Next, create class Lab14A with a main method or driver which should do the following: Create two building objects: o buildingone should be created using the default constructor o buildingTwo should be created using the overloaded (argument) constructor with the values provided: 30 Stories 30 apartments Occupancy variable set to 75% (0.75) – you may have to cast to float • You should then print out the information of buildingOne like the example shown below in the sample using the getters You should then print out the information of buildingTwo like the example shown below in the sample using the getters • Use the Setter of the occupancy percentage of buildingOne to change its value to 0% (0.0) • Use the Setter of the occupancy percentage of buildingTwo to change it to 100% (1.0) Remember, the name of the class containing the main method should be Lab14A. Please make sure that your two classes (Lab14A and BuildingBlueprint) are in the one file Please keep in mind that output of everyone should be the same and as shown below... Sample (AND ONLY) output: Year 2020: Building 1 has 10 floors, 20 apartments, and is 100% occupied. Full? true Building 2 has 30 floors, 30 apartments, and is 75% occupied. Full? false Many years pass. Year 2043: Building 1 has 10 floors, 20 apartments, and is 0% occupied. Full? false Building 2 has 30 floors, 30 apartments, and is 100% occupied. Full? true Looks like people prefer taller buildings.
Next, create class Lab14A with a main method or driver which should do the following: Create two building objects: o buildingone should be created using the default constructor o buildingTwo should be created using the overloaded (argument) constructor with the values provided: 30 Stories 30 apartments Occupancy variable set to 75% (0.75) – you may have to cast to float • You should then print out the information of buildingOne like the example shown below in the sample using the getters You should then print out the information of buildingTwo like the example shown below in the sample using the getters • Use the Setter of the occupancy percentage of buildingOne to change its value to 0% (0.0) • Use the Setter of the occupancy percentage of buildingTwo to change it to 100% (1.0) Remember, the name of the class containing the main method should be Lab14A. Please make sure that your two classes (Lab14A and BuildingBlueprint) are in the one file Please keep in mind that output of everyone should be the same and as shown below... Sample (AND ONLY) output: Year 2020: Building 1 has 10 floors, 20 apartments, and is 100% occupied. Full? true Building 2 has 30 floors, 30 apartments, and is 75% occupied. Full? false Many years pass. Year 2043: Building 1 has 10 floors, 20 apartments, and is 0% occupied. Full? false Building 2 has 30 floors, 30 apartments, and is 100% occupied. Full? true Looks like people prefer taller buildings.
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 3PE
Related questions
Question
only in c++ please!
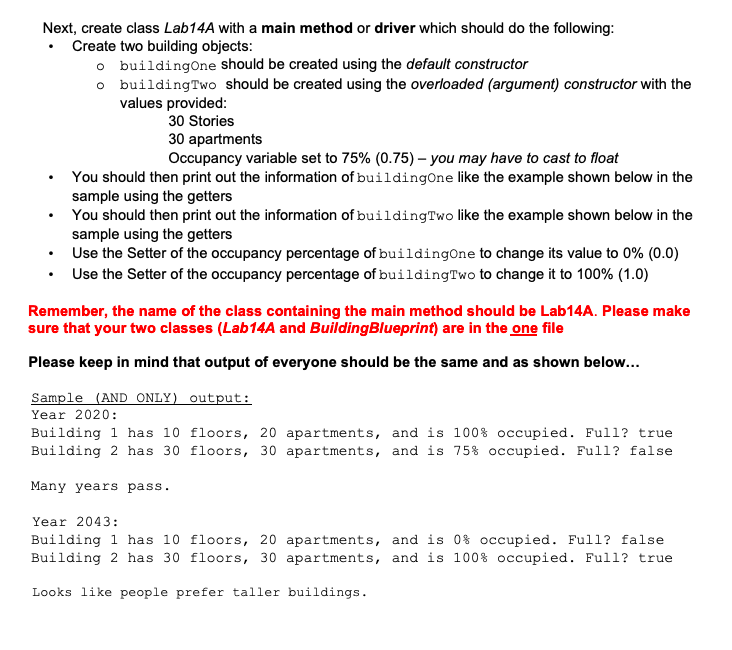
Transcribed Image Text:Next, create class Lab14A with a main method or driver which should do the following:
Create two building objects:
o buildingone should be created using the default constructor
o buildingTwo should be created using the overloaded (argument) constructor with the
values provided:
30 Stories
30 apartments
Occupancy variable set to 75% (0.75) – you may have to cast to float
You should then print out the information of building0ne like the example shown below in the
sample using the getters
You should then print out the information of buildingTwo like the example shown below in the
sample using the getters
• Use the Setter of the occupancy percentage of buildingOne to change its value to 0% (0.0)
• Use the Setter of the occupancy percentage of buildingTwo to change it to 100% (1.0)
Remember, the name of the class containing the main method should be Lab14A. Please make
sure that your two classes (Lab14A and BuildingBlueprint) are in the one file
Please keep in mind that output of everyone should be the same and as shown below...
