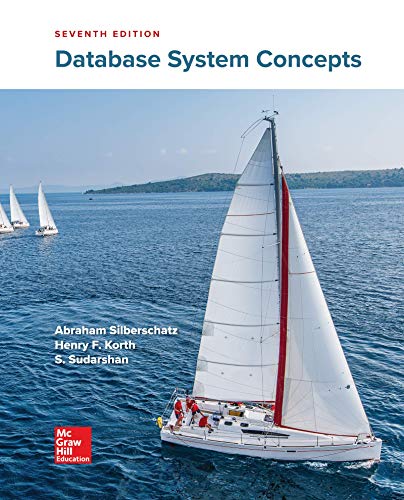
Concept explainers
In this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not impact the overall functionality of the set class. • A minimum size (initial capacity) may be specified for the ArrayList object. • When elements are added, the ensureCapacity() method may be called to ensure that adding a new element can proceed. • The trimToSize() method can be used to maintain a constant capacity equal to the number of elements in the set. Set Class Methods There are many methods that one would expect to be supported in a set class. This section will describe the interface of the set class. Unless specified, you will have to implement all of the described methods. Constructors • Set() o Description: constructs a set by allocating an ArrayList. • Set(int size) o Parameters: size - the desired size of the set. o Description: constructs a set with an initial capacity of size. • Set(int low, int high) o Parameters: low – an integer specifying the start value of a range of values. high – an integer specifying the end value of a range of values. o Description: constructs a set of Integer objects containing all the inclusive values from the range low…high. The default size of the set must accommodate this mode of construction. Addition • boolean add(Integer o) o Parameters: o – element to be added to this set. o Description: if o is not in this set, o is added to this set. o Returns: TRUE if the element is successfully added to this set. FALSE if the element is not added to this set. • int add(Integer[] s) o Parameters: s – array of elements to be added to this set. o Description: adds all elements of s to this set. o Returns: the number of elements successfully added to this set. Removal • Integer remove(Integer o) o Parameters: o – element to be deleted from this set. o Description: if o is in this set, the element is deleted. o Returns: the object that will be removed from this set. If the element is not contained in this set, null is returned. • int remove(Set s) o Parameters: s – set of elements to be deleted from this set. o Description: deletes all elements of s from this set. o Returns: the number of elements successfully deleted from this set. Miscellaneous • boolean contains(Integer o) o Parameters: o – the element to be searched for in this set. o Description: determines whether the given element is in this set. o Returns: TRUE or FALSE whether o is in this set. • void clear() o Description: Removes all the elements from this set. • boolean isEmpty() o Description: determines whether this set contains any elements.
in java and can you a lot of comments in it thank you
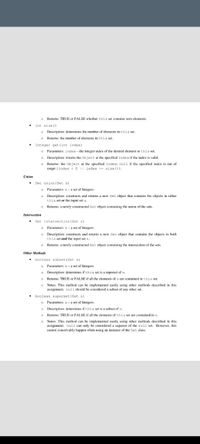
![Supplied Methods
Besides the interface specification described above, there are other methods that have been provided; feel
free to use them as required in your implementation and testing. Do not modify these methods.
• String toString ()
o Description: provides a means of viewing the values contained within the Set object.
o Returns: a String representation of this set.
o Notes: It is important that this method remain unmodified since it will be used for
evaluation purposes. It has been provided for debugging purposes.
boolean equals (Object o)
o Parameters: s- a set of values to compare against this set.
o Description: determines whether the content of o equals this set.
o Returns: TRUE or FALSE whether o is an instance of class Set and contains all
elements of this set.
o Notes: It is important that this method remain unmodified since it will be used for
evaluation purposes. It has been provided for debugging purposes.
Testing
public static void main (String args[])
o Parameters: args - unused in this implementation.
o Description: this is a method that will be part of a test class Set Tester.
o Notes: A complete testing class (SetTester) used for grading has been provided to
expedite development. This method can be modified as desired; you will not have to
submit this file.](https://content.bartleby.com/qna-images/question/36e2a10a-56c0-4adb-bccf-fb7de3247667/ccd9f583-be3e-47ee-b22c-ffdbea700cfe/ut8prtv_thumbnail.jpeg)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Cs10 Quiz 1 6 / 7 100% + 26. Given the definition of the classes Node and List above but with this new List public member function named mystery added, draw the memory diagram after all of the shown code in the main function executes (but before the main function ends). Be careful, there may be dangling pointers and/or memory leaks after calling the mystery function. Be sure to show these in your diagram. Circle ALL memory leaks. You may put ?? in a dangling pointer variable or just leave the arrow dangling in your drawing. Be sure to draw pointers in the same manner as I showed in lecture (arrow starts inside variable and ends at memory location it points to). void List::mystery (int val) { if (head == nullptr) { head = new Node (val); tail = head; } else if (head->next == nullptr) { head->next = new Node (val); } else ( Node *nl = new Node (val); int main () { List list; list.mystery (2); list.mystery (3) ; list.mystery (5); // Draw what memory // looks like now // (Note: the main…arrow_forwardCan you please help me with this code because i am struggling on how to do this, this code has to be in C code.question that i need help with:Priority with round-robin schedules tasks in order of priority and uses round-robin scheduling for tasks with equal priority. There will be multiple queues in the system each representing one priority class. For ease of implementation, you need total 1 through max_priority numbers of queues. You should start scheduling job out of the max priority queue and serve the members of the queue following RR. The schedule of tasks has the form [task name] [priority] [CPU burst], with the following example format: T1, 4, 20 T2, 2, 25 T3, 3, 25 T4, 3, 15 T5, 10, 10 The output should look like this: Running task = [P1] [4] [5] for 5 units. Task P1 finished. Running task = [P5] [3] [4] for 4 units. Task P5 finished. Running task = [P4] [2] [7] for 1 units. Task P4 exhausted its Quantum hence will be Rescheduled. Running task = [P3] [2] [1] for 1 units. Task…arrow_forwardProvide an explanation for the primary distinction that exists here between collection and an arraylist.arrow_forward
- I have written a class that has the characteristics of the data structure Set and within it house an array that stores 10 integers. I would like a function written in C++ that will perform a set union comparison between two class set instances and only print out one of each element from both of them, (no duplicates). Thanks!arrow_forwardIn this project, you will implement a Set class that represents a general collection of values. For this assignment, a set is generally defined as a list of values that are sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. (in java)Requirements among all implementations there are some requirements that all implementations must maintain. • Your implementation should always reflect the definition of a set. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections. the sort that automatically sorts a list may not be used. Instead, when a successful addition of an element to the Set is done, you can ensure that the elements inside the ArrayList<Integer>…arrow_forwardHi, I am a bit lost on how to do this coding assignment. It would be great if someone could help me fill in the MyStack.java file with the given information. All the information is in the pictures. Thank you!arrow_forward
- implement a c# program that tests our implementation of the CSet class by creating two sets, performing a union of the two sets, an intersection of the two sets, finding the subset of the two sets, and the difference of the two sets.arrow_forwardFor the Assignment 5 (Part 2), Routes v.2 at the end of this module you will create a Route class that has a member a "bag" of Leg objects. The bag is to use a vector of pointers, but since all the objects in the bag are to be Leg s, it's okay to use Leg* instead of void* in the bag's declaration. You wrote its first constructor in a previous exercise. Now write a second one. (You may refer to the Assignment page to refer to the completed class declarations of Leg and Route classes). Assume that class Leg has data member C strings const char* const beginCity; and const char* const endCity; that are available to Route by a friend relationship. Write a constructor function for a Route class that takes exactly two parameters: a constant Route object reference to an already-existing Route , and a constant Leg object reference to be appended to the end of that Route to form the new Route . Here's the algorithm: Copy the first parameter's bag into the host object's bag. If the endCity of…arrow_forwardThis chapter described the array implementation of queues that use a special array slot, called the reserved slot, to distinguish between an empty and a full queue. Write the definition of the class and the definitions of the function members of this queue design. Also, write a test program to test various operations on a queue.arrow_forward
- In this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not…arrow_forwardSpecify and implement an iterator method called sortedElements for IntSet. The iterator method sortedElements returns an iterator that will produce each element of the intset in ascending order. The intset cannot be mutated when the iterator is in use. Be sure to specify the iterator method before implementation. Do not forget to give the rep invariant and abstraction function for your implementation. Note: IntSet.java has been provided. You can directly add in the code the specification, the implementation of the iterator method sortedElements, and the inner iterator class. There is a segment of simple testing code for sortedElements in main() close to the end in the provided IntSet.java. The program will not compile until you have properly implemented sortedElements. find the provided code below: https://drive.google.com/open?id=1hANO8kVigGXwFLLJDJQLE5joM1BBeCCnarrow_forwardMovie Recommendations via Item-Item Collaborative Filtering. You are providedwith real-data (Movie-Lens dataset) of user ratings for different movies. There is a readmefile that describes the data format. In this project, you will implement the item-item collab-orative filtering algorithm that we discussed in the class. The high-level steps are as follows: a) Construct the profile of each item (i.e., movie). At the minimum, you should use theratings given by each user for a given item (i.e., movie). Optionally, you can use other in-formation (e.g., genre information for each movie and tag information given by user for eachmovie) creatively. If you use this additional information, you should explain your method-ology in the submitted report. b) Compute similarity score for all item-item (i.e., movie-movie) pairs. You will employ thecentered cosine similarity metric that we discussed in class. c) Compute the neighborhood set Nfor each item (i.e. movie). You will select the moviesthat…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
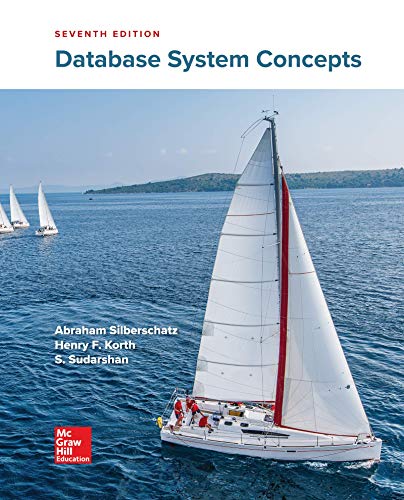
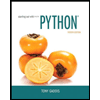
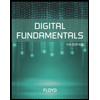
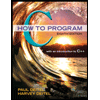
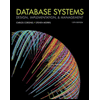
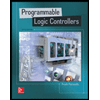