Often times after a big meal, we may find ourselves considering how much we need to exercise to work off what we just ate. In this exercise we will actually compute it. Write a program FitnessPlanner_1 that asks the user to enter his/her weight in lbs, how many chicken nuggets were eaten, average walk speed in mph (typically 3 mph, which is a 20-minute mile pace), and average run speed in mph (typically 6 mph, which is a 10-minute mile pace). The program should then output how long the user needs to walk and how long the user needs to run in order to burn those chicken nuggets off. 1) Oxygen consumption (VO2) can be used as an indicator of exercise intensity, which is closely tied to energy expenditure. In other words, VO2 can be used to approximate how many calories are burned during an activity. The approximate VO2 for walking (on flat land) at a given speed (mph) can be computed as: Declare the calories of a chicken nugget as a global constant. vO2 for walking = 2.68224 x speed3.5 The approximate VO2 for running (on flat land) at a given speed (mph) can be computed as: ***This Fitness Planner program will calculate how long you need to exercise to burn off what you just ate. *** *** vO2 for running = 5.36448 x speed3.5 What is your weight in pounds? 125 2) The calorie burn rate (CBR) is the number of calories burned per minute when doing a certain activity. A simplified way to compute the CBR from VO2 and a person's weight (lbs) is given by the formula: How many chicken nuggets did you eat? (2.268 x 103) x VO2 x weight 20 CBR What is your average WALKING speed in mph (3 is typical, a 20-min/mi pace)? 3 You need to write three (3) versions of a program that calculates how many minutes one needs to exercise to burn off a given amount of food they ate. To calculate the number of minutes required to burn off a given number of calories, simply divide the total calories by the calorie burn rate. What is your average RUNNING speed in mph (6 is typical, a 10-min/mi pace)? In the descriptions for PART A, PART B, and PART C, I use "chicken nuggets" as the food of choice. Assume that chicken nugget has 47 calories each. However, in your program you may use whatever type of food you want.. You need to walk for 287 minutes to burn off the 20 chicken nuggets you ate You need to run for 81 minutes to burn off the 20 chicken nuggets ycu ate Make a copy of the previous program and rename the class to FitnessPlanner_2. This time, before asking the user to enter a walking or running speed, ask the user if he/she prefers walking or running by asking the user to input "W" for walking or "R" for running. You then should only ask the user to enter the walking speed OR the running speed according to the user's response to this query. Likewise, use the appropriate formula (for walking or for running). Make a copy of the previous program and rename the class to FitnessPlanner_3. This time, instead of asking the user how many chicken nuggets he/she ate, ask for how much time the user will have to run or walk. Your program should then tell the user how many chicken nuggets he/she is allowed to eat without accumulating surplus calories, assuming they follow through with their exercise commitment. Also display how many calories will be burned When you read the letters w/W or r/R from the user, save them as strings. The method to read a string is next(). Example: = new Scanner (System.in); Scanner keyboard System.out.println("Enter your gender: "); String gender ***This Fitness Planner program will calculate how much food *** keyboard.next () ; you can eat and how many calories you need to burn off. This Fitness Planner program will calculate how long you need to exercise to burn off what you just ate. What is your weight in pounds? *** 115 What is your weight in pounds? 150 Do you prefer walking or running (W or R)? How many chicken nuggets did you eat? What is your average RUNNING speed in mph (6 is typical, a 10-min/mi pace)? 12 8 Do you prefer walking or running (W R)? How many minutes will you run? W a 20-min/mi pace)? What is your average WALKING speed in mph (3 is typical, 30 2 Congratulations, you are allowed to eat 7 chicken nuggets You need to walk for 187 minutes to burn off the 12 chicken nuggets you ate. You will burn off 363 calories later.
Often times after a big meal, we may find ourselves considering how much we need to exercise to work off what we just ate. In this exercise we will actually compute it. Write a program FitnessPlanner_1 that asks the user to enter his/her weight in lbs, how many chicken nuggets were eaten, average walk speed in mph (typically 3 mph, which is a 20-minute mile pace), and average run speed in mph (typically 6 mph, which is a 10-minute mile pace). The program should then output how long the user needs to walk and how long the user needs to run in order to burn those chicken nuggets off. 1) Oxygen consumption (VO2) can be used as an indicator of exercise intensity, which is closely tied to energy expenditure. In other words, VO2 can be used to approximate how many calories are burned during an activity. The approximate VO2 for walking (on flat land) at a given speed (mph) can be computed as: Declare the calories of a chicken nugget as a global constant. vO2 for walking = 2.68224 x speed3.5 The approximate VO2 for running (on flat land) at a given speed (mph) can be computed as: ***This Fitness Planner program will calculate how long you need to exercise to burn off what you just ate. *** *** vO2 for running = 5.36448 x speed3.5 What is your weight in pounds? 125 2) The calorie burn rate (CBR) is the number of calories burned per minute when doing a certain activity. A simplified way to compute the CBR from VO2 and a person's weight (lbs) is given by the formula: How many chicken nuggets did you eat? (2.268 x 103) x VO2 x weight 20 CBR What is your average WALKING speed in mph (3 is typical, a 20-min/mi pace)? 3 You need to write three (3) versions of a program that calculates how many minutes one needs to exercise to burn off a given amount of food they ate. To calculate the number of minutes required to burn off a given number of calories, simply divide the total calories by the calorie burn rate. What is your average RUNNING speed in mph (6 is typical, a 10-min/mi pace)? In the descriptions for PART A, PART B, and PART C, I use "chicken nuggets" as the food of choice. Assume that chicken nugget has 47 calories each. However, in your program you may use whatever type of food you want.. You need to walk for 287 minutes to burn off the 20 chicken nuggets you ate You need to run for 81 minutes to burn off the 20 chicken nuggets ycu ate Make a copy of the previous program and rename the class to FitnessPlanner_2. This time, before asking the user to enter a walking or running speed, ask the user if he/she prefers walking or running by asking the user to input "W" for walking or "R" for running. You then should only ask the user to enter the walking speed OR the running speed according to the user's response to this query. Likewise, use the appropriate formula (for walking or for running). Make a copy of the previous program and rename the class to FitnessPlanner_3. This time, instead of asking the user how many chicken nuggets he/she ate, ask for how much time the user will have to run or walk. Your program should then tell the user how many chicken nuggets he/she is allowed to eat without accumulating surplus calories, assuming they follow through with their exercise commitment. Also display how many calories will be burned When you read the letters w/W or r/R from the user, save them as strings. The method to read a string is next(). Example: = new Scanner (System.in); Scanner keyboard System.out.println("Enter your gender: "); String gender ***This Fitness Planner program will calculate how much food *** keyboard.next () ; you can eat and how many calories you need to burn off. This Fitness Planner program will calculate how long you need to exercise to burn off what you just ate. What is your weight in pounds? *** 115 What is your weight in pounds? 150 Do you prefer walking or running (W or R)? How many chicken nuggets did you eat? What is your average RUNNING speed in mph (6 is typical, a 10-min/mi pace)? 12 8 Do you prefer walking or running (W R)? How many minutes will you run? W a 20-min/mi pace)? What is your average WALKING speed in mph (3 is typical, 30 2 Congratulations, you are allowed to eat 7 chicken nuggets You need to walk for 187 minutes to burn off the 12 chicken nuggets you ate. You will burn off 363 calories later.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
How do I solve this problem that deals with counting and subtracting calories in Java without using arrays or lists? It has 3 steps. thanks :<)
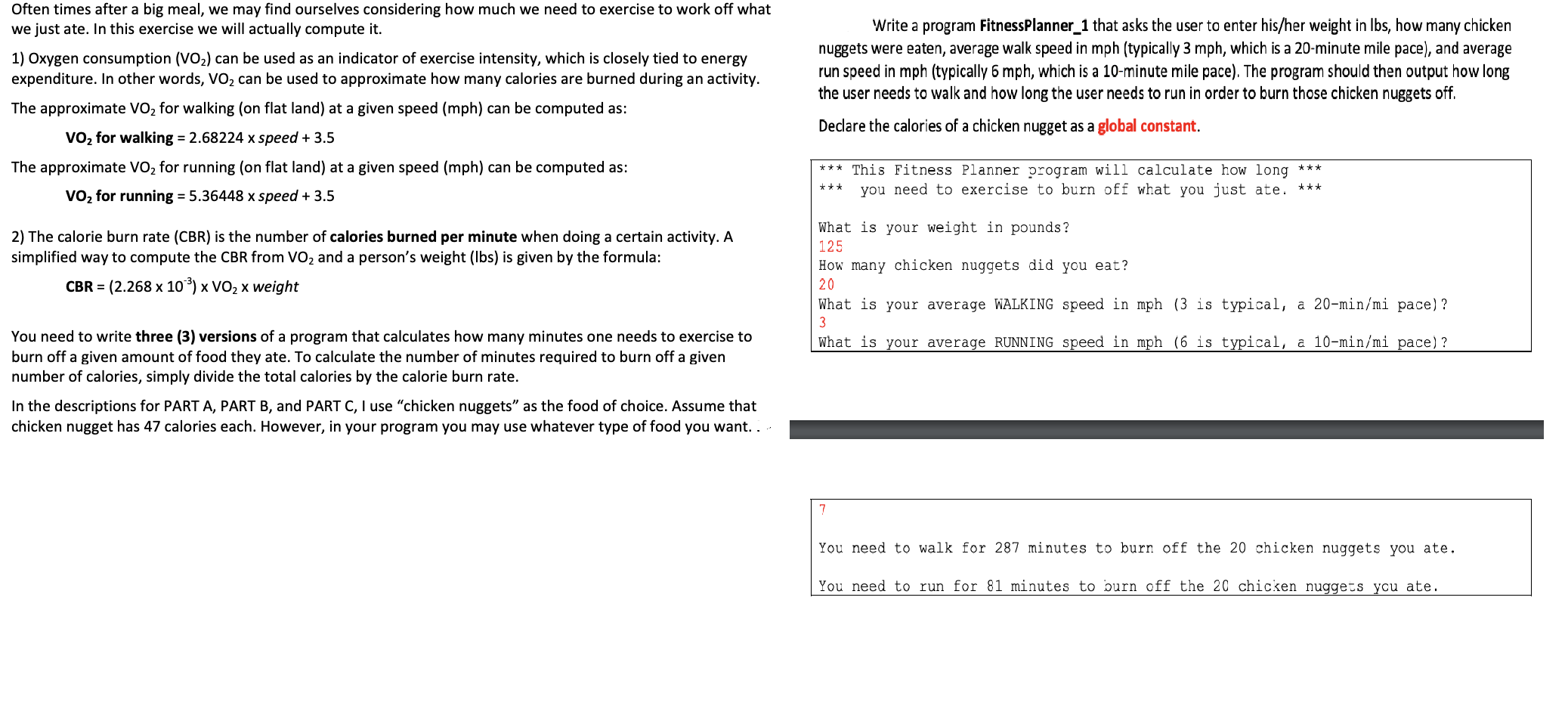
Transcribed Image Text:Often times after a big meal, we may find ourselves considering how much we need to exercise to work off what
we just ate. In this exercise we will actually compute it.
Write a program FitnessPlanner_1 that asks the user to enter his/her weight in lbs, how many chicken
nuggets were eaten, average walk speed in mph (typically 3 mph, which is a 20-minute mile pace), and average
run speed in mph (typically 6 mph, which is a 10-minute mile pace). The program should then output how long
the user needs to walk and how long the user needs to run in order to burn those chicken nuggets off.
1) Oxygen consumption (VO2) can be used as an indicator of exercise intensity, which is closely tied to energy
expenditure. In other words, VO2 can be used to approximate how many calories are burned during an activity.
The approximate VO2 for walking (on flat land) at a given speed (mph) can be computed as:
Declare the calories of a chicken nugget as a global constant.
vO2 for walking = 2.68224 x speed3.5
The approximate VO2 for running (on flat land) at a given speed (mph) can be computed as:
***This Fitness Planner program will calculate how long
you need to exercise to burn off what you just ate. ***
***
vO2 for running = 5.36448 x speed3.5
What is your weight in pounds?
125
2) The calorie burn rate (CBR) is the number of calories burned per minute when doing a certain activity. A
simplified way to compute the CBR from VO2 and a person's weight (lbs) is given by the formula:
How many chicken nuggets did you eat?
(2.268 x 103) x VO2 x weight
20
CBR
What is your average WALKING speed in mph (3 is typical, a 20-min/mi pace)?
3
You need to write three (3) versions of a program that calculates how many minutes one needs to exercise to
burn off a given amount of food they ate. To calculate the number of minutes required to burn off a given
number of calories, simply divide the total calories by the calorie burn rate.
What is your average RUNNING speed in mph (6 is typical, a 10-min/mi pace)?
In the descriptions for PART A, PART B, and PART C, I use "chicken nuggets" as the food of choice. Assume that
chicken nugget has 47 calories each. However, in your program you may use whatever type of food you want..
You need to walk for 287 minutes to burn off the 20 chicken nuggets you ate
You need to run for 81 minutes to burn off the 20 chicken nuggets ycu ate
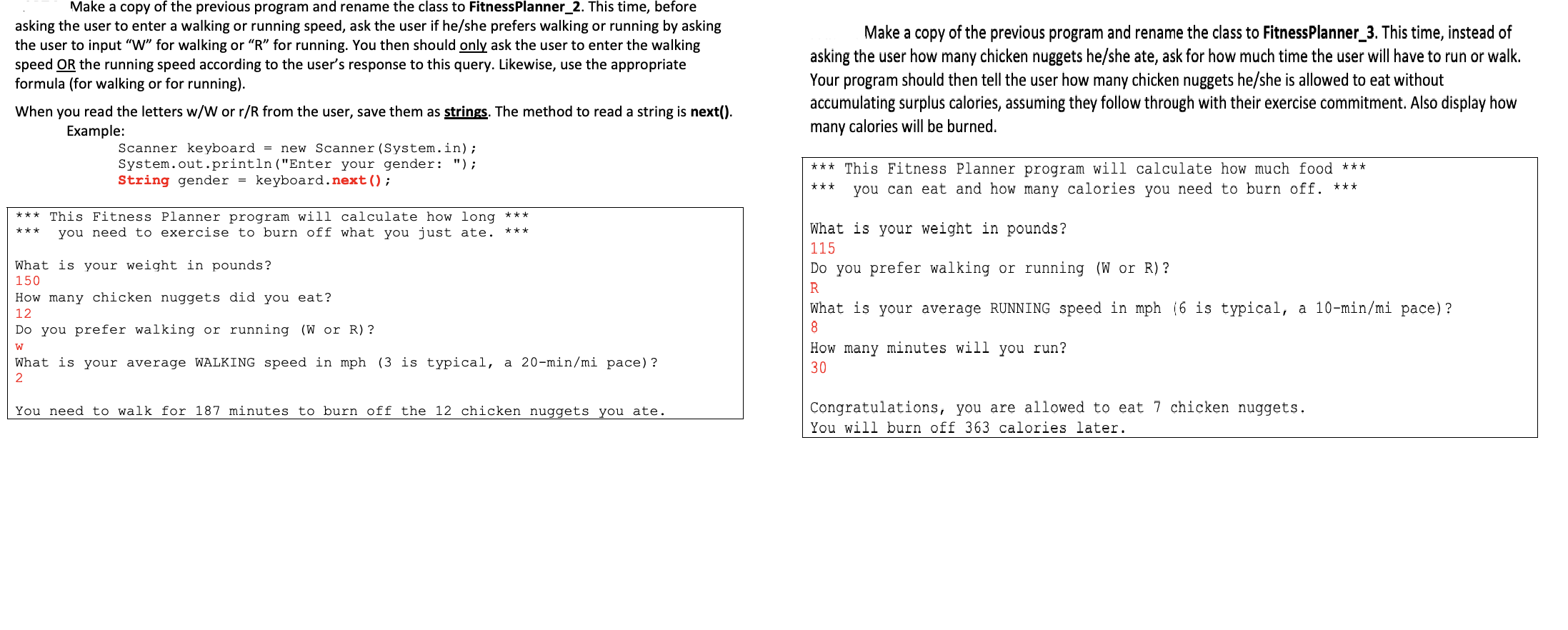
Transcribed Image Text:Make a copy of the previous program and rename the class to FitnessPlanner_2. This time, before
asking the user to enter a walking or running speed, ask the user if he/she prefers walking or running by asking
the user to input "W" for walking or "R" for running. You then should only ask the user to enter the walking
speed OR the running speed according to the user's response to this query. Likewise, use the appropriate
formula (for walking or for running).
Make a copy of the previous program and rename the class to FitnessPlanner_3. This time, instead of
asking the user how many chicken nuggets he/she ate, ask for how much time the user will have to run or walk.
Your program should then tell the user how many chicken nuggets he/she is allowed to eat without
accumulating surplus calories, assuming they follow through with their exercise commitment. Also display how
many calories will be burned
When you read the letters w/W or r/R from the user, save them as strings. The method to read a string is next().
Example:
= new Scanner (System.in);
Scanner keyboard
System.out.println("Enter your gender: ");
String gender
***This Fitness Planner program will calculate how much food ***
keyboard.next () ;
you can eat and how many calories you need to burn off.
This Fitness Planner program will calculate how long
you need to exercise to burn off what you just ate.
What is your weight in pounds?
***
115
What is your weight in pounds?
150
Do you prefer walking
or running (W or R)?
How many chicken nuggets did you eat?
What is your average RUNNING speed in mph (6 is typical, a 10-min/mi pace)?
12
8
Do you prefer walking or running (W
R)?
How many minutes will you run?
W
a 20-min/mi pace)?
What is your average WALKING speed in mph (3 is typical,
30
2
Congratulations, you are allowed to eat 7 chicken nuggets
You need to walk for 187 minutes to burn off the 12 chicken nuggets you ate.
You will burn off 363 calories later.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
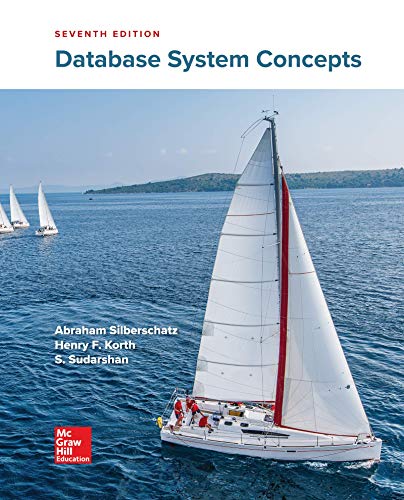
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
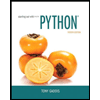
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
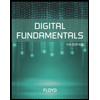
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
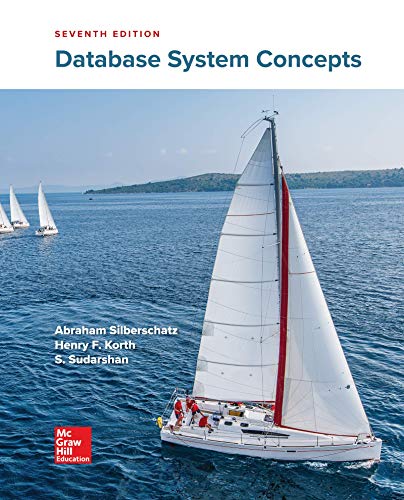
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
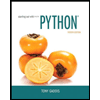
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
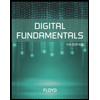
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
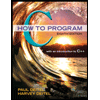
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
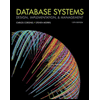
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
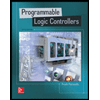
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education