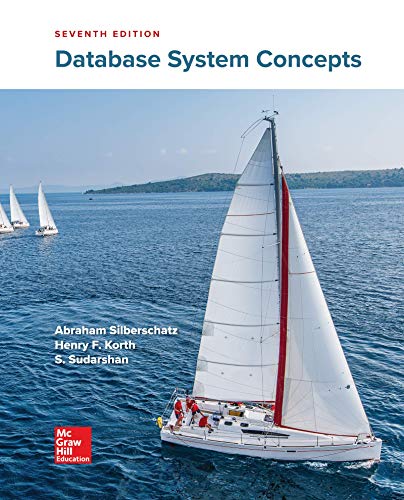
Concept explainers
03 Inheritance / Polymorphism
Task 1
Currently, you are using composition by creating an instance of a
C++ derive AddressBook from vector. In other words, use the vector as a base class and derive AddressBook from it. This means that you will need to remove the Vector from the private section of your class.
You are going to have to make some fundamental changes to your AddressBook so that you are using the vector / ArrayList functionality to store and retrieve people. In other words, you should be accessing the ArrayList / vector using this. C++ people, don’t forget you are returning pointers in the accessors of the AddressBook class
Test your AddressBook the same way you did for assignment 2. If you have coded everything properly it should work the same.
Task 2
Create class called Player that is derived from Person. The Player class should hold the following information
- Average
- Slugging Percentage
- On Base Percentage
All should be double data types
Constructors
- Default
- Player(string first, string last, string address, double average, double slugging, double onBase)
You should delegate the default constructor to eliminate multiple code paths.
Mutators
- void setAverage(double avg
- void setSlugging(double slug)
- void setOBP(double OnBase)
Accessors
- double getAverage()
- double getSlugging()
- double getOBP()
print function
In the Person class add a public function called print. This function will print out the person’s first, last, address. This function should be a virtual function or method. In essence all overridden methods in Java are virtual Java students don't need to do anything except override the method.
In Player override the print function so that you print out the players name, address, average, slugging %, On base %.
To print the information from the Person class you should call the print function from the Player print function.
Test your new class by creating a couple of Players. Make sure you test all functionality and that your print function prints everything from both Person and Player.
Can you help me solve this problem in C++ forms using the Inheritance / Polymorphism
If you decide to create in Java then as least please transform the Java into C++ and explain to me in C++ form because I never took Java.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Topical Information Use C++. This lab will help you practice with dynamic memory (NOT mixed with classes). Program Information Heat flow through a rod can be simulated fairly easily in a computer program. We can simulate the rod using an array of temperatures. The rod begins all at the same temperature (user determined), but holding some position(s) of the rod at constant temperature (holding a match or ice cube to the rod) provides a [set of] heat source(s) (or sink(s)). To update the temperature at each time step (second?), you take the average of the positions on each side from the previous time step (and at the current position). For instance, if the following was the initial state of the rod: +------+------+------+------+------+------+------+------+ | 10 | 10 | 10 | 10 | 10 | 10 | 10 | 10 | +------+------+------+------+------+------+------+------+ And then the user specifies that there is a heat source at the left end of 100 degrees:…arrow_forwardWrite a simple trivia quiz game using c++ Start by creating a Trivia class that contains information about a single trivia question. The class should contain a string for the question, a string for the answer to the question, and an integer representing the dollar amount the question is worth (harder questions should be worth more). Add appropriate constructor and accessor functions. In your main function create either an array or a vector of type Trivia and hard-code at least five trivia questions of your choice. Your program should then ask each question to the player, input the player’s answer, and check if the player’s answer matches the actual answer. If so, award the player the dollar amount for that question. If the player enters the wrong answer your program should display the correct answer. When all questions have been asked display the total amount that the player has won.arrow_forwardplease help me with codingarrow_forward
- Course: Data Structure and Algorithims Language: Java Kindly make the program in 2 hours. Task is well explained. You have to make the proogram properly in Java: Restriction: Prototype cannot be change you have to make program by using given prototype. TAsk: Create a class Node having two data members int data; Node next; Write the parametrized constructor of the class Node which contain one parameter int value assign this value to data and assign next to null Create class LinkList having one data members of type Node. Node head Write the following function in the LinkList class publicvoidinsertAtLast(int data);//this function add node at the end of the list publicvoid insertAthead(int data);//this function add node at the head of the list publicvoid deleteNode(int key);//this function find a node containing "key" and delete it publicvoid printLinkList();//this function print all the values in the Linklist public LinkListmergeList(LinkList l1,LinkList l2);// this function…arrow_forwardcreate using c++ One problem with dynamic arrays is that once the array is created using the new operator the size cannot be changed. For example, you might want to add or delete entries from the array similar to the behavior of a vector . This project asks you to create a class called DynamicStringArray that includes member functions that allow it to emulate the behavior of a vector of strings. The class should have the following A private member variable called dynamicArray that references a dynamic array of type string. A private member variable called size that holds the number of entries in the array. A default constructor that sets the dynamic array to NULL and sets size to 0. A function that returns size . A function named addEntry that takes a string as input. The function should create a new dynamic array one element larger than dynamicArray , copy all elements from dynamicArray into the new array, add the new string onto the end of the new array, increment size, delete the…arrow_forwardC++ Create a function append(v1, v2) that adds a copy of all the elements of vector v1 to the end of vector v2. The arguments are vectors of integers. Write a test driver.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
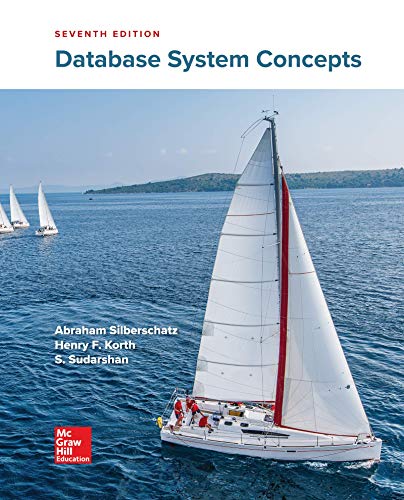
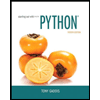
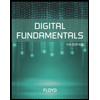
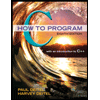
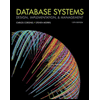
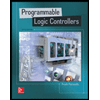