Write a C++ program to add new details in the existing ArrayList and to remove details from the ArrayList. Use Menu to add, remove and display the Hall details. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names and function names should be the same as specified in the problem statement. Create Hall class with private member variables Data type Variables string name int contactNumber double costPerDay string ownerName Include appropriate getters, and setters and constructor (name,contactNumber,costPerDay,ownerName) in this order for the Hall class. Include following public member function in Hall class Method Name Description list getHallDetails() This method is used to return the hall details. [Hall details are already pre-populated in the template code] Create HallBO class and include following member function Method Name Description bool contains(list halls, string hallName ) This function is used to compare whether the hall list contains that hall name. If the hall name presents it returns true to remove that name otherwise it returns false. void display(list halls) This function is used to display the list of halls along with hardcoded details. Use "%-25s%-25d%-25.2f%-25s\n" to print table the details. In the main method test the above class and print the string "Hall Details:" in the main method itself Input and Output format: Print the costPerDay with two decimal values. [All text in bold corresponds to input and the rest corresponds to output] Sample Input and Output 1: 1.Add 2.Remove 3.Display Enter your choice 3 Hall Details: Hall Name Contact Number Cost Owner Chiltington 1798888137 30000.00 Robert Kilmersdon 1761436767 22000.00 James Press 1 to continue 2 Sample Input and Output 2: 1.Add 2.Remove 3.Display Enter your choice 1 Enter the details of Hall Hall Name : Mahal Contact Number : 1761436007 Cost per day : 22000 Owner Name : Akshay Press 1 to continue 1 1.Add 2.Remove 3.Display Enter your choice 1 Enter the details of Hall Hall Name : Manor Contact Number : 1515232061 Cost per day : 25000 Owner Name : Jack Press 1 to continue 1 1.Add 2.Remove 3.Display Enter your choice 3 Hall Details: Hall Name Contact Number Cost Owner Mahal 1761436007 22000.00 Akshay Manor 1515232061 25000.00 Jack Chiltington 1798888137 30000.00 Robert Kilmersdon 1761436767 22000.00 James Press 1 to continue 1 1.Add 2.Remove 3.Display Enter your choice 2 Enter the Hall name to be removed Kilmersdon Press 1 to continue 1 1.Add 2.Remove 3.Display Enter your choice 3 Hall Details: Hall Name Contact Number Cost Owner Mahal 1761436007 22000.00 Akshay Manor 1515232061 25000.00 Jack Chiltington 1798888137 30000.00 Robert Press 1 to continue 2 --------Strictly Use Below Template While Making Solution------------- main.cpp #include #include #include //#include "Hall.cpp" #include "HallBO.cpp" using namespace std; int main(){ int n, choice, p; string name, ownerName, hallName; int contactNumber; double costPerDay; static list hallList; list::iterator it; Hall h3; HallBO hbo; hallList = h3.getHallDetails(); do{ cout<<"1.Add\n"; cout<<"2.Remove\n"; cout<<"3.Display\n"; cout<<"Enter your choice\n"; cin>>choice; //fill the code }while(p==1); } HallBo.cpp #include #include "Hall.cpp" #include using namespace std; class HallBO{ public: Hall h; bool contains(list halls, string hallName){ //fill the code } void display(list halls){ //fill the code } }; Hall.cpp #include #include #include using namespace std; class Hall{ private: string name; int contactNumber; double costPerDay; string ownerName; public: Hall(){ } Hall(string name, int contactNumber, double costPerDay, string ownerName){ this->name = name; this->contactNumber = contactNumber; this->costPerDay = costPerDay; this->ownerName = ownerName; } list getHallDetails(){ list hallList; hallList.push_back(Hall("Chiltington",1798888137,30000,"Robert")); hallList.push_back(Hall("Kilmersdon",1761436767,22000,"James")); return hallList; } void setName(string name){ this->name=name; } string getName(){ return this->name; } void setContactNumber(int contactNumber){ this->contactNumber=contactNumber; } int getContactNumber(){ return this->contactNumber; } void setCostPerDay(double costPerDay){ this->costPerDay=costPerDay; } double getCostPerDay(){ return this->costPerDay; } void setOwnerName(string ownerName){ this->ownerName=ownerName; } string getOwnerName(){ return this->ownerName; } };
