Marked Problems. Complete an implementation for the following prototype of a function that searches for a student with a given ID in an array of students. If the student was found, the function should return a pointer to the student's name. If no student with that ID exists in the array then the function should return NULL. Your function must run in O(n) time in the worst case and O(1) time in the best case where n is the number of student records in the array. // find_id(id, arr, n) searches for a student with given id in arr; // returns a pointer to the student's name or NULL if no student J1 has the given id // requires: arr has length n // students in arr have unique ids char * find_id(int id, struct student * arr[], int n); Complete an implementation for the "inverse" lookup, i.e., a function that searches for students with a given name. Note that many students might share the same name. Your function must run in O(nm) time where n is the number of student records in the array and m is the length of the longest student name. // find_name (name, arr, n, ids) searches for student (s) with given name in arr; // returns the number of students found and the array ids is updated to 11 contain the id numbers of those students
Marked Problems. Complete an implementation for the following prototype of a function that searches for a student with a given ID in an array of students. If the student was found, the function should return a pointer to the student's name. If no student with that ID exists in the array then the function should return NULL. Your function must run in O(n) time in the worst case and O(1) time in the best case where n is the number of student records in the array. // find_id(id, arr, n) searches for a student with given id in arr; // returns a pointer to the student's name or NULL if no student J1 has the given id // requires: arr has length n // students in arr have unique ids char * find_id(int id, struct student * arr[], int n); Complete an implementation for the "inverse" lookup, i.e., a function that searches for students with a given name. Note that many students might share the same name. Your function must run in O(nm) time where n is the number of student records in the array and m is the length of the longest student name. // find_name (name, arr, n, ids) searches for student (s) with given name in arr; // returns the number of students found and the array ids is updated to 11 contain the id numbers of those students
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![Marked Problems. Complete an implementation for the following prototype of a function that
searches for a student with a given ID in an array of students. If the student was found, the function
should return a pointer to the student's name. If no student with that ID exists in the array then the
function should return NULL. Your function must run in O(n) time in the worst case and O(1) time in
the best case where n is the number of student records in the array.
//
// find_id(id, arr, n) searches for a student with given id in arr;
returns a pointer to the student's name or NULL if no student
has the given id
//
// requires: arr has length n
//
char *
students in arr have unique ids
find_id (int id, struct student * arr[], int n) ;
Complete an implementation for the "inverse" lookup, i.e., a function that searches for students
with a given name. Note that many students might share the same name. Your function must run in
O(nm) time where n is the number of student records in the array and m is the length of the longest
student name.
// find_name (name, arr, n, ids) searches for student (s) with given name in arr;
returns the number of students found and the array ids is updated to
// contain the id numbers of those students
// requires: arr has length n
students in arr have unique ids
ids points to enough memory to hold the found student ids.
int find_name (char * name, struct student * arr [], int n, int ids []);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F971cf0c0-f175-43eb-81b0-784ad4b29e4a%2F8cdfbb42-1ada-438d-b3bc-a06894b15f29%2F4tgao4_processed.png&w=3840&q=75)
Transcribed Image Text:Marked Problems. Complete an implementation for the following prototype of a function that
searches for a student with a given ID in an array of students. If the student was found, the function
should return a pointer to the student's name. If no student with that ID exists in the array then the
function should return NULL. Your function must run in O(n) time in the worst case and O(1) time in
the best case where n is the number of student records in the array.
//
// find_id(id, arr, n) searches for a student with given id in arr;
returns a pointer to the student's name or NULL if no student
has the given id
//
// requires: arr has length n
//
char *
students in arr have unique ids
find_id (int id, struct student * arr[], int n) ;
Complete an implementation for the "inverse" lookup, i.e., a function that searches for students
with a given name. Note that many students might share the same name. Your function must run in
O(nm) time where n is the number of student records in the array and m is the length of the longest
student name.
// find_name (name, arr, n, ids) searches for student (s) with given name in arr;
returns the number of students found and the array ids is updated to
// contain the id numbers of those students
// requires: arr has length n
students in arr have unique ids
ids points to enough memory to hold the found student ids.
int find_name (char * name, struct student * arr [], int n, int ids []);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
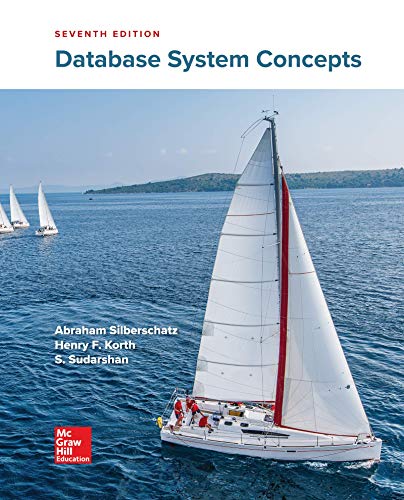
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
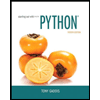
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
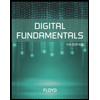
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
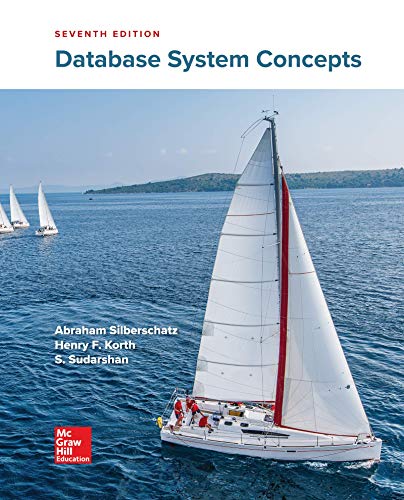
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
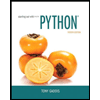
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
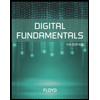
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
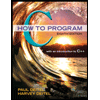
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
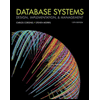
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
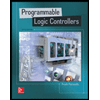
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education