need help completing the code in java ArrayList implementation of a Set data structure. Recall from math that sets are collections of objects where: order does not matter elements cannot repeat For example, a set may look as follows: A = {3, 5, a, d}. In this case: The set name is A The set has 4 elements (3, 5, a, and d) The order does not matter - for example, A could have been represented as: {a, 5, d, 3} The starter code for this data structure is: public class Set { private ArrayList elements; public Set () { elements = new ArrayList(); } public String toString () { //Will display the set in the format {e1, e2, e3,...,en} where e1 through en are the elements in the set boolean printComma = false; StringBuilder sb = new StringBuilder(); sb.append("{"); for (int i = 0; i < elements.size(); i++) { if (i != 0) { sb.append(", "); } sb.append(elements.get(i)); } sb.append("}"); return sb.toString();
need help completing the code in java
ArrayList implementation of a Set data structure. Recall from math that sets are collections of objects where:
order does not matter
elements cannot repeat
For example, a set may look as follows: A = {3, 5, a, d}. In this case:
The set name is A
The set has 4 elements (3, 5, a, and d)
The order does not matter - for example, A could have been represented as: {a, 5, d, 3}
The starter code for this data structure is:
public class Set {
private ArrayList<Integer> elements;
public Set () {
elements = new ArrayList<Integer>();
}
public String toString () {
//Will display the set in the format {e1, e2, e3,...,en} where e1 through en are the elements in the set
boolean printComma = false;
StringBuilder sb = new StringBuilder();
sb.append("{");
for (int i = 0; i < elements.size(); i++) {
if (i != 0) {
sb.append(", ");
}
sb.append(elements.get(i));
}
sb.append("}");
return sb.toString();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

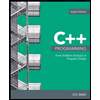
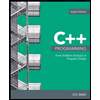