Write a C++ program to add new details in the existing ArrayList and to remove details from the ArrayList. Use Menu to add, remove and display the Hall details. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names and function names should be the same as specified in the problem statement. Create Hall class with private member variables Data type Variables string name int contactNumber double costPerDay string ownerName Include appropriate getters, and setters and constructor (name,contactNumber,costPerDay,ownerName) in this order for the Hall class. Include following public member function in Hall class Method Name Description list getHallDetails() This method is used to return the hall details. [Hall details are already pre-populated in the template code] Create HallBO class and include following member function Method Name Description bool contains(list halls, string hallName ) This function is used to compare whether the hall list contains that hall name. If the hall name presents it returns true to remove that name otherwise it returns false. void display(list halls) This function is used to display the list of halls along with hardcoded details. Use "%-25s%-25d%-25.2f%-25s\n" to print table the details. In the main method test the above class and print the string "Hall Details:" in the main method itself Input and Output format: Print the costPerDay with two decimal values. [All text in bold corresponds to input and the rest corresponds to output] Sample Input and Output 1: 1.Add 2.Remove 3.Display Enter your choice 3 Hall Details: Hall Name Contact Number Cost Owner Chiltington 1798888137 30000.00 Robert Kilmersdon 1761436767 22000.00 James Press 1 to continue 2 Sample Input and Output 2: 1.Add 2.Remove 3.Display Enter your choice 1 Enter the details of Hall Hall Name : Mahal Contact Number : 1761436007 Cost per day : 22000 Owner Name : Akshay Press 1 to continue 1 1.Add 2.Remove 3.Display Enter your choice 1 Enter the details of Hall Hall Name : Manor Contact Number : 1515232061 Cost per day : 25000 Owner Name : Jack Press 1 to continue 1 1.Add 2.Remove 3.Display Enter your choice 3 Hall Details: Hall Name Contact Number Cost Owner Mahal 1761436007 22000.00 Akshay Manor 1515232061 25000.00 Jack Chiltington 1798888137 30000.00 Robert Kilmersdon 1761436767 22000.00 James Press 1 to continue 1 1.Add 2.Remove 3.Display Enter your choice 2 Enter the Hall name to be removed Kilmersdon Press 1 to continue 1 1.Add 2.Remove 3.Display Enter your choice 3 Hall Details: Hall Name Contact Number Cost Owner Mahal 1761436007 22000.00 Akshay Manor 1515232061 25000.00 Jack Chiltington 1798888137 30000.00 Robert Press 1 to continue 2 --------Strictly Use Below Template While Making Solution------------- main.cpp #include #include #include //#include "Hall.cpp" #include "HallBO.cpp" using namespace std; int main(){ int n, choice, p; string name, ownerName, hallName; int contactNumber; double costPerDay; static list hallList; list::iterator it; Hall h3; HallBO hbo; hallList = h3.getHallDetails(); do{ cout<<"1.Add\n"; cout<<"2.Remove\n"; cout<<"3.Display\n"; cout<<"Enter your choice\n"; cin>>choice; //fill the code }while(p==1); } HallBo.cpp #include #include "Hall.cpp" #include using namespace std; class HallBO{ public: Hall h; bool contains(list halls, string hallName){ //fill the code } void display(list halls){ //fill the code } }; Hall.cpp #include #include #include using namespace std; class Hall{ private: string name; int contactNumber; double costPerDay; string ownerName; public: Hall(){ } Hall(string name, int contactNumber, double costPerDay, string ownerName){ this->name = name; this->contactNumber = contactNumber; this->costPerDay = costPerDay; this->ownerName = ownerName; } list getHallDetails(){ list hallList; hallList.push_back(Hall("Chiltington",1798888137,30000,"Robert")); hallList.push_back(Hall("Kilmersdon",1761436767,22000,"James")); return hallList; } void setName(string name){ this->name=name; } string getName(){ return this->name; } void setContactNumber(int contactNumber){ this->contactNumber=contactNumber; } int getContactNumber(){ return this->contactNumber; } void setCostPerDay(double costPerDay){ this->costPerDay=costPerDay; } double getCostPerDay(){ return this->costPerDay; } void setOwnerName(string ownerName){ this->ownerName=ownerName; } string getOwnerName(){ return this->ownerName; } };
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter12: Adding Functionality To Your Classes
Section12.2: Providing Class Conversion Capabilities
Problem 2E
Related questions
Question
100%
Write a C++ program to add new details in the existing ArrayList and to remove details from the ArrayList. Use Menu to add, remove and display the Hall details.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names and function names should be the same as specified in the problem statement.
Create Hall class with private member variables
Data type | Variables |
string | name |
int | contactNumber |
double | costPerDay |
string | ownerName |
Include appropriate getters, and setters and constructor (name,contactNumber,costPerDay,ownerName) in this order for the Hall class.
Include following public member function in Hall class
Method Name | Description |
list<Hall> getHallDetails() | This method is used to return the hall details. [Hall details are already pre-populated in the template code] |
Create HallBO class and include following member function
Method Name | Description |
bool contains(list <Hall> halls, string hallName ) | This function is used to compare whether the hall list contains that hall name. If the hall name presents it returns true to remove that name otherwise it returns false. |
void display(list<Hall> halls) | This function is used to display the list of halls along with hardcoded details. Use "%-25s%-25d%-25.2f%-25s\n" to print table the details. |
In the main method test the above class and print the string "Hall Details:" in the main method itself
Input and Output format:
Print the costPerDay with two decimal values.
[All text in bold corresponds to input and the rest corresponds to output]
Sample Input and Output 1:
Sample Input and Output 1:
1.Add
2.Remove
3.Display
Enter your choice
3
Hall Details:
Hall Name Contact Number Cost Owner
Chiltington 1798888137 30000.00 Robert
Kilmersdon 1761436767 22000.00 James
Press 1 to continue
2
Sample Input and Output 2:
1.Add
1.Add
2.Remove
3.Display
Enter your choice
1
Enter the details of Hall
Hall Name :
Mahal
Contact Number :
1761436007
Cost per day :
22000
Owner Name :
Akshay
Press 1 to continue
1
1.Add
2.Remove
3.Display
Enter your choice
1
Enter the details of Hall
Hall Name :
Manor
Contact Number :
1515232061
Cost per day :
25000
Owner Name :
Jack
Press 1 to continue
1
1.Add
2.Remove
3.Display
Enter your choice
3
Hall Details:
Hall Details:
Hall Name Contact Number Cost Owner
Mahal 1761436007 22000.00 Akshay
Manor 1515232061 25000.00 Jack
Chiltington 1798888137 30000.00 Robert
Kilmersdon 1761436767 22000.00 James
Press 1 to continue
1
1.Add
2.Remove
3.Display
Enter your choice
2
Enter the Hall name to be removed
Kilmersdon
Press 1 to continue
1
1.Add
2.Remove
3.Display
Enter your choice
3
Hall Details:
Hall Details:
Hall Name Contact Number Cost Owner
Mahal 1761436007 22000.00 Akshay
Manor 1515232061 25000.00 Jack
Chiltington 1798888137 30000.00 Robert
Press 1 to continue
2
--------Strictly Use Below Template While Making Solution-------------
main.cpp
#include <iostream> #include <stdio.h> #include<list> //#include "Hall.cpp" #include "HallBO.cpp" using namespace std; int main(){ int n, choice, p; string name, ownerName, hallName; int contactNumber; double costPerDay; static list<Hall> hallList; list<Hall>::iterator it; Hall h3; HallBO hbo; hallList = h3.getHallDetails(); do{ cout<<"1.Add\n"; cout<<"2.Remove\n"; cout<<"3.Display\n"; cout<<"Enter your choice\n"; cin>>choice; //fill the code }while(p==1); } |
HallBo.cpp
#include<iostream> #include "Hall.cpp" #include<list> using namespace std; class HallBO{ public: Hall h; bool contains(list <Hall> halls, string hallName){ //fill the code } void display(list<Hall> halls){ //fill the code } }; |
Hall.cpp
#include <iostream> #include <stdio.h> #include<list> using namespace std; class Hall{ private: string name; int contactNumber; double costPerDay; string ownerName; public: Hall(){ } Hall(string name, int contactNumber, double costPerDay, string ownerName){ this->name = name; this->contactNumber = contactNumber; this->costPerDay = costPerDay; this->ownerName = ownerName; } list<Hall> getHallDetails(){ list<Hall> hallList; hallList.push_back(Hall("Chiltington",1798888137,30000,"Robert")); hallList.push_back(Hall("Kilmersdon",1761436767,22000,"James")); return hallList; } void setName(string name){ this->name=name; } string getName(){ return this->name; } void setContactNumber(int contactNumber){ this->contactNumber=contactNumber; } int getContactNumber(){ return this->contactNumber; } void setCostPerDay(double costPerDay){ this->costPerDay=costPerDay; } double getCostPerDay(){ return this->costPerDay; } void setOwnerName(string ownerName){ this->ownerName=ownerName; } string getOwnerName(){ return this->ownerName; } }; |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Recommended textbooks for you
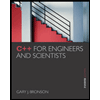
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
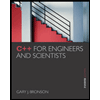
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr