ncorporate CSTRING COMPARE strcmp, Incoporate a word frequencies , i have already done most code incorporate these details into the code below. #include #include struct node{ int data; struct node *next; }; struct node *head, *tail = NULL; void addNode(int data) { struct node *newNode = (struct node*)malloc(sizeof(struct node)); newNode->data = data; newNode->next = NULL; if(head == NULL) { head = newNode; tail = newNode; } else { tail->next = newNode; tail = newNode; } } void removeDuplicate() { struct node *current = head, *index = NULL, *temp = NULL; if(head == NULL) { return; } else { while(current != NULL){ temp = current; index = current->next; while(index != NULL) { if(current->data == index->data) { temp->next = index->next; } else { temp = index; } index = index->next; } current = current->next; } } } void display() { struct node *current = head; if(head == NULL) { printf("List is empty \n"); return; } while(current != NULL) { printf("%d ", current->data); current = current->next; } printf("\n"); } struct node* findMiddle(struct node* b, struct node* e) { if(b == NULL) return NULL; struct node* slow = b; struct node* fast = b->next; while(fast != e) { fast=fast->next; if(fast != e) { slow = slow->next; fast = fast->next; } } return slow; }; struct node* binarysearch(int searchItem) { struct node* beg = head; struct node* end = NULL; do { struct node* middle = findMiddle(beg, end); if(middle == NULL) return middle; else if(middle->data == searchItem) return middle; else if(middle->data < searchItem) beg = middle->next; else end = middle; }while(end==NULL || end != beg); return NULL; } int main() { addNode(1); addNode(2); addNode(3); addNode(2); addNode(2); addNode(4); addNode(1); printf("Originals list: \n"); display(); removeDuplicate(); printf("List after removing duplicates: \n"); display(); printf("Search for 3 in the linked list:\n"); struct node* n = binarysearch(3); if(n==NULL) { printf("Item not Found\n"); } else { printf("Item Found\n"); printf("%d\n",n->data); } printf("Search for 7 in the linked list:\n"); n = binarysearch(7); if(n==NULL) { printf("Item not Found\n"); } else { printf("Item Found\n"); printf("%d",n->data); } return 0; }
ncorporate CSTRING COMPARE strcmp, Incoporate a word frequencies , i have already done most code incorporate these details into the code below.
#include <stdio.h>
#include <stdlib.h>
struct node{
int data;
struct node *next;
};
struct node *head, *tail = NULL;
void addNode(int data) {
struct node *newNode = (struct node*)malloc(sizeof(struct node));
newNode->data = data;
newNode->next = NULL;
if(head == NULL) {
head = newNode;
tail = newNode;
}
else {
tail->next = newNode;
tail = newNode;
}
}
void removeDuplicate() {
struct node *current = head, *index = NULL, *temp = NULL;
if(head == NULL) {
return;
}
else {
while(current != NULL){
temp = current;
index = current->next;
while(index != NULL) {
if(current->data == index->data) {
temp->next = index->next;
}
else {
temp = index;
}
index = index->next;
}
current = current->next;
}
}
}
void display() {
struct node *current = head;
if(head == NULL) {
printf("List is empty \n");
return;
}
while(current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
struct node* findMiddle(struct node* b, struct node* e)
{
if(b == NULL)
return NULL;
struct node* slow = b;
struct node* fast = b->next;
while(fast != e)
{
fast=fast->next;
if(fast != e)
{
slow = slow->next;
fast = fast->next;
}
}
return slow;
};
struct node* binarysearch(int searchItem)
{
struct node* beg = head;
struct node* end = NULL;
do
{
struct node* middle = findMiddle(beg, end);
if(middle == NULL)
return middle;
else if(middle->data == searchItem)
return middle;
else if(middle->data < searchItem)
beg = middle->next;
else
end = middle;
}while(end==NULL || end != beg);
return NULL;
}
int main()
{
addNode(1);
addNode(2);
addNode(3);
addNode(2);
addNode(2);
addNode(4);
addNode(1);
printf("Originals list: \n");
display();
removeDuplicate();
printf("List after removing duplicates: \n");
display();
printf("Search for 3 in the linked list:\n");
struct node* n = binarysearch(3);
if(n==NULL)
{
printf("Item not Found\n");
}
else
{
printf("Item Found\n");
printf("%d\n",n->data);
}
printf("Search for 7 in the linked list:\n");
n = binarysearch(7);
if(n==NULL)
{
printf("Item not Found\n");
}
else
{
printf("Item Found\n");
printf("%d",n->data);
}
return 0;
}

Step by step
Solved in 3 steps with 1 images

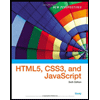
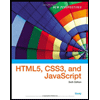