ;
return monthlyPayment;
}
public static void main (String [] args) {
new LoanAccount (5000);
new LoanAccount (31000);
LoanAccount loan1
LoanAccount loan2 =](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1bb54c50-d653-47f8-8cfc-545a4ed262e0%2F7ba6f947-850f-4d6e-800e-ab60f06c19b5%2F2i0g5f8_processed.png&w=3840&q=75)
Transcribed Image Text:Click nbf://nbhost/SystemFileSystem/Templates/Licenses/license-default.txt to change this license
Click nbfs://nbhost/SystemFileSystem/Templates/Classes/Class.java to edit this template
*/
package programmingassignmentone;
/**
@author sl
public class LoanAccount {
private static float annualInterestRate;
private double principle;
public LoanAccount (double principle) {
this.principle
principle;
public static void setAnnualInterestRate (double annualInterestRate)
{
LoanAccount.annualInterestRate = (float) annualInterestRate;
public double calculateMonthlyPayment (int numberOfPayments)
{
double monthlyInterest
annualInterestRate / 12;
double monthlyPayment
principle *
(monthlyInterest / (1 - Math.pow (1 + monthlyInterest, -number0fPayments))) ;
return monthlyPayment;
}
public static void main (String [] args) {
new LoanAccount (5000);
new LoanAccount (31000);
LoanAccount loan1
LoanAccount loan2 =
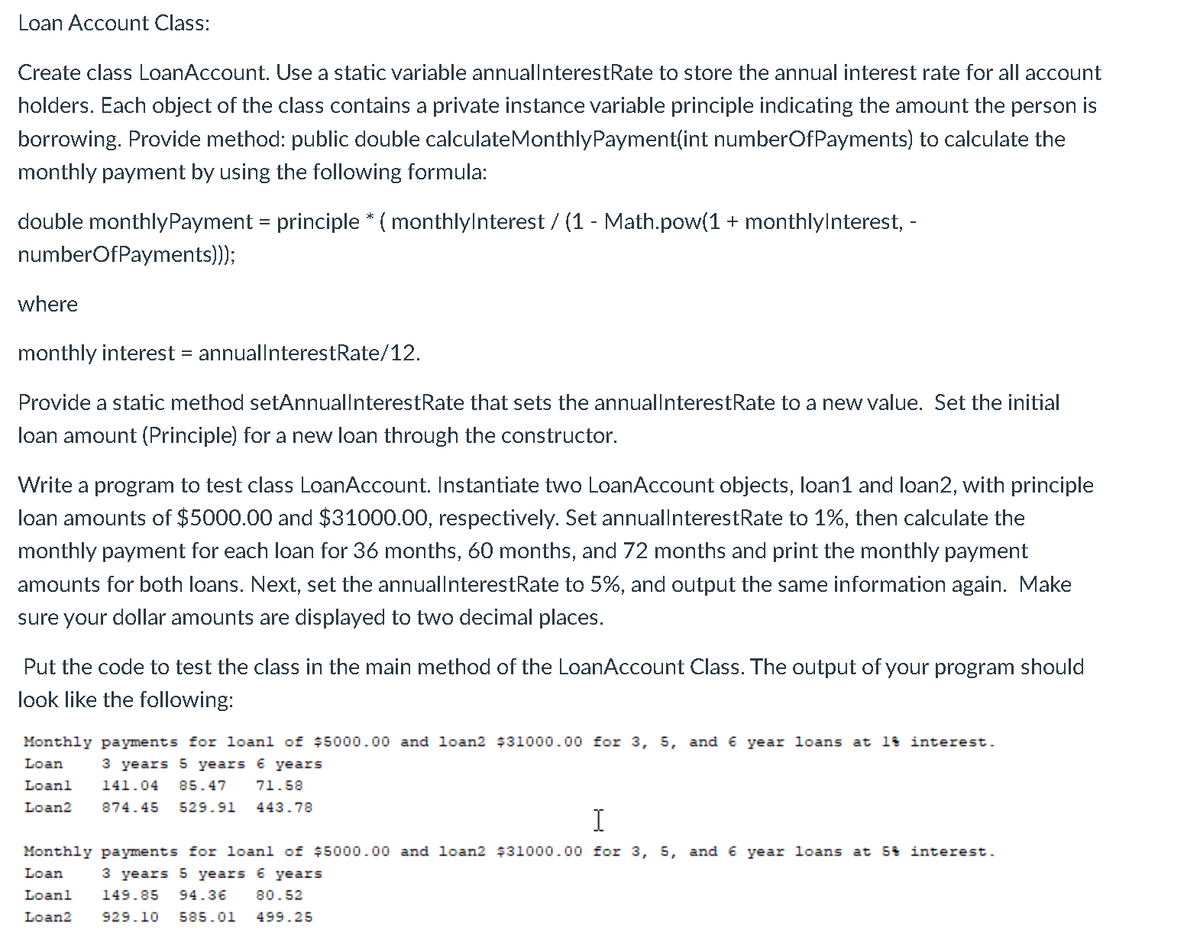
Transcribed Image Text:Loan Account Class:
Create class LoanAccount. Use a static variable annuallnterestRate to store the annual interest rate for all account
holders. Each object of the class contains a private instance variable principle indicating the amount the person is
borrowing. Provide method: public double calculateMonthlyPayment(int numberOfPayments) to calculate the
monthly payment by using the following formula:
double monthlyPayment = principle * ( monthlylnterest / (1 - Math.pow(1 + monthlylnterest, -
numberOfPayments));
where
monthly interest = annuallnterestRate/12.
%3D
Provide a static method setAnnuallnterestRate that sets the annuallnterestRate to a new value. Set the initial
loan amount (Principle) for a new loan through the constructor.
Write a program to test class LoanAccount. Instantiate two LoanAccount objects, loan1 and loan2, with principle
loan amounts of $5000.00 and $31000.00, respectively. Set annuallnterestRate to 1%, then calculate the
monthly payment for each loan for 36 months, 60 months, and 72 months and print the monthly payment
amounts for both loans. Next, set the annuallnterestRate to 5%, and output the same information again. Make
sure your dollar amounts are displayed to two decimal places.
Put the code to test the class in the main method of the LoanAccount Class. The output of your program should
look like the following:
Monthly payments for loanl of $5000.00 and loan2 $31000.00 for 3, 5, and 6 year loans at l interest.
Loan
3 years 5 years 6 years
Loanl
141.04
85.47
71.58
Loan2
874.45
529.91
443.78
I
Monthly payments for loanl of $5000.00 and loan2 $31000.00 for 3, 5, and 6 year loans at 5% interest.
Loan
3 years 5 years 6 years
Loanl
149.85
94.36
80.52
Loan2
929.10
585.01
499.25
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage