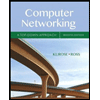
Use Java Programming Language
Loan Account Class:
Create class LoanAccount. Use a static variable annualInterestRate to store the annual interest rate for all account holders. Each object of the class contains a private instance variable principal indicating the amount the person is borrowing. Provide method: public double calculateMonthlyPayment(int numberOfPayments) to calculate the monthly payment by using the following formula:
double monthlyPayment = principal * ( monthlyInterest / (1 - Math.pow(1 + monthlyInterest, -numberOfPayments)));
where
monthly interest = annualInterestRate/12.
Provide a static method setAnnualInterestRate that sets the annualInterestRate to a new value. Set the initial loan amount (Principal) for a new loan through the constructor.
Write a program to test class LoanAccount. Instantiate two LoanAccount objects, loan1 and loan2, with principal loan amounts of $5000.00 and $31000.00, respectively. Set annualInterestRate to 1%, then calculate the monthly payment for each loan for 36 months, 60 months, and 72 months and print the monthly payment amounts for both loans. Next, set the annualInterestRate to 5%, and output the same information again. Make sure your dollar amounts are displayed to two decimal places.
Put the code to test the class in the main method of the LoanAccount Class. The output of your program should look like the following:


Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Java code public static named sumAllarrow_forward... in javaarrow_forwardPYTHON CODE WRITE SHORT DESCRIPTION OF DESIGN OF THE CODE Papa John, the owner of Papa Johns Pizzeria, needs help in keeping track of the number and types of pizzas he has cooked on a given day and to produce statistics regarding the choices her customers made. Your task is to write a Python application which will contain the following methods.. a main driver, that will: Display a welcome message Prompt Papa John for the maximum number of pizzas he can prepare on that day and create an empty list called todaysPizzasthat will have the potential of keeping track of that many DeluxePizza Display a main menu (fig 1) attached in images with the following choices. If Papa John enters an invalid choice keep prompting him until he enters a valid choice. When option 1 of the main menu is selected: Prompt user for the password. (Make sure you have a constant variable containing the password “deluxepizza” - don’t use any other password. John has 3 tries to enter the correct password. You…arrow_forward
- Javaarrow_forwardThis is the question - Create a class named Pizza with the following data fields: description - of type String price - of type double The description stores the type of pizza (such as sausage and onion). Include a constructor that requires arguments for both fields and a method named display to display the data. For example, if the description is 'sausage and onion' and the price is '14.99', the display method should output: sausage and onion pizza Price: $14.99 Create a subclass named DeliveryPizza that inherits from Pizza but adds the following data fields: deliveryFee - of type double address - of type String The description, price, and delivery address are required as arguments to the constructor. The delivery fee is $3 if the pizza ordered costs more than $15; otherwise it is $5. This is the code I have it won't accept - class DeliveryPizza extends Pizza { private double deliveryFee; private String address; public DeliveryPizza(String desc, double price, double deliveryFee,…arrow_forward1 a. is the amount the same, yes or no, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { balance = balance + amount ; } // modified toString() method public String toString() { return "Account: " + actNum + "\tName: " + nameOnAct + "\tBalance: " + amount ; } } b. public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { // scope of amount starts here balance = balance + amount ; // scope of amount ends here } public void processCheck( int amount ) { // scope of amount starts here int charge; incrementUse(); if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // scope of amount ends here } } c. is the…arrow_forward
- java Assignment Description: Write a class named Car that has the following fields: The yearModel field is an int that holds the car’s year model. The make field references a String object that holds the make of the car. The speed field is an int that holds the car’s current speed. In addition, the class should have the following constructor and other methods. The constructor should accept the car’s year and make as arguments. These values should be assigned to the object’s yearModel and make fields. The constructor should also assign 0 to the speed field. Constructor: The constructor should accept no arguments. Constructor: The constructor should accept the car’s year as argument.Assign the value to the object’s yearModel. No need to assign anything to the make and assign 0 to speed. Appropriate mutator methods should set the values in an object’s yearModel, make, and speed fields. Appropriate accessor methods should get the values stored in an object’s yearModel, make, and…arrow_forwardQ1. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { balance = balance + amount ; } // modified toString() method public String toString() { return "Account: " + actNum + "\tName: " + nameOnAct + "\tBalance: " + amount ; } } Q2. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { // scope of amount starts here balance = balance + amount ; // scope of amount ends here } public void processCheck( int amount ) { // scope of amount starts here int charge; incrementUse(); if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // scope of amount ends here }…arrow_forwardsolve in python Street ClassStep 1:• Create a class to store information about a street:• Instance variables should include:• the street name• the length of the street (in km)• the number of cars that travel the street per day• the condition of the street (“poor”, “fair”, or “good”).• Write a constructor (__init__), that takes four arguments corresponding tothe instance variables and creates one Street instance. Street ClassStep 2:• Add additional methods:• __str__• Should return a string with the Street information neatly-formatted, as in:Elm is 4.10 km long, sees 3000 cars per day, and is in poor condition.• compare:• This method should compare one Street to another, with the Street the method is calledon being compared to a second Street passed to a parameter.• Return True or False, indicating whether the first street needs repairs more urgently thanthe second.• Streets in “poor” condition need repairs more urgently than streets in “fair” condition, whichneed repairs more…arrow_forward
- IN JAVA Look at the following class definition: public class Dog {private int age = 0; // age is given in years, the default value is 0private String name = "Fido"; // default name is FidoDog(String firstName, int firstAge) {name = firstName;age = firstAge;if (age < 0) {age = 0;}}public void setName(String newName) {name = newName;}public int getAge() {return age;}public String getName() {return name;}public void haveBirthdayParty() {age = age + 1;System.out.println("Happy birthday " + name + "! You are now " + age + " years old!");}public void printInfo() {System.out.println("This dog's name is " + name + " and he's " + age + " years old!");}} Make a post containing your answers to all of the questions below: Can you create a new dog without specifying its name? Why or why not? Which of the Dog class's methods are accessors? Which ones are mutators? Can you use the methods provided to make a dog younger? (In other words, can you set age to a lower value than it already was?)…arrow_forwardpublic class Cat { private int age; public Cat (int a) { age = а; } public int getAge() { return a; } } Which of the following best explains why the getAge method will NOT work as intended? The instance variable age should be returned instead of a, which is local to the constructor. The variable age is not declared inside the getAge method. The getAge method should be declared as private. The getAge method should have at least one parameter.arrow_forwardWrite a member method for the Team class named : calculatePercentage that it will calculate and return the winning percentage of a team. The winning percentage is calculated as follows: wins / ( wins + losses)arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
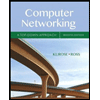
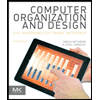
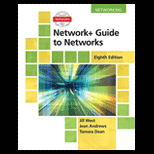
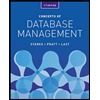
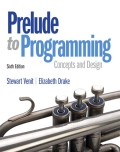
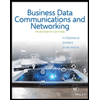