Modify the simulate() method in the Simulator class to work with the revised interface of ParkingLot and the new peek() method in Queue. In addition, implement the getIncomingQueueSize() method of Simulator using the size() method of Queue. The getIncomingQueueSize() is going to be used in the CapacityOptimizer class (next task) to determine the size of the incoming queue after a simulation run. CODE TO MODIFY IN JAVA: public class Simulator { /** * Length of car plate numbers */ public static final int PLATE_NUM_LENGTH = 3; /** * Number of seconds in one hour */ public static final int NUM_SECONDS_IN_1H = 3600; /** * Maximum duration a car can be parked in the lot */ public static final int MAX_PARKING_DURATION = 8 * NUM_SECONDS_IN_1H; /** * Total duration of the simulation in (simulated) seconds */ public static final int SIMULATION_DURATION = 24 * NUM_SECONDS_IN_1H; /** * The probability distribution for a car leaving the lot based on the duration * that the car has been parked in the lot */ public static final TriangularDistribution departurePDF = new TriangularDistribution(0, MAX_PARKING_DURATION / 2, MAX_PARKING_DURATION); /** * The probability that a car would arrive at any given (simulated) second */ private Rational probabilityOfArrivalPerSec; /** * The simulation clock. Initially the clock should be set to zero; the clock * should then be incremented by one unit after each (simulated) second */ private int clock; /** * Total number of steps (simulated seconds) that the simulation should run for. * This value is fixed at the start of the simulation. The simulation loop * should be executed for as long as clock < steps. When clock == steps, the * simulation is finished. */ private int steps; /** * Instance of the parking lot being simulated. */ private ParkingLot lot; /** * Queue for the cars wanting to enter the parking lot */ private Queue incomingQueue; /** * Queue for the cars wanting to leave the parking lot */ private Queue outgoingQueue; /** * @param lot is the parking lot to be simulated * @param steps is the total number of steps for simulation */ public Simulator(ParkingLot lot, int perHourArrivalRate, int steps) { throw new UnsupportedOperationException("This method has not been implemented yet!"); } /** * Simulate the parking lot for the number of steps specified by the steps * instance variable * NOTE: Make sure your implementation of simulate() uses peek() from the Queue interface. */ public void simulate() { throw new UnsupportedOperationException("This method has not been implemented yet!"); } public int getIncomingQueueSize() { throw new UnsupportedOperationException("This method has not been implemented yet!"); } }
Modify the simulate() method in the Simulator class to work with the revised interface of ParkingLot and the new peek() method in Queue. In addition, implement the getIncomingQueueSize() method of Simulator using the size() method of Queue. The getIncomingQueueSize() is going to be used in the CapacityOptimizer class (next task) to determine the size of the incoming queue after a simulation run. CODE TO MODIFY IN JAVA: public class Simulator { /** * Length of car plate numbers */ public static final int PLATE_NUM_LENGTH = 3; /** * Number of seconds in one hour */ public static final int NUM_SECONDS_IN_1H = 3600; /** * Maximum duration a car can be parked in the lot */ public static final int MAX_PARKING_DURATION = 8 * NUM_SECONDS_IN_1H; /** * Total duration of the simulation in (simulated) seconds */ public static final int SIMULATION_DURATION = 24 * NUM_SECONDS_IN_1H; /** * The probability distribution for a car leaving the lot based on the duration * that the car has been parked in the lot */ public static final TriangularDistribution departurePDF = new TriangularDistribution(0, MAX_PARKING_DURATION / 2, MAX_PARKING_DURATION); /** * The probability that a car would arrive at any given (simulated) second */ private Rational probabilityOfArrivalPerSec; /** * The simulation clock. Initially the clock should be set to zero; the clock * should then be incremented by one unit after each (simulated) second */ private int clock; /** * Total number of steps (simulated seconds) that the simulation should run for. * This value is fixed at the start of the simulation. The simulation loop * should be executed for as long as clock < steps. When clock == steps, the * simulation is finished. */ private int steps; /** * Instance of the parking lot being simulated. */ private ParkingLot lot; /** * Queue for the cars wanting to enter the parking lot */ private Queue incomingQueue; /** * Queue for the cars wanting to leave the parking lot */ private Queue outgoingQueue; /** * @param lot is the parking lot to be simulated * @param steps is the total number of steps for simulation */ public Simulator(ParkingLot lot, int perHourArrivalRate, int steps) { throw new UnsupportedOperationException("This method has not been implemented yet!"); } /** * Simulate the parking lot for the number of steps specified by the steps * instance variable * NOTE: Make sure your implementation of simulate() uses peek() from the Queue interface. */ public void simulate() { throw new UnsupportedOperationException("This method has not been implemented yet!"); } public int getIncomingQueueSize() { throw new UnsupportedOperationException("This method has not been implemented yet!"); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Modify the simulate() method in the Simulator class to work with the revised interface of ParkingLot and the new peek() method in Queue. In addition, implement the getIncomingQueueSize() method of Simulator using the size() method of Queue. The getIncomingQueueSize() is going to be used in the CapacityOptimizer class (next task) to determine the size of the incoming queue after a simulation run.
