Need help with this python question. You can't use break, str.endswith, list.index, keywords like: await, as, assert, class, except, lambda, and built in functions like: any, all, breakpoint, callable. ---------You also can't use Sorted---------- Create a recursive function def ab_equal(n, k, current): Print out all of the strings of a's and b's of length n so that the number of a's and b's are equal. For n = 2, there's ab and ba. For n = 3 there are no strings since they'd have to have 2 a's and 1 b, or 2 b's a 1 a so not equal. For n = 4, there will be 6 of these strings, and for n = 5, zero again. Hint: use k to track the difference between a's and b's. So for instance if your current is aaabb then k should be equal to either 1 or -1 (your choice depending). When you call the function, you should call it from your main or testing function with the length in the n parameter, 0 should be put into the k parameter, and then an empty string will be passed in for current. Sample Output linux5[109]% python3 ab_equality.py What length do you want to run? 4 aabb abab abba baab baba bbaa linux5[110]% python3 ab_equality.py What length do you want to run? 6 aaabbb aababb aabbab aabbba abaabb ababab ababba abbaab abbaba abbbaa baaabb baabab baabba babaab bababa babbaa bbaaab bbaaba bbabaa bbbaaa Coding Standards Constants above your function definitions, outside of the "if __name__ == '__main__':" block. A magic value is a string which is outside of a print or input statement, but is used to check a variable, so for instance: print(first_creature_name, 'has died in the fight. ') does not involve magic values. However, if my_string == 'EXIT': exit is a magic value since it's being used to compare against variables within your code, so it should be: EXIT_STRING = 'EXIT' ... if my_string == EXIT_STRING: A number is a magic value when it is not 0, 1, and if it is not 2 being used to test parity (even/odd). A number is magic if it is a position in an array, like my_array[23], where we know that at the 23rd position, there is some special data. Instead it should be USERNAME_INDEX = 23 my_array[USERNAME_INDEX] Constants in mathematical formulas can either be made into official constants or kept in a formula. Previously checked coding standards involving: snake_case_variable_names CAPITAL_SNAKE_CASE_CONSTANT_NAMES Use of whitespace (2 before and after a function, 1 for readability.)
Need help with this python question. You can't use break, str.endswith, list.index, keywords like: await, as, assert, class, except, lambda, and built in functions like: any, all, breakpoint, callable. ---------You also can't use Sorted---------- Create a recursive function def ab_equal(n, k, current): Print out all of the strings of a's and b's of length n so that the number of a's and b's are equal. For n = 2, there's ab and ba. For n = 3 there are no strings since they'd have to have 2 a's and 1 b, or 2 b's a 1 a so not equal. For n = 4, there will be 6 of these strings, and for n = 5, zero again. Hint: use k to track the difference between a's and b's. So for instance if your current is aaabb then k should be equal to either 1 or -1 (your choice depending). When you call the function, you should call it from your main or testing function with the length in the n parameter, 0 should be put into the k parameter, and then an empty string will be passed in for current. Sample Output linux5[109]% python3 ab_equality.py What length do you want to run? 4 aabb abab abba baab baba bbaa linux5[110]% python3 ab_equality.py What length do you want to run? 6 aaabbb aababb aabbab aabbba abaabb ababab ababba abbaab abbaba abbbaa baaabb baabab baabba babaab bababa babbaa bbaaab bbaaba bbabaa bbbaaa Coding Standards Constants above your function definitions, outside of the "if __name__ == '__main__':" block. A magic value is a string which is outside of a print or input statement, but is used to check a variable, so for instance: print(first_creature_name, 'has died in the fight. ') does not involve magic values. However, if my_string == 'EXIT': exit is a magic value since it's being used to compare against variables within your code, so it should be: EXIT_STRING = 'EXIT' ... if my_string == EXIT_STRING: A number is a magic value when it is not 0, 1, and if it is not 2 being used to test parity (even/odd). A number is magic if it is a position in an array, like my_array[23], where we know that at the 23rd position, there is some special data. Instead it should be USERNAME_INDEX = 23 my_array[USERNAME_INDEX] Constants in mathematical formulas can either be made into official constants or kept in a formula. Previously checked coding standards involving: snake_case_variable_names CAPITAL_SNAKE_CASE_CONSTANT_NAMES Use of whitespace (2 before and after a function, 1 for readability.)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Need help with this python question. You can't use break, str.endswith, list.index, keywords like: await, as, assert, class, except, lambda, and built in functions like: any, all, breakpoint, callable.
---------You also can't use Sorted----------
Create a recursive function
def ab_equal(n, k, current):
Print out all of the strings of a's and b's of length n so that the number of a's and b's are equal.
For n = 2, there's ab and ba. For n = 3 there are no strings since they'd have to have 2 a's and 1 b, or 2 b's a 1 a so not equal. For n = 4, there will be 6 of these strings, and for n = 5, zero again.
Hint: use k to track the difference between a's and b's. So for instance if your current is aaabb then k should be equal to either 1 or -1 (your choice depending).
When you call the function, you should call it from your main or testing function with the length in the n parameter, 0 should be put into the k parameter, and then an empty string will be passed in for current.
Sample Output
linux5[109]% python3 ab_equality.py What length do you want to run? 4 aabb abab abba baab baba bbaa linux5[110]% python3 ab_equality.py What length do you want to run? 6 aaabbb aababb aabbab aabbba abaabb ababab ababba abbaab abbaba abbbaa baaabb baabab baabba babaab bababa babbaa bbaaab bbaaba bbabaa bbbaaa |
Coding Standards
- Constants above your function definitions, outside of the "if __name__ == '__main__':" block.
- A magic value is a string which is outside of a print or input statement, but is used to check a variable, so for instance:
- print(first_creature_name, 'has died in the fight. ') does not involve magic values.
- However, if my_string == 'EXIT': exit is a magic value since it's being used to compare against variables within your code, so it should be:
EXIT_STRING = 'EXIT'
- A magic value is a string which is outside of a print or input statement, but is used to check a variable, so for instance:
...
if my_string == EXIT_STRING:
- A number is a magic value when it is not 0, 1, and if it is not 2 being used to test parity (even/odd).
- A number is magic if it is a position in an array, like my_array[23], where we know that at the 23rd position, there is some special data. Instead it should be
USERNAME_INDEX = 23
my_array[USERNAME_INDEX]
- Constants in mathematical formulas can either be made into official constants or kept in a formula.
- Previously checked coding standards involving:
- snake_case_variable_names
- CAPITAL_SNAKE_CASE_CONSTANT_NAMES
- Use of whitespace (2 before and after a function, 1 for readability.)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
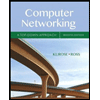
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
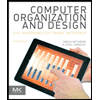
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
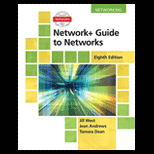
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
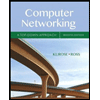
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
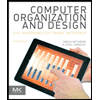
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
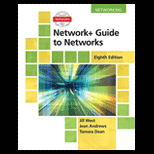
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
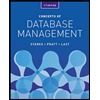
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
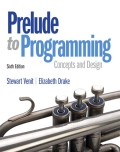
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
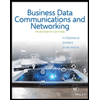
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY