Ludo Ladders Game (C++)Structural code is given below of the project.Few parts of the code is removed and comments are added, you need to complete the code. #pragma warning(disable:4996) #include #include #include #include using namespace std; struct Ludo { int Game[100]; // This array contains the position of ladders and snakes in the game // If the position contains a +ve number then there is a ladder at that position // If the position contains a -ve number then there is a snake at that position int Turn = 0; int position[2]; int state = -1; }; int Roll() { /** use rand() function from Math.h This function will roll a dice. The rules for dice roll are as follows. If the player roles a six then he gets an other turn. If he rolls three sixes then his score becomes zero but if he rolls more than three sixes then all rolls count. */ srand(time(0)); int i; int n = 6; for (i = 0; n == 6; i++) { if (i == 0) { n = rand() % 6 + 1; } else if (n == 18) { n = 0; } else { n += rand() % 6 + 1; } } return n; } int Move(Ludo* G) { /* This function will roll a dice using the Roll() function defined above. It will then update the game status (i.e change the turn, update player position etc. */ int score; score = Roll(); if (G->Turn == 0) { G->position[0] = G->position[0] + score; if (G->Game[G->position[0]] > 0) { G->position[0] = G->position[0] + G->Game[G->position[0]]; if (G->position[0] + G->Game[G->position[0]] >= 100) { G->position[0] = 99; } } if (G->Game[G->position[0]] < 0) { G->position[0] = G->position[0] - G->Game[G->position[0]]; if (G->position[0] + G->Game[G->position[0]] < 0) { G->position[0] = 0; } } } if (G->Turn == 1) { G->position[1] = G->position[1] + score; if (G->Game[G->position[1]] > 0) { G->position[1] = G->position[1] + G->Game[G->position[1]]; if (G->position[1] + G->Game[G->position[1]] >= 100) { G->position[1] = 99; } } if (G->Game[G->position[1]] < 0) { G->position[1] = G->position[1] - G->Game[G->position[1]]; if (G->position[0] + G->Game[G->position[0]] < 0) { G->position[0] = 0; } } } if (G->position[0] == 99) { G->state = 1; return 0; } if (G->position[1] == 99) { G->state = 2; return 0; } if (G->Turn == 0) { G->Turn = 1; } else { G->Turn = 0; } return 0; } int show(Ludo G) { system("CLS"); cout << "\t\t\t\t\tTable\n\n"; //This function will show the game status on screen. //It will clear the screen first //Show 10 Numbers on a Line //Show Position of Each player on the Game //Show the Position of snake and ladder //Show the players Turn int i; for (i = 0; i < 100; i++) { if (i == G.position[0]) { if (i % 10 == 0 && i != 0) { cout << endl; cout << "A\t"; } else { cout << "A\t"; } } else if (i == G.position[1]) { if (i % 10 == 0 && i != 0) { cout << endl; cout << "B\t"; } else { cout << "B\t"; } } else if (i % 10 == 0) { cout << endl << G.Game[i] << "\t"; } else { cout << G.Game[i] << "\t"; } } cout << "\n\nPosition of player 1: " << G.position[0]; cout << "\n\nPosition of player 2: " << G.position[1]; if (G.state == -1) { if (G.Turn == 0) cout << "\n\n\t\tPlayer 1 turn."; else cout << "\n\n\t\tPlayer 2 turn."; } return 0; } void init(Ludo* game) { for (int i = 0; i< 10; i++) { int position = rand() % 100; int change = rand() % 10 + 1; if (position + change < 100) { game->Game[position] = change; } } for (int i = 0; i< 10; i++) { int position = rand() % 100; int change = rand() % 10 + 1; if (position - change > 0) { if (game->Game[position] == 0) { game->Game[position] = -change; } } } } int main() { Ludo newGame; int i; for (i = 0; i < 100; i++) { newGame.Game[i] = 0; } newGame.position[0] = 0; newGame.position[1] = 0; init(&newGame); cout << "\n\nPlayer 1 Turn"; while (newGame.state == -1) { show(newGame); cout << "\n\n\t\tPress Enter To make your turn "; getchar(); Move(&newGame); } if (newGame.state == 1) { show(newGame); cout << "\n\n\t\tPlayer 1 Wins.......\n\n"; } else if (newGame.state == 2) { show(newGame); cout << "\n\n\t\tPlayer 2 Wins.......\n\n"; } cout << "\t\t"; return 0; }
Ludo Ladders Game
(C++)Structural code is given below of the project.Few parts of the code is removed and comments are added, you need to complete the code.
#pragma warning(disable:4996)
#include <iostream>
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
using namespace std;
struct Ludo
{
int Game[100];
// This array contains the position of ladders and snakes in the game
// If the position contains a +ve number then there is a ladder at that position
// If the position contains a -ve number then there is a snake at that position
int Turn = 0;
int position[2];
int state = -1;
};
int Roll()
{
/**
use rand() function from Math.h
This function will roll a dice. The rules for dice roll are as follows.
If the player roles a six then he gets an other turn.
If he rolls three sixes then his score becomes zero but if he rolls more than three sixes then all rolls count.
*/
srand(time(0));
int i;
int n = 6;
for (i = 0; n == 6; i++)
{
if (i == 0)
{
n = rand() % 6 + 1;
}
else if (n == 18)
{
n = 0;
}
else
{
n += rand() % 6 + 1;
}
}
return n;
}
int Move(Ludo* G)
{
/*
This function will roll a dice using the Roll() function defined above.
It will then update the game status (i.e change the turn, update player position etc.
*/
int score;
score = Roll();
if (G->Turn == 0)
{
G->position[0] = G->position[0] + score;
if (G->Game[G->position[0]] > 0)
{
G->position[0] = G->position[0] + G->Game[G->position[0]];
if (G->position[0] + G->Game[G->position[0]] >= 100)
{
G->position[0] = 99;
}
}
if (G->Game[G->position[0]] < 0)
{
G->position[0] = G->position[0] - G->Game[G->position[0]];
if (G->position[0] + G->Game[G->position[0]] < 0)
{
G->position[0] = 0;
}
}
}
if (G->Turn == 1)
{
G->position[1] = G->position[1] + score;
if (G->Game[G->position[1]] > 0)
{
G->position[1] = G->position[1] + G->Game[G->position[1]];
if (G->position[1] + G->Game[G->position[1]] >= 100)
{
G->position[1] = 99;
}
}
if (G->Game[G->position[1]] < 0)
{
G->position[1] = G->position[1] - G->Game[G->position[1]];
if (G->position[0] + G->Game[G->position[0]] < 0)
{
G->position[0] = 0;
}
}
}
if (G->position[0] == 99)
{
G->state = 1;
return 0;
}
if (G->position[1] == 99)
{
G->state = 2;
return 0;
}
if (G->Turn == 0)
{
G->Turn = 1;
}
else
{
G->Turn = 0;
}
return 0;
}
int show(Ludo G)
{
system("CLS");
cout << "\t\t\t\t\tTable\n\n";
//This function will show the game status on screen.
//It will clear the screen first
//Show 10 Numbers on a Line
//Show Position of Each player on the Game
//Show the Position of snake and ladder
//Show the players Turn
int i;
for (i = 0; i < 100; i++)
{
if (i == G.position[0])
{
if (i % 10 == 0 && i != 0)
{
cout << endl;
cout << "A\t";
}
else
{
cout << "A\t";
}
}
else if (i == G.position[1])
{
if (i % 10 == 0 && i != 0)
{
cout << endl;
cout << "B\t";
}
else
{
cout << "B\t";
}
}
else if (i % 10 == 0)
{
cout << endl << G.Game[i] << "\t";
}
else
{
cout << G.Game[i] << "\t";
}
}
cout << "\n\nPosition of player 1: " << G.position[0];
cout << "\n\nPosition of player 2: " << G.position[1];
if (G.state == -1)
{
if (G.Turn == 0)
cout << "\n\n\t\tPlayer 1 turn.";
else
cout << "\n\n\t\tPlayer 2 turn.";
}
return 0;
}
void init(Ludo* game)
{
for (int i = 0; i< 10; i++)
{
int position = rand() % 100;
int change = rand() % 10 + 1;
if (position + change < 100)
{
game->Game[position] = change;
}
}
for (int i = 0; i< 10; i++)
{
int position = rand() % 100;
int change = rand() % 10 + 1;
if (position - change > 0)
{
if (game->Game[position] == 0)
{
game->Game[position] = -change;
}
}
}
}
int main()
{
Ludo newGame;
int i;
for (i = 0; i < 100; i++)
{
newGame.Game[i] = 0;
}
newGame.position[0] = 0;
newGame.position[1] = 0;
init(&newGame);
cout << "\n\nPlayer 1 Turn";
while (newGame.state == -1)
{
show(newGame);
cout << "\n\n\t\tPress Enter To make your turn ";
getchar();
Move(&newGame);
}
if (newGame.state == 1)
{
show(newGame);
cout << "\n\n\t\tPlayer 1 Wins.......\n\n";
}
else if (newGame.state == 2)
{
show(newGame);
cout << "\n\n\t\tPlayer 2 Wins.......\n\n";
}
cout << "\t\t";
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

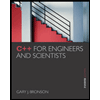
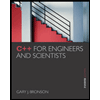