CODE TO MODIFY IN JAVA:
public class Simulator { /** * Length of car plate numbers */ public static final int PLATE_NUM_LENGTH = 3; /** * Number of seconds in one hour */ public static final int NUM_SECONDS_IN_1H = 3600; /** * Maximum duration a car can be parked in the lot */ public static final int MAX_PARKING_DURATION = 8 * NUM_SECONDS_IN_1H; /** * Total duration of the simulation in (simulated) seconds */ public static final int SIMULATION_DURATION = 24 * NUM_SECONDS_IN_1H; /** * The probability distribution for a car leaving the lot based on the duration * that the car has been parked in the lot */ public static final TriangularDistribution departurePDF = new TriangularDistribution(0, MAX_PARKING_DURATION / 2, MAX_PARKING_DURATION); /** * The probability that a car would arrive at any given (simulated) second */ private Rational probabilityOfArrivalPerSec; /** * The simulation clock. Initially the clock should be set to zero; the clock * should then be incremented by one unit after each (simulated) second */ private int clock; /** * Total number of steps (simulated seconds) that the simulation should run for. * This value is fixed at the start of the simulation. The simulation loop * should be executed for as long as clock < steps. When clock == steps, the * simulation is finished. */ private int steps; /** * Instance of the parking lot being simulated. */ private ParkingLot lot; /** * Queue for the cars wanting to enter the parking lot */ private Queue<Spot> incomingQueue; /** * Queue for the cars wanting to leave the parking lot */ private Queue<Spot> outgoingQueue; /** * @param lot is the parking lot to be simulated * @param steps is the total number of steps for simulation */ public Simulator(ParkingLot lot, int perHourArrivalRate, int steps) { throw new UnsupportedOperationException("This method has not been implemented yet!"); } /** * Simulate the parking lot for the number of steps specified by the steps * instance variable * NOTE: Make sure your implementation of simulate() uses peek() from the Queue interface. */ public void simulate() { throw new UnsupportedOperationException("This method has not been implemented yet!"); } public int getIncomingQueueSize() { throw new UnsupportedOperationException("This method has not been implemented yet!"); } }Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
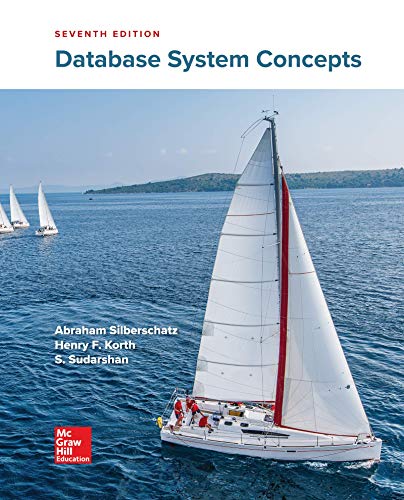
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
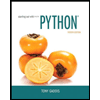
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
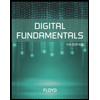
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
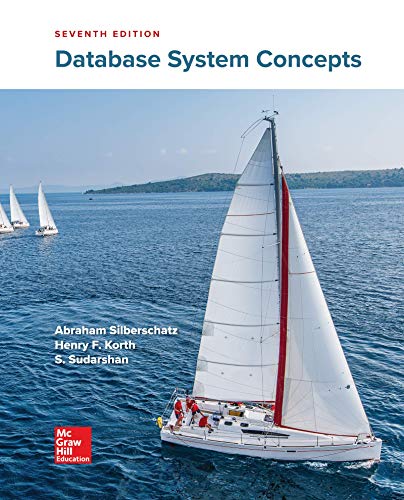
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
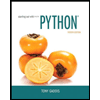
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
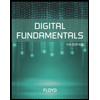
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
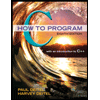
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
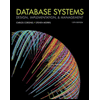
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
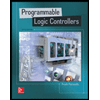
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education