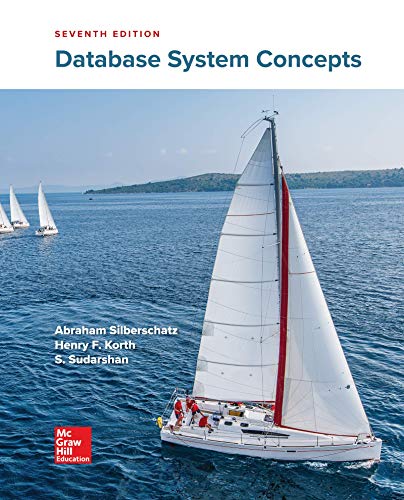
Concept explainers
Implement in C Programming
6.10.1: Function pass by pointer: Transforming coordinates.
Define a function CoordTransform() that transforms its first two input parameters xVal and yVal into two output parameters xValNew and yValNew. The function returns void. The transformation is new = (old + 1) * 2. Ex: If xVal = 3 and yVal = 4, then xValNew is 8 and yValNew is 10.
#include <stdio.h>
/* Your solution goes here */
int main(void) {
int xValNew;
int yValNew;
int xValUser;
int yValUser;
scanf("%d", &xValUser);
scanf("%d", &yValUser);
CoordTransform(xValUser, yValUser, &xValNew, &yValNew);
printf("(%d, %d) becomes (%d, %d)\n", xValUser, yValUser, xValNew, yValNew);
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Implement C Programming 7.6.1: LAB: Simple car Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar variable that performs the following operations: Drives input number of miles forward Drives input number of miles in reverse Honks the horn Reports car status SimpleCar.h contains the struct definition and related function declarations. SimpleCar.c contains related function definitions. Ex: If the input is: 100 4 the output is: beep beep Car has driven: 96 miles #include <stdio.h> #include "SimpleCar.h" int main() { /* Type your code here. */ return 0;}arrow_forwardWrite a c++ function reportDuplicates() that takes a two dimensional integer array as a parameter and identifies the duplicate valuesin the array. The function then reports these to user. Sample input and corresponding output:How many rows? 5How many columns? 2Let’s populate the array:1 86 97 312 522 4Thank you, there are no duplicate elements!How many rows? 3How many columns? 4Let’s populate the array:3 7 5 76 9 7 38 5 12 6Thank you, 3 appears 2 times, 7 appears 3 times, 5 appears 2 times,and 6 appears 2 times.arrow_forwardImplement in C Programming 7.6.1: LAB: Simple car Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar variable that performs the following operations: Drives input number of miles forward Drives input number of miles in reverse Honks the horn Reports car status SimpleCar.h contains the struct definition and related function declarations. SimpleCar.c contains related function definitions. Ex: If the input is: 100 4 the output is: beep beep Car has driven: 96 miles main.c #include <stdio.h> #include "SimpleCar.h" int main() { /* Type your code here. */ return 0;} SimpleCar.h #ifndef SIMPLE_CAR_H#define SIMPLE_CAR_H typedef struct SimpleCar_struct { int miles;} SimpleCar; SimpleCar InitCar();SimpleCar Drive(int dist, SimpleCar car);SimpleCar Reverse(int dist, SimpleCar car);int GetOdometer(SimpleCar car);void HonkHorn(SimpleCar car);void Report(SimpleCar car); #endif SimpleCar.c #include…arrow_forward
- Write a C++ program with two user defined functions. The first function named "functionWithArray" takes two user input arguments of character types and return True if first argument is smaller than the second argument (alphabetically) and returns False otherwise. Write the second function named "functionWithPointers" which behaves like the first functions but uses pointers to receive the arguments. You may assume that both character arrays contain only lower-case letters, and no blanks or other non- alphabetic characters. Write a suitable Main function to test these two functions. Sample Output: Enter First String: C++Programming Enter Second String: JavaProgramming According to functionWithArrays: 'c++programming' is smaller than 'javaprogramming'. According to functionWithPointers: 'c++programming' is smaller than 'javaprogramming'.arrow_forwardThis is in c++. Create a class of function objects called StartsWith that satisfies the following specification: when initialized with character c, an object of this class behaves as a unary predicate that determines if its string argument starts with c. For example, StartsWith(’a’) is a function object that can be used as a unary predicate to determine if a string starts with an a. So, StartsWith(’a’)("alice") would return true but StartsWith(’a’)("bob") would return false. The function objects should return false when called on an empty string. Test your function objects by using one of them together with the STL algorithm count_if to count the number of strings that start with some letter in some vector of strings. So basically, I want a class that returns true or false based on if the name that is typed starts with the character that I put down.arrow_forwardImplement C Programming 7.7.1: LAB: Vending machine Given two integers as user inputs that represent the number of drinks to buy and the number of bottles to restock, create a VendingMachine variable that performs the following operations: Purchases input number of drinks Restocks input number of bottles Reports inventory VendingMachine.h contains the struct definition and related function declarations. VendingMachine.c contains related function definitions. A VendingMachine's initial inventory is 20 drinks. Ex: If the input is: 5 2 the output is: Inventory: 17 bottles #include <stdio.h> #include "VendingMachine.h" int main() { /* Type your code here. */ return 0;}arrow_forward
- Write a program that will sort a prmiitive array of data using the following guidelines - DO NOT USE VECTORS, COLLECTIONS, SETS or any other data structures from your programming language. The codes for both the money and currency should stay the same, the main focus should be on the main file code (Programming language Java) Create a helper function called 'RecurInsSort' such that: It is a standalone function not part of any class from the prior lab or any new class you feel like creating here, Takes in the same type of parameters as any standard Insertion Sort with recursion behavior, i.e. void RecurInsSort(Currency arr[], int size) Prints out how the array looks every time a recursive step returns back to its caller The objects in the array should be Money objects added or manipulated using Currency references/pointers. It is OK to print out the array partially when returning from a particular step as long as the process of sorting is clearly demonstrated in the output. In…arrow_forwardSolve the Problem in C++ (invalid_argument )Listing 6.18 gives the hex2Dec(const string & hexString) function that returns a decimal number from a hex string. Implement the hex2Dec function to throw a invalid_argument exception if the string is not a hex string.Write a test program that prompts the user to enter a hex number as a string and display the number in decimal. If the function throws an exception, display "Not a hex number".arrow_forwardImplement in C Programing 7.10.1: LAB: Product struct Given main(), build a struct called Product that will manage product inventory. Product struct has three data members: a product code (string), the product's price (double), and the number count of product in inventory (int). Assume product code has a maximum length of 20. Implement the Product struct and related function declarations in Product.h, and implement the related function definitions in Product.c as listed below: Product InitProduct(char *code, double price, int count) - set the data members using the three parameters Product SetCode(char *code, Product product) - set the product code (i.e. SKU234) to parameter code void GetCode(char *productCode, Product product) - return the product code in productCode Product SetPrice(double price, Product product) - set the price to parameter product double GetPrice(Product product) - return the price Product SetCount(int count, Product product) - set the number of items in inventory…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
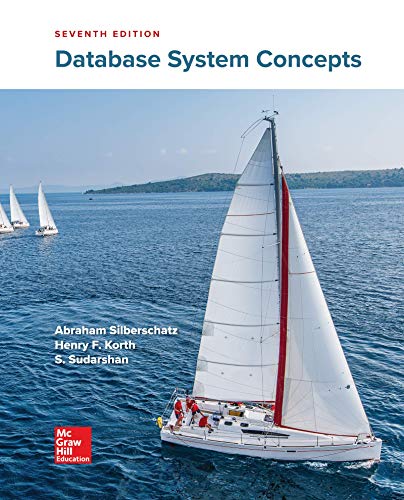
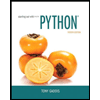
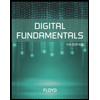
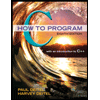
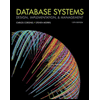
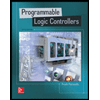