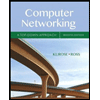
import java.util.*;
public class MyLinkedListQueue
{
class Node
{
public Object data;
public Node next;
private Node first;
public MyLinkedListQueue()
{
first = null;
}
public Object peek()
{
if(first==null)
{
throw new NoSuchElementException();
}
return first.data;
}
public void enqueue(Object element)
{
Node newNode = new newNode();
newNode.data = element;
newNode.next = first;
first = newNode;
}
public boolean isEmpty()
{
return(first==null);
}
public int size()
{
int count = 0;
Node p = first;
while(p ==! null)
{
count++;
p = p.next;
}
return count;
}
}
}
Hello, I am getting an error message when I execute this program. This is what I get:
MyLinkedListQueue.java:11: error: invalid method declaration; return type required
public MyLinkedListQueue()
^
1 error
I also need to have a dequeue method in my program. It is very similar to the enqueue method.
I'll appreciate the help, Thanks!

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- import java.util.LinkedList; public class IntTree { private Node root; private static class Node { public int key; public Node left, right; public Node(int key) { this.key = key; } } public void printInOrder() { printInOrder(root); } private void printInOrder(Node n) { if (n == null) return; printInOrder(n.left); System.out.println(n.key); printInOrder(n.right); } /* * Returns true if this tree and that tree "look the same." (i.e. They have the * same keys in the same locations in the tree.) Note that just having the same * keys is NOT enough. They must also be in the same positions in the tree. */ public boolean treeEquals(IntTree that) { // TODO throw new RuntimeException("Not implemented"); } • You are not allowed to use any kind of loop in your solutions. • You may not modify the Node class in any way• You may not modify the function…arrow_forwardpackage hw5; public class LinkedIntSet {private static class Node {private int data;private Node next; public Node(int data, Node next) {this.data = data;this.next = next;}} private Node first; // Always points to the first node of the list.// THE LIST IS ALWAYS IN SORTED ORDER!private int size; // Always equal to the number of elements in the set. /*** Construts an empty set.*/public LinkedIntSet() {throw new RuntimeException("Not implemented");} /*** Returns the number of elements in the set.* * @return the number of elements in the set.*/public int size() {throw new RuntimeException("Not implemented");} /*** Tests if the set contains a number* * @param i the number to check* @return <code>true</code> if the number is in the set and <code>false</code>* otherwise.*/public boolean contains(int i) {throw new RuntimeException("Not implemented");} /*** Adds <code>element</code> to this set if it is not already present and* returns…arrow_forwardTree Define a class called TreeNode containing three data fields: element, left and right. The element is a generic type. Create constructors, setters and getters as appropriate. Define a class called BinaryTree containing two data fields: root and numberElement. Create constructors, setters and getters as appropriate. Define a method balanceCheck to check if a tree is balanced. A tree being balanced means that the balance factor is -1, 0, or 1.arrow_forward
- Can someone explain it to me.arrow_forwardComplete public class Solution { public static LinkedListNode<Integer> findPrevNode(LinkedListNode<Integer> head, int count) { for (int i=0;i<count-1;i++) { head=head.next; } return head; } public static LinkedListNode<Integer> swapNodes(LinkedListNode<Integer> head, int i, int j) { //Your code goes here if (head==null) { return head; } else if (j==0 || (i-j==-1)) { int temp=i; i=j; j=temp; } LinkedListNode<Integer> swap1=null,swap2=null,p1=null,n1=null,p2=null,n2=null; if (i==0 && i-j==1) { swap1=head; swap2=head.next; swap1=swap1.next; head=swap2; swap2.next=swap1; } else if(i-j==1) { p1=findPrevNode(head,j); swap1=p1.next; swap2=swap1.next; n2=swap2.next; //System.out.println(p1.data); //System.out.println(swap1.data);…arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forward
- 1 Assume some Node class with info & link fields. Complete this method in class List that returns a reference to the node containing the data item in the argument find This, Assume that find this is in the list public class List { protected Node head; protected int size; fublic Public Node find (char find This)arrow_forwardImplement LeafNode and InteriorNode classes for the expression tree as discussed on this page Use this template: Please don't change any function namesAdd any methods if necesssaryTODO: Remove the pass statements and implement the methods. '''class LeafNode:def __init__(self, data):self.data = datadef postfix(self):return str(self)def __str__(self):return str(self.data)def prefix(self):passdef infix(self):passdef value(self):return self.dataclass InteriorNode:def __init__(self, op, left_op, right_op):self.op = opself.left_op = left_opself.right_op = right_opdef postfix(self):return self.left_op.postfix() + " " + self.right_op.postfix() + " " + self.opdef prefix(self):passdef infix(self):passdef value(self):passif __name__ == "__main__":# TODO: (Optional) your test code here.a = LeafNode(4)b = InteriorNode('+', LeafNode(2), LeafNode(3))c = InteriorNode('*', a, b)c = InteriorNode('-', c, b)arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forward
- Redesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forwardPLEASE HELP, PYTHON THANK YOU def add_vertex(self, vertex): if vertex not in self.__graph_dict: self.__graph_dict[vertex] = [] def add_edge(self, edge): edge = set(edge) (vertex1, vertex2) = tuple(edge) ################# # Problem 4: # Check to see if vertex1 is in the current graph dictionary ################## #DELETE AND PUT IN THE IF STATEMENTS self.__graph_dict[vertex1].append(vertex2) else: self.__graph_dict[vertex1] = [vertex2] def __generate_edges(self): edges = [] ################# # Problem 5: # Loop through all of the data in the graph dictionary and use the variable vertex for the loop'ed data ################## #DELETE AND PUT IN THE LOOP STATEMENTS for neighbour in self.__graph_dict[vertex]: if {neighbour, vertex} not in edges: edges.append({vertex, neighbour}) return edges…arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
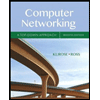
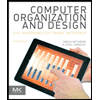
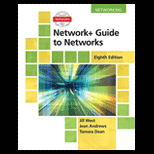
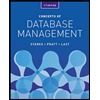
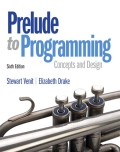
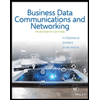