Sample (AND ONLY) output:
Year 2020:
Building 1 has 10 floors, 20 apartments, and is 100% occupied. Full? true
Building 2 has 30 floors, 30 apartments, and is 75% occupied. Full? false
Many years pass.
Year 2043:
Building 1 has 10 floors, 20 apartments, and is 0% occupied. Full? false
Building 2 has 30 floors, 30 apartments, and is 100% occupied. Full? true
Looks like people prefer taller buildings.
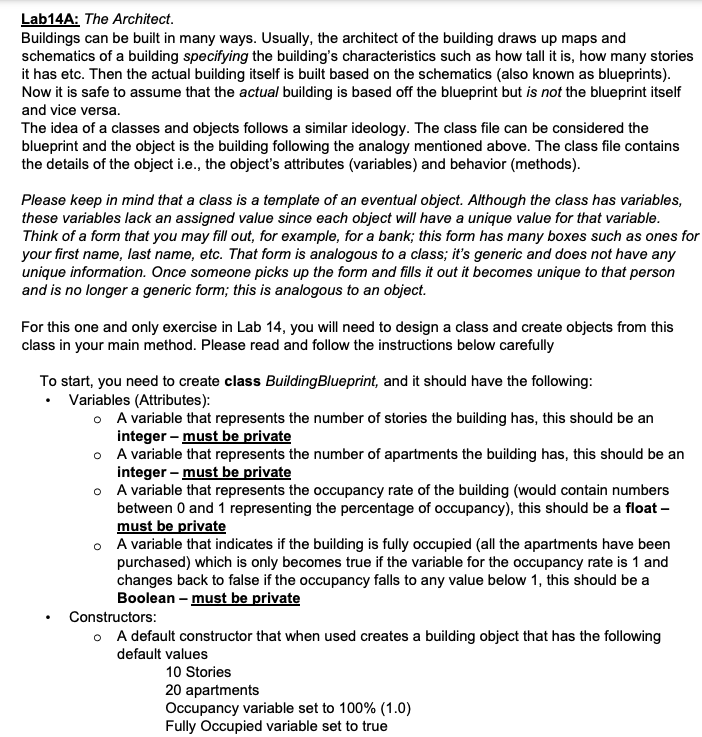
Transcribed Image Text:Lab14A: The Architect.
Buildings can be built in many ways. Usually, the architect of the building draws up maps and
schematics of a building specifying the building's characteristics such as how tall it is, how many stories
it has etc. Then the actual building itself is built based on the schematics (also known as blueprints).
Now it is safe to assume that the actual building is based off the blueprint but is not the blueprint itself
and vice versa.
The idea of a classes and objects follows a similar ideology. The class file can be considered the
blueprint and the object is the building following the analogy mentioned above. The class file contains
the details of the object i.e., the object's attributes (variables) and behavior (methods).
Please keep in mind that a class is a template of an eventual object. Although the class has variables,
these variables lack an assigned value since each object will have a unique value for that variable.
Think of a form that you may fill out, for example, for a bank; this form has many boxes such as ones for
your first name, last name, etc. That form is analogous to a class; it's generic and does not have any
unique information. Once someone picks up the form and fills it out it becomes unique to that person
and is no longer a generic form; this is analogous to an object.
For this one and only exercise in Lab 14, you will need to design a class and create objects from this
class in your main method. Please read and follow the instructions below carefully
To start, you need to create class BuildingBlueprint, and it should have the following:
• Variables (Attributes):
o A variable that represents the number of stories the building has, this should be an
integer – must be private
o A variable that represents the number of apartments the building has, this should be an
integer – must be private
o A variable that represents the occupancy rate of the building (would contain numbers
between 0 and 1 representing the percentage of occupancy), this should be a float –
must be private
o A variable that indicates if the building is fully occupied (all the apartments have been
purchased) which is only becomes true if the variable for the occupancy rate is 1 and
changes back to false if the occupancy falls to any value below 1, this should be a
Boolean - must be private
Constructors:
o A default constructor that when used creates a building object that has the following
default values
10 Stories
20 apartments
Occupancy variable set to 100% (1.0)
Fully Occupied variable set to true
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
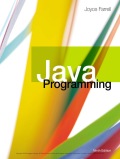
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
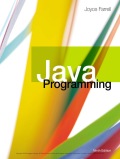
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT