The following function f uses recursion: def f(n): if n <= 1 return n else return f(n-1) + f(n-2) 5 Let n be a valid input, i.e., a natural number. Which of the following functions returns the same result but without recursion? a) def f(n): a <- 0 b <- 1 if n = 0 return a elsif n = 1 return b else for i in 1..n c <- a + b a <- b b <- c return b b) def f(n): a <- 0 i <- n while i > 0 a <- a + i + (i-1) return a c) def f(n): arr[0] <- 0 arr[1] <- 1 if n <= 1 return arr[n] else for i in 2..n arr[i] <- arr[i-1] + arr[i-2] return arr[n] d) def f(n): arr[0..n] <- [0, ..., n] if n <= 1 return arr[n] else a <- 0 for i in 0..n a <- a + arr[i] return a
The following function f uses recursion: def f(n): if n <= 1 return n else return f(n-1) + f(n-2) 5 Let n be a valid input, i.e., a natural number. Which of the following functions returns the same result but without recursion? a) def f(n): a <- 0 b <- 1 if n = 0 return a elsif n = 1 return b else for i in 1..n c <- a + b a <- b b <- c return b b) def f(n): a <- 0 i <- n while i > 0 a <- a + i + (i-1) return a c) def f(n): arr[0] <- 0 arr[1] <- 1 if n <= 1 return arr[n] else for i in 2..n arr[i] <- arr[i-1] + arr[i-2] return arr[n] d) def f(n): arr[0..n] <- [0, ..., n] if n <= 1 return arr[n] else a <- 0 for i in 0..n a <- a + arr[i] return a
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter15: Recursion
Section: Chapter Questions
Problem 1TF
Related questions
Question
The following function f uses recursion:
def f(n):
if n <= 1
return n
else
return f(n-1) + f(n-2)
5
Let n be a valid input, i.e., a natural number. Which of the following functions returns the same result but without recursion?
a) def f(n):
a <- 0
b <- 1
if n = 0
return a
elsif n = 1
return b
else
for i in 1..n
c <- a + b
a <- b
b <- c
return b
b) def f(n):
a <- 0
i <- n
while i > 0
a <- a + i + (i-1)
return a
c) def f(n):
arr[0] <- 0
arr[1] <- 1
if n <= 1
return arr[n]
else
for i in 2..n
arr[i] <- arr[i-1] + arr[i-2]
return arr[n]
d) def f(n):
arr[0..n] <- [0, ..., n]
if n <= 1
return arr[n]
else
a <- 0
for i in 0..n
a <- a + arr[i]
return a
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
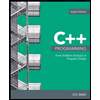
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
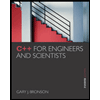
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
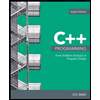
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
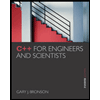
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr