python code. Instructions are given below for each function. Run function is already done. 1) def num_to_digit_rec(num, base): """ Return a list of digits for num with given base; Return an empty list [] if base < 2 or num <= 0 """ # Write your code here return [] 2) def digit_sum(num, base): """ Return the sum of all digits for a num with given base Your implementation should use num_to_digit_rec() function """ # Write your code here return 0 3) def digit_str(num, base): """ Given a number and a base, for base between [2, 36] inclusive Converts the number to its string representation using digits 0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ to represent digits 0 to 35. Return the string representation of the number Return an empty string '' if base is not between [2, 36] Your implementation should use num_to_digit_rec() function """ digits = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ" # Can not find str representations for base not in [2, 36] if base > 36 or base < 2: return "" # Calculate and return the str representation for num for the given base # Write your code here return "" 4) def digit_to_num(rep, base): """ Return -1 if base is not between [2,36] inclusive; or if the string rep contains characters not a digit for the base; Return the number represented by the string for the given base otherwise. For example digit_to_num("1001", 2) is 9; digit_to_num("ABC", 16) is 2748. """ # Check if the base is valid if base > 36 or base < 2: return -1 digits = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ" rep = rep.upper() # Check if the rep only uses proper digits # Write your code here return 0 5) def uses_only(word, letters): """ Return True if word only uses characters from letters; otherwise return False """ # Write your code here return True 6) def run(): num = int(input("Enter a num: ")) base = int(input("Enter a base: ")) print(f"Digit list is {num_to_digit_rec(num, base)}") print(f"Digit sum is {digit_sum(num, base)}") print(f"String Rep is {digit_str(num, base)}") rep = input(f"Enter a string rep of a num with base {base}: ") print(f"The number is {digit_to_num(rep, base)}")
python code. Instructions are given below for each function. Run function is already done. 1) def num_to_digit_rec(num, base): """ Return a list of digits for num with given base; Return an empty list [] if base < 2 or num <= 0 """ # Write your code here return [] 2) def digit_sum(num, base): """ Return the sum of all digits for a num with given base Your implementation should use num_to_digit_rec() function """ # Write your code here return 0 3) def digit_str(num, base): """ Given a number and a base, for base between [2, 36] inclusive Converts the number to its string representation using digits 0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ to represent digits 0 to 35. Return the string representation of the number Return an empty string '' if base is not between [2, 36] Your implementation should use num_to_digit_rec() function """ digits = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ" # Can not find str representations for base not in [2, 36] if base > 36 or base < 2: return "" # Calculate and return the str representation for num for the given base # Write your code here return "" 4) def digit_to_num(rep, base): """ Return -1 if base is not between [2,36] inclusive; or if the string rep contains characters not a digit for the base; Return the number represented by the string for the given base otherwise. For example digit_to_num("1001", 2) is 9; digit_to_num("ABC", 16) is 2748. """ # Check if the base is valid if base > 36 or base < 2: return -1 digits = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ" rep = rep.upper() # Check if the rep only uses proper digits # Write your code here return 0 5) def uses_only(word, letters): """ Return True if word only uses characters from letters; otherwise return False """ # Write your code here return True 6) def run(): num = int(input("Enter a num: ")) base = int(input("Enter a base: ")) print(f"Digit list is {num_to_digit_rec(num, base)}") print(f"Digit sum is {digit_sum(num, base)}") print(f"String Rep is {digit_str(num, base)}") rep = input(f"Enter a string rep of a num with base {base}: ") print(f"The number is {digit_to_num(rep, base)}")
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 8PE
Related questions
Question
python code. Instructions are given below for each function. Run function is already done.
1) def num_to_digit_rec(num, base):
"""
Return a list of digits for num with given base;
Return an empty list [] if base < 2 or num <= 0
"""
# Write your code here
return []
2) def digit_sum(num, base):
"""
Return the sum of all digits for a num with given base
Your implementation should use num_to_digit_rec() function
"""
# Write your code here
return 0
3) def digit_str(num, base):
"""
Given a number and a base, for base between [2, 36] inclusive
Converts the number to its string representation using digits
0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ
to represent digits 0 to 35.
Return the string representation of the number
Return an empty string '' if base is not between [2, 36]
Your implementation should use num_to_digit_rec() function
"""
digits = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ"
# Can not find str representations for base not in [2, 36]
if base > 36 or base < 2:
return ""
# Calculate and return the str representation for num for the given base
# Write your code here
return ""
4) def digit_to_num(rep, base):
"""
Return -1 if base is not between [2,36] inclusive;
or if the string rep contains characters not a digit for the base;
Return the number represented by the string for the given base otherwise.
For example digit_to_num("1001", 2) is 9; digit_to_num("ABC", 16) is 2748.
"""
# Check if the base is valid
if base > 36 or base < 2:
return -1
digits = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ"
rep = rep.upper()
# Check if the rep only uses proper digits
# Write your code here
return 0
5) def uses_only(word, letters):
"""
Return True if word only uses characters from letters;
otherwise return False
"""
# Write your code here
return True
6) def run():
num = int(input("Enter a num: "))
base = int(input("Enter a base: "))
print(f"Digit list is {num_to_digit_rec(num, base)}")
print(f"Digit sum is {digit_sum(num, base)}")
print(f"String Rep is {digit_str(num, base)}")
rep = input(f"Enter a string rep of a num with base {base}: ")
print(f"The number is {digit_to_num(rep, base)}")
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
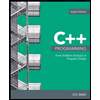
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
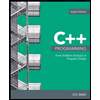
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning