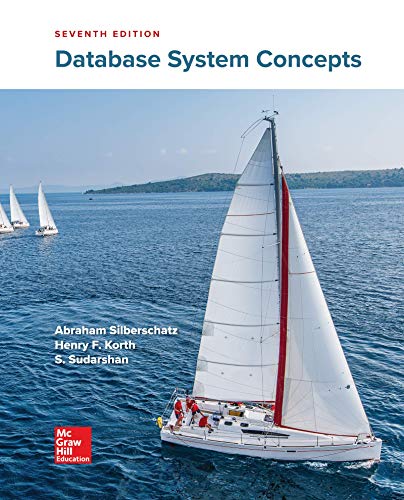
Using the Card.java class file, write a program to simulate a Deck of Cards. See
public class Card
{
public final static int ACE = 1;
public final static int TWO = 2;
public final static int THREE = 3;
public final static int FOUR = 4;
public final static int FIVE = 5;
public final static int SIX = 6;
public final static int SEVEN = 7;
public final static int EIGHT = 8;
public final static int NINE = 9;
public final static int TEN = 10;
public final static int JACK = 11;
public final static int QUEEN = 12;
public final static int KING = 13;
public final static int CLUBS = 1;
public final static int DIAMONDS = 2;
public final static int HEARTS = 3;
public final static int SPADES = 4;
private final static int NUM_FACES = 13;
private final static int NUM_SUITS = 4;
private int face, suit;
private String faceName, suitName;
//-----------------------------------------------------------------
// Creates a random card.
//-----------------------------------------------------------------
public Card()
{
face = (int) (Math.random() * NUM_FACES) + 1;
setFaceName();
suit = (int) (Math.random() * NUM_SUITS) + 1;
setSuitName();
}
//-----------------------------------------------------------------
// Creates a card of the specified suit and face value.
//-----------------------------------------------------------------
public Card(int faceValue, int suitValue)
{
face = faceValue;
setFaceName();
suit = suitValue;
setSuitName();
}
//-----------------------------------------------------------------
// Sets the string representation of the face using its stored
// numeric value.
//-----------------------------------------------------------------
private void setFaceName()
{
switch (face)
{
case ACE:
faceName = "Ace";
break;
case TWO:
faceName = "Two";
break;
case THREE:
faceName = "Three";
break;
case FOUR:
faceName = "Four";
break;
case FIVE:
faceName = "Five";
break;
case SIX:
faceName = "Six";
break;
case SEVEN:
faceName = "Seven";
break;
case EIGHT:
faceName = "Eight";
break;
case NINE:
faceName = "Nine";
break;
case TEN:
faceName = "Ten";
break;
case JACK:
faceName = "Jack";
break;
case QUEEN:
faceName = "Queen";
break;
case KING:
faceName = "King";
break;
}
}
//-----------------------------------------------------------------
// Sets the string representation of the suit using its stored
// numeric value.
//-----------------------------------------------------------------
private void setSuitName()
{
switch (suit)
{
case CLUBS:
suitName = "Clubs";
break;
case DIAMONDS:
suitName = "Diamonds";
break;
case HEARTS:
suitName = "Hearts";
break;
case SPADES:
suitName = "Spades";
break;
}
}
//-----------------------------------------------------------------
// Determines if this card is higher than the parameter. The
// second parameter determines if aces should be considered high
// (beats a King) or low (lowest of all faces). Uses the suit
// if both cards have the same face.
//-----------------------------------------------------------------
public boolean isHigherThan(Card card2, boolean aceHigh)
{
boolean result = false;
if (face == card2.getFace())
{
if (suit > card2.getSuit())
result = true;
}
else
{
if (aceHigh && face == ACE)
result = true;
else
if (face > card2.getFace())
result = true;
}
return result;
}
//-----------------------------------------------------------------
// Determines if this card is higher than the passed card,
// assuming that aces should be considered high.
//-----------------------------------------------------------------
public boolean isHigherThan(Card card2)
{
return isHigherThan(card2, true);
}
//-----------------------------------------------------------------
// Returns the face (numeric value) of this card.
//-----------------------------------------------------------------
public int getFace()
{
return face;
}
//-----------------------------------------------------------------
// Returns the suit (numeric value) of this card.
//-----------------------------------------------------------------
public int getSuit()
{
return suit;
}
//-----------------------------------------------------------------
// Returns the face (string value) of this card.
//-----------------------------------------------------------------
public String getFaceName()
{
return faceName;
}
//-----------------------------------------------------------------
// Returns the suit (string value) of this card.
//-----------------------------------------------------------------
public String getSuitName()
{
return suitName;
}
//-----------------------------------------------------------------
// Returns the string representation of this card, including
// both face and suit.
//-----------------------------------------------------------------
public String toString()
{
return faceName + " of " + suitName;
}
}
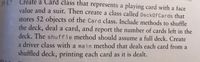

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Develop a Java program and run it for the following: the program asks the user to enter students' grades for three courses. The first course contains 5 students, the second 7 students. For the third course, the user input the grades until -1 entered (use while loop). Use three types of loops (for-loop, while-loop, do-while) for the three courses. Then output/print the average grade of each one of the three classes. {note: this will be a complete program with one class and one method in the class, the main method}. Submit one sample run (screenshot).arrow_forwardThis may make more sense if I show you a sample run of a program that implements the wizard shown above: Do you want to buy a snowboard? no Do you want to buy downhill skis? yes Have you gone skiing before? yes Are you an expert? no Buy the ZR200 model. Notice how a series of questions is asked by the wizard and answered by the shopper. When there are no more questions to be asked, the conclusion is printed by the wizard and the program exits. The series of questions asked by the wizard closely follows the rules in the flow chart. A Decision object represents a triangle in the flow cart. The Decision object asks a question and then returns either the next decision in the flow chart, or a message that should be printed before the program terminates. This is an example of the Composite design pattern because a Decision object contains other Decision objects. Here is a description of the methods in the IDecision interface: public void setYes(IDecision yes): Specifies the IDecision that…arrow_forwardOne last implementation, can you have the user implement their own names and employee info (like first and last name, # of hours worked, hourly rate, and their major) and it computes it for them and prints it out?arrow_forward
- Write a Python application for the following scenario. You have some students in your Introduction to Python Programming class. Write an interactive program that asks the user to input the names of each student and then their grades. Each student inputs three grades (Exam 1, 2 and 3 (you can create categories of your choice)). Calculate the total scores of each student. With the data that you have collected, please create a graph (pie chart or column charts) with the information of your students. Try to use data labels for your program. Please follow the rubric and submit everything as required. Hint: Use any of the concepts that you have learnt through the course. You can use methods, lists, or anything that you would find necessary to create the solution.arrow_forwardFor debugging, there are benefits and drawbacks to using print statements and manual walkthroughs. Discuss.arrow_forwardProgramming language is C. I would really be appriciate if you could help me with this. Please provide me with your cashapp, so I can tip you for your work. THANK YOU:) In this project, we shall simulate the operations of an ATM machine. Suppose you’re in charge of this simulation and here is a scenario of what is required to do: The customer will be assigned a random or fixed number for his/her balance. First, the customer is prompted to enter his personal identification number pin (for this case study, we test only if this pin is formed by 4 digits! otherwise, a message like “Invalid PIN, try again . . .” will be displayed) and the user is re-prompted to enter the pin. The customer is given three chances to enter his pin. If he/she fails during the three trials you display a message like “Sorry you can’t continue, contact your bank for assistance!” If the pin is correct (formed by 4 digits), then the system will ask the customer for the receipt ( 1 for YES and 2 for NO ) and a menu…arrow_forward
