Modify class Time2 (pasted below) to include a tick method that increments the time stored in a Time2 object by one second. Provide method incrementMinute to increment the minute by one and method incrementHour to increment the hour by one. Write a program that tests the tick method, the incrementMinute method and the incrementHour method to ensure that they work correctly. Be sure to test the following cases: a. incrementing into the next minute, b. incrementing into the next hour and
Modify class Time2 (pasted below) to include a tick method that increments the time stored in a Time2 object by one second. Provide method incrementMinute to increment the minute by one and method incrementHour to increment the hour by one. Write a program that tests the tick method, the incrementMinute method and the incrementHour method to ensure that they work correctly. Be sure to test the following cases:
a. incrementing into the next minute,
b. incrementing into the next hour and
c. incrementing into the next day (i.e., 11:59:59 PM to 12:00:00 AM).
public class Time2 {
private int hour; // 0 - 23
private int minute; // 0 - 59
private int second; // 0 - 59
//no-argument constructor that initializes each instance variable to zero
public Time2() {
this(0, 0, 0); //invoke constructor with three arguments
}
//constructor, hour supplied, min and sec defaulted to 0
public Time2(int hour) {
this(hour, 0, 0); //invoke constructor
}
//hour and min supplied
public Time2(int hour, int minute) {
this(hour, minute, 0);
}
//hour, min, and sec supplied
public Time2(int hour, int minute, int second) {
if (hour < 0 || hour >= 24) {
throw new IllegalArgumentException("hour must be 0-23");
}
if (minute < 0 || minute >= 60) {
throw new IllegalArgumentException("minute must be 0-59");
}
if (second < 0 || second >= 60) {
throw new IllegalArgumentException("second must be 0-59");
}
this.hour = hour;
this.minute = minute;
this.second = second;
}
public Time2(Time2 time) {
//invoke constructor with 3 arguments
this(time.hour, time.minute, time.second);
}
//set methods
//set a new time value using universal time
public void setTime(int hour, int minute, int second) {
if (hour < 0 || hour >= 24) {
throw new IllegalArgumentException("hour must be 0-23");
}
if (minute < 0 || minute >= 60) {
throw new IllegalArgumentException("minute must be 0-59");
}
if (second < 0 || second >= 60) {
throw new IllegalArgumentException("second must be 0-59");
}
this.hour = hour;
this.minute = minute;
this.second = second;
}
//validate and set hour
public void setHour(int hour) {
if(hour < 0 || hour >= 24) {
throw new IllegalArgumentException("hour must be 0-23");
}
this.hour = hour;
}
public void setMinute(int minute) {
if (minute < 0 || minute >= 60) {
throw new IllegalArgumentException("minute must be 0-59");
}
this.minute = minute;
}
public void setSecond(int second) {
if (second < 0 || second >= 60) {
throw new IllegalArgumentException("second must be 0-59");
}
this.second = second;
}
//get methods
public int getHour() {return hour;}
public int getMinute() {return minute;}
public int getSecond() {return second;}
//convert to universal-time format
public String toUniversalString() {
return String.format("%02d:%02d:%02d", getHour(), getMinute(), getSecond());
}
public String toString() {
return String.format("%02d:%02d:%02d", ((getHour() == 0 || getHour() == 12) ? 12 : getHour() % 12), getMinute(), getSecond(), (getHour() < 12 ? "AM" : "PM"));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

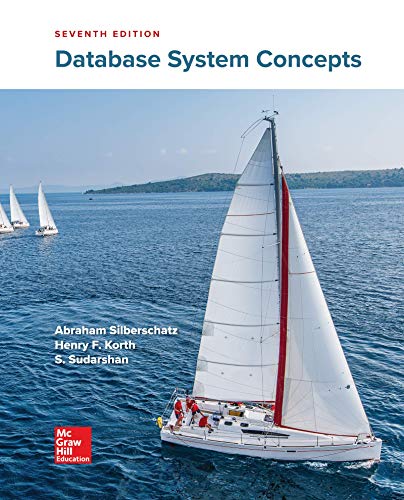
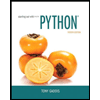
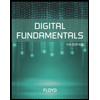
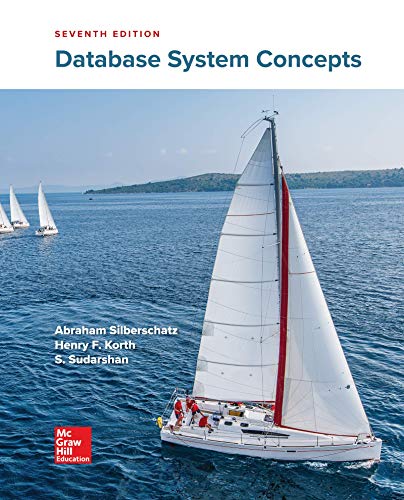
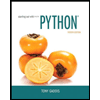
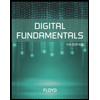
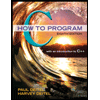
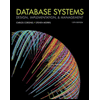
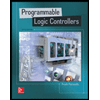