/Can you explain how this code work or the meaning of this code?
//Can you explain how this code work or the meaning of this code?
#include <iostream>
using namespace std;
struct Node {
int data;
struct Node *next;
};
struct Node* head = NULL;
struct Node* rhead = NULL;
int count1;
//insert at front for reverselist
void insertfront(int data)
{
Node* new_node = (Node*) malloc(sizeof(Node));
new_node->data = data;
new_node->next = rhead;
rhead=new_node;
}
//insert at end
void insert(int new_data)
{
Node* ptr;
ptr = head;
Node* new_node = (Node*) malloc(sizeof(Node));
new_node->data = new_data;
new_node->next = NULL;
if (head == NULL)
{
head = new_node;
}
else
{
while (ptr->next!= NULL)
{
ptr = ptr->next;
}
ptr->next=new_node;
}
count1++;
}
//display list
void display() {
struct Node* ptr;
ptr = head;
if(head==NULL)
{
cout<<"Sorry the list is empty";
return;
}
while (ptr != NULL) {
cout<< ptr->data <<" ";
ptr = ptr->next;
}
cout<<endl;
}
//delete data
void deletedata(int data)
{
Node* ptr,*ptrpre;
ptr = head;
if (head == NULL)
{
cout<<"List is empty";
}
else
{
while (ptr->next!= NULL && ptr->data !=data)
{
ptrpre=ptr;
ptr = ptr->next;
}
if(ptr->next==NULL)
{
cout<<"Sorry we cannot remove the data as it is not in the list"<<endl;
return;
}
else
{
ptrpre->next=ptr->next;
cout<<"*Display List *"<<endl;
display();
}
count1--;
}
}
//reverse list
void reverselist()
{
Node *ptr,*ptr1;
ptr=head;
while (ptr != NULL) {
insertfront(ptr->data);
ptr = ptr->next;
}
ptr1=rhead;
cout<<"Reversed list"<<endl;
while (ptr1 != NULL) {
cout<<ptr1->data<<" ";
ptr1 = ptr1->next;
}
cout<<endl;
}
//search data
void searchdata(int data)
{
Node* ptr,*ptrpre;
ptr = head;
if (head == NULL)
{
cout<<"List is empty";
}
else
{
while (ptr->next!= NULL && ptr->data !=data)
{
ptrpre=ptr;
ptr = ptr->next;
}
if(ptr->next==NULL)
{
cout<<"No, the data is not found!"<<endl;
return;
}
else
{
cout<<"Yes, the data is found!"<<endl;
return;
}
}
}
//swap for sorting
void swap(struct Node *a, struct Node *b)
{
int temp;
temp= a->data;
a->data = b->data;
b->data = temp;
}
//function for sort
void datasort()
{
int swapped, i;
struct Node *ptr2;
struct Node *lptr = NULL;
/* Checking for empty list */
if (head == NULL)
return;
else{
do
{
swapped = 0;
ptr2 = head;
while (ptr2->next != lptr)
{
if (ptr2->data> ptr2->next->data)
{
swap(ptr2, ptr2->next);
swapped = 1;
}
ptr2 = ptr2->next;
}
lptr = ptr2;
} while (swapped);
}
}
//delete list
void deleteList()
{
cout<<"*Delete list*"<<endl;
head=NULL;
}
int main() {
int num,rnum,snum;
for(int i=0;i<5;i++)
{
cout<<"Please enter a new value to be inserted :";
cin>>num;
insert(num);
}
cout<<"*Display List *"<<endl;
display();
cout<<"Please enter a data to be removed : ";
cin>>rnum;
deletedata(rnum);
cout<<"Please enter a data to be removed : ";
cin>>rnum;
deletedata(rnum);
cout<<"Please enter a data that you want to find :";
cin>>snum;
searchdata(snum);
reverselist();
datasort();
cout<<"Sorted List"<<endl;
display();
rhead=NULL;
reverselist();
deleteList();
cout<<"*Display List *"<<endl;
display();
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

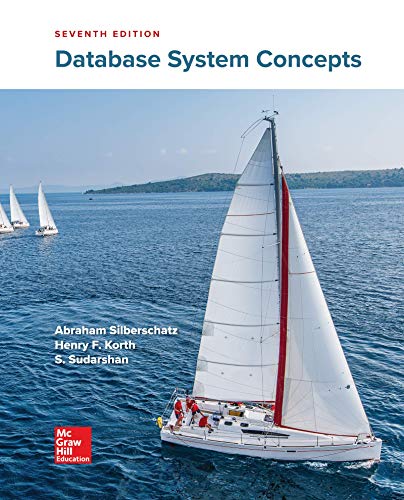
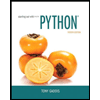
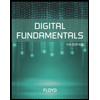
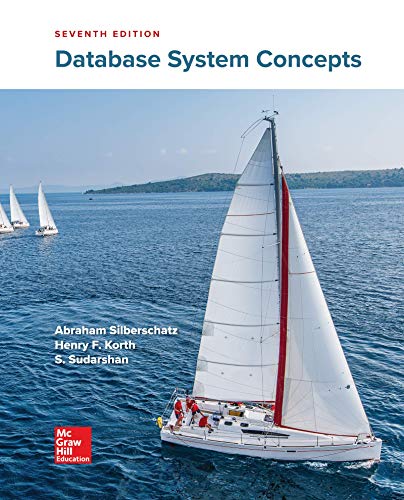
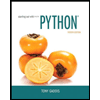
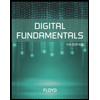
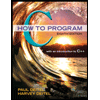
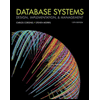
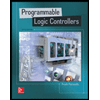