- In java and UML diagram, Please show output and comment code out Source file: Each public class must be contained in a separate Java source file. Only one source file will have a main() method and this source will be named BlackjackGameSimulator.java. Other source/class names are up to you following the guidelines specified so far in the course. The format of the Java source must meet the general Java coding style guidelines discussed so far during the course. Pay special attention to naming guidelines, use of appropriate variable names and types, variable scope (public, private, protected, etc.), indentation, and comments. Classes and methods should be commented with JavaDoc-style comments (see below). Please use course office hours or contact the instructor directly if there are any coding style questions. JavaDocs: Sources should be commented using JavaDoc-style comments for classes and methods. Each class should have a short comment on what it represents and use the @author…arrow_forwardTHIS IS MEANT TO BE IN JAVA. What we've learned so far is variables, loops, and we just started learning some array today. The assignment is to get an integer from input, and output that integer squared, ending with newline. But i've been given some instructions that are kind of confusing to me. Please explain why and please show what the end result is. Here are some extra things i've been told to do with the small amount of code i've already written... Type 2 in the input box, then run the program so I can note that the answer is 4 Type 3 in the input box instead, run, and note the output is 6. Change the output statement to output a newline: System.out.println(userNumSquared);. Type 2 in the input box Change the program to use * rather than +, and try running with input 2 (output is 4) and 3 (output is now 9, not 6 as before). This is what I have so far... import java.util.Scanner; public class NumSquared {public static void main(String[] args) {Scanner scnr = new…arrow_forwardMake a Java electronic voting program. There should be 4 total parties(people you can vote for) use object-oriented code and output your final result to a text file. Some things to consider: Well implemented variables, data structures, well-running codearrow_forward
- Utilize Salary.java. The file contains a program that shows how a manager decides an employee’s salary. Two input values are read in: an employee’s current salary a rating of the employee’s performance // ************************************************************// Salary.java// ************************************************************import java.util.Scanner;import java.text.NumberFormat;public class Salary{ public static void main (String[] args) { double currentSalary; // employee's current salary double raise; // amount of the raise double newSalary; // new salary for the employee int rating; // performance rating Scanner scan = new Scanner(System.in); System.out.print ("Enter the current salary: "); currentSalary = scan.nextDouble(); System.out.print ("Enter the performance rating (3 is Excellent, 2 is Good, or 1 is Poor): "); rating = scan.nextInt(); // Enter the code here:…arrow_forwardIn Java, design a class that models a 6-sided die. The die will be used in a game. Simulate a roll of the die, return a random value from {1,2,3,4,5,6}.arrow_forwardProgramming language: JAVA: Processing Exercise 2. In section 7.3, you made a ball orbit the mouse. Make the ball orbit in an ellipse instead of a circle. You can do this by using a different radius for the x and for the y calculations. Make multiple balls orbit the mouse at different speeds. E.g., make three. Make one move in the opposite direction (counter-clockwise). Example in 7.3 codes attached with a photo. Cannot use advanced coding! we have only learned active processing, user defined functions, float, simple trig so fararrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
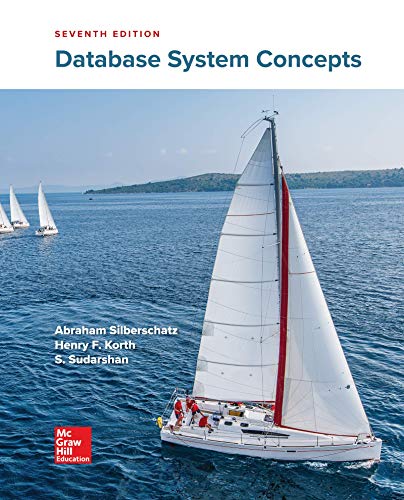
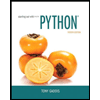
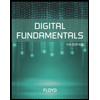
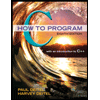
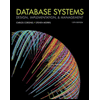
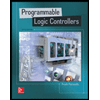