MyFileWriterTest.java import static org.junit.Assert.assertEquals; import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import org.junit.jupiter.api.BeforeEach; import org.junit.jupiter.api.Test; public class MyFileWriterTest { MyFileWriter myFileWriter1; MyFileWriter myFileWriter2; MyFileWriter myFileWriter3; MyFileWriter myFileWriter4; @BeforeEach void setUp() { this.myFileWriter1 =new MyFileWriter("test1_fw.txt"); // file with multiple words per line this.myFileWriter2 =new MyFileWriter("test2_fw.txt"); // similar to info.txt file this.myFileWriter3 =new MyFileWriter("test3_fw.txt"); // similar to info.txt file but with personal info added this.myFileWriter4 =new MyFileWriter("test4_fw.txt"); // similar to info.txt file but with different info and personal info added } @Test publicvoid testWriteToFile() { ArrayList actualLines = new ArrayList(); // Test file with multiple words per line actualLines.add("hello world"); actualLines.add("Course Name and ID"); actualLines.add("The quick brown fox jumps over the lazy dog."); // Write to file myFileWriter1.writeToFile(actualLines); // Read the written file to test its contents ArrayList expectedLines = readWrittenFile("test1_fw.txt"); assertEquals(expectedLines, actualLines); // Test original info.txt file actualLines.removeAll(actualLines); actualLines.add("Course: MCIT_590"); actualLines.add("CourseID: 590"); actualLines.add("StudentID: 101"); // Write to file myFileWriter2.writeToFile(actualLines); // Read the written file to test its contents expectedLines = readWrittenFile("test2_fw.txt"); assertEquals(expectedLines, actualLines); // TODO write at least 2 additional test cases using different MyFileWriters // Recommended: A test similar to info.txt file but with personal info added // Recommended: A test similar to info.txt file but with different info and personal info added } /** * Helper method for reading in the written file to check its contents. * @param writtenFilename to read * @return an ArrayList of the lines from the written file */ private ArrayList readWrittenFile(String writtenFilename) { ArrayList expectedLines = new ArrayList(); try { BufferedReader file = new BufferedReader(new FileReader(writtenFilename)); String line = file.readLine(); while (line !=null) { expectedLines.add(line); line = file.readLine(); } file.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } return expectedLines; } }
MyFileWriterTest.java import static org.junit.Assert.assertEquals; import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import org.junit.jupiter.api.BeforeEach; import org.junit.jupiter.api.Test; public class MyFileWriterTest { MyFileWriter myFileWriter1; MyFileWriter myFileWriter2; MyFileWriter myFileWriter3; MyFileWriter myFileWriter4; @BeforeEach void setUp() { this.myFileWriter1 =new MyFileWriter("test1_fw.txt"); // file with multiple words per line this.myFileWriter2 =new MyFileWriter("test2_fw.txt"); // similar to info.txt file this.myFileWriter3 =new MyFileWriter("test3_fw.txt"); // similar to info.txt file but with personal info added this.myFileWriter4 =new MyFileWriter("test4_fw.txt"); // similar to info.txt file but with different info and personal info added } @Test publicvoid testWriteToFile() { ArrayList actualLines = new ArrayList(); // Test file with multiple words per line actualLines.add("hello world"); actualLines.add("Course Name and ID"); actualLines.add("The quick brown fox jumps over the lazy dog."); // Write to file myFileWriter1.writeToFile(actualLines); // Read the written file to test its contents ArrayList expectedLines = readWrittenFile("test1_fw.txt"); assertEquals(expectedLines, actualLines); // Test original info.txt file actualLines.removeAll(actualLines); actualLines.add("Course: MCIT_590"); actualLines.add("CourseID: 590"); actualLines.add("StudentID: 101"); // Write to file myFileWriter2.writeToFile(actualLines); // Read the written file to test its contents expectedLines = readWrittenFile("test2_fw.txt"); assertEquals(expectedLines, actualLines); // TODO write at least 2 additional test cases using different MyFileWriters // Recommended: A test similar to info.txt file but with personal info added // Recommended: A test similar to info.txt file but with different info and personal info added } /** * Helper method for reading in the written file to check its contents. * @param writtenFilename to read * @return an ArrayList of the lines from the written file */ private ArrayList readWrittenFile(String writtenFilename) { ArrayList expectedLines = new ArrayList(); try { BufferedReader file = new BufferedReader(new FileReader(writtenFilename)); String line = file.readLine(); while (line !=null) { expectedLines.add(line); line = file.readLine(); } file.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } return expectedLines; } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
MyFileWriterTest.java
import static org.junit.Assert.assertEquals;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
public class MyFileWriterTest {
MyFileWriter myFileWriter1;
MyFileWriter myFileWriter2;
MyFileWriter myFileWriter3;
MyFileWriter myFileWriter4;
@BeforeEach
void setUp() {
this.myFileWriter1 =new MyFileWriter("test1_fw.txt"); // file with multiple words per line
this.myFileWriter2 =new MyFileWriter("test2_fw.txt"); // similar to info.txt file
this.myFileWriter3 =new MyFileWriter("test3_fw.txt"); // similar to info.txt file but with personal info added
this.myFileWriter4 =new MyFileWriter("test4_fw.txt"); // similar to info.txt file but with different info and personal info added
}
@Test
publicvoid testWriteToFile() {
ArrayList<String> actualLines = new ArrayList<String>();
// Test file with multiple words per line
actualLines.add("hello world");
actualLines.add("Course Name and ID");
actualLines.add("The quick brown fox jumps over the lazy dog.");
// Write to file
myFileWriter1.writeToFile(actualLines);
// Read the written file to test its contents
ArrayList<String> expectedLines = readWrittenFile("test1_fw.txt");
assertEquals(expectedLines, actualLines);
// Test original info.txt file
actualLines.removeAll(actualLines);
actualLines.add("Course: MCIT_590");
actualLines.add("CourseID: 590");
actualLines.add("StudentID: 101");
// Write to file
myFileWriter2.writeToFile(actualLines);
// Read the written file to test its contents
expectedLines = readWrittenFile("test2_fw.txt");
assertEquals(expectedLines, actualLines);
// TODO write at least 2 additional test cases using different MyFileWriters
// Recommended: A test similar to info.txt file but with personal info added
// Recommended: A test similar to info.txt file but with different info and personal info added
}
/**
* Helper method for reading in the written file to check its contents.
* @param writtenFilename to read
* @return an ArrayList of the lines from the written file
*/
private ArrayList<String> readWrittenFile(String writtenFilename) {
ArrayList<String> expectedLines = new ArrayList<String>();
try {
BufferedReader file = new BufferedReader(new FileReader(writtenFilename));
String line = file.readLine();
while (line !=null) {
expectedLines.add(line);
line = file.readLine();
}
file.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
catch (IOException e) {
e.printStackTrace();
}
return expectedLines;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 5 images

Recommended textbooks for you
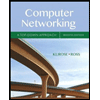
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
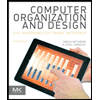
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
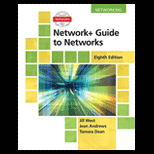
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
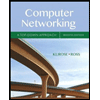
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
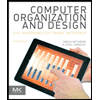
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
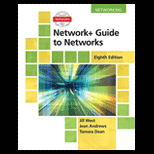
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
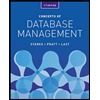
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
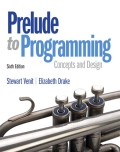
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
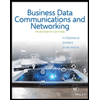
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY