Consider the following Java program. Which line give the "TestFx" class access to the "Button" class import javafx.application.Application; import javafx.scene.Scene; import javafx.state.Stage; import javafx.application.Platform; import javafx.scene.layout.BorderPane; import javafx.scene.layout.HBox; import javafx.scene.control.Button; public class TestFx extends Application { public void start(Stage.stage) { Button quitButton = newButton('Quit"); quitButton.setOnAction(e -> Platform.exit()); HBox buttonBar = new HBox(quitButton); BorderPane root = new BorderPane(); root.setBottom(buttonBar); Scene.scene = new Scene(root, 100, 50); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } } a. Button quitButton = newButton("Quit"); b. import javafx.application.Application c. import javafx.scene.control.Button; d. root.setBottom(buttonBar); e. public class TestFx extends Application
Consider the following Java program. Which line give the "TestFx" class access to the "Button" class
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.state.Stage;
import javafx.application.Platform;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.control.Button;
public class TestFx extends Application {
public void start(Stage.stage) {
Button quitButton = newButton('Quit");
quitButton.setOnAction(e -> Platform.exit());
HBox buttonBar = new HBox(quitButton);
BorderPane root = new BorderPane();
root.setBottom(buttonBar);
Scene.scene = new Scene(root, 100, 50);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
a. Button quitButton = newButton("Quit");
b. import javafx.application.Application
c. import javafx.scene.control.Button;
d. root.setBottom(buttonBar);
e. public class TestFx extends Application

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

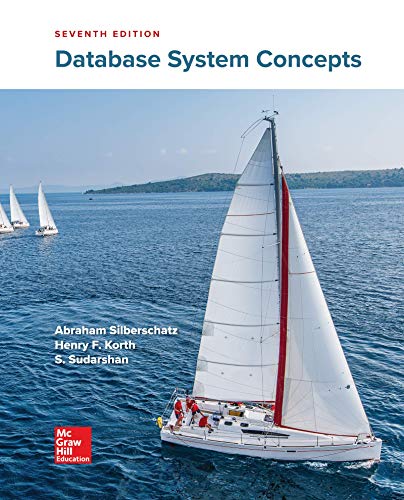
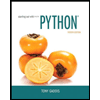
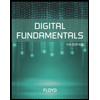
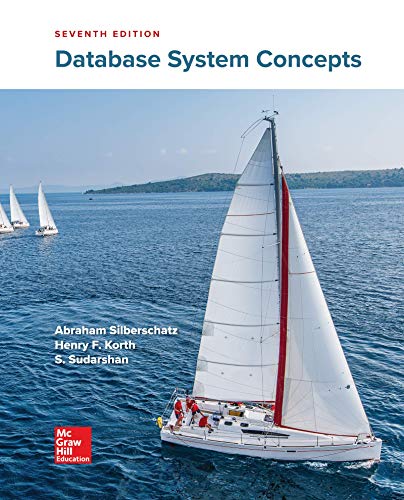
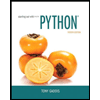
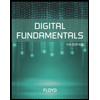
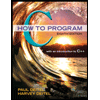
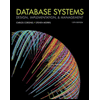
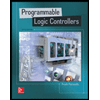