Part 3: Negative Binary Numbers This standard method of binary number representation doesn't account for negative numbers. We can't just throw a negative sign in front of a binary number though - everything in a computer must be represented by 1's and 0's. One way we can get around this is by using the very first digit of each binary number to represent the sign: 0 for positive and 1 for negative. This is called 1's complement representation. 00010110 -> 0 0010110 -> positive 22 10010110 -> 1 0010110 -> negative 22 Using 1's complement representation we can represent the numbers -127 through 127 in a single byte (note that we can only go half as high as an unsigned number because half of the possible representations for that byte are being used for the negative numbers). However, there is a flaw with 1's complement: the representation of the number 0. 00000000 -> 0 0000000 -> zero 10000000 -> 1 0000000 -> negative zero? Because of this, we usually prefer to use 2's complement representation, which cleverly avoids the double 0 issue while still using the first digit of the binary number to show the sign. Even in 2's complement representation, if the first digit is 0 the number is positive (or 0), and if the first digit if 1 the number is negative. You can convert a positive binary number to it's 2's complement negative by following these steps: Reverse each bit of the number (0's turn to 1's and 1's turn to 0's) Add 1 For example, lets say we want to represent -99 in binary: 99 in binary is 01100011 Flipping all of the bits gets us 10011100 Adding 1 gets us 10011101 Therefore 10011101 is -99 in 2's complement binary. The process to convert a negative binary number to a positive one is the exact same: -99 in binary is 10011101 Flipping all of the bits gets us 01100010 Adding 1 gets us 01100011 01100011 is 99 in binary, just like we started with So far we've only shown negative numbers using 8 digit binary numbers. Like I mentioned above, we usually use this size because that represents a byte in the computer's memory. We could just as easily write our 2's complement binary numbers using any number of digits, and in that case the leftmost digit will always represent the sign of the number. Exercise 6: How would you represent the number -5 in 2's complement binary? Show your answer as an 8 digit number. Another convenient aspect of 2's complement binary representation is that it remains consistent with addition, which means we can add positive and negative binary numbers and get the mathematically correct result. This also allows us to easily perform subtraction: to subtract, reverse the sign of the subtracted number by doing the 2's complement conversion, then add that to your other number. Exercise 7: What is 01010010 - 00001110? Show the result in binary. (You can check your work by converting all of the numbers to decimal and verifying that the result is correct)
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
Part 3: Negative Binary Numbers
This standard method of binary number representation doesn't account for negative numbers. We can't just throw a negative sign in front of a binary number though - everything in a computer must be represented by 1's and 0's. One way we can get around this is by using the very first digit of each binary number to represent the sign: 0 for positive and 1 for negative. This is called 1's complement representation.
00010110 -> 0 0010110 -> positive 22
10010110 -> 1 0010110 -> negative 22
Using 1's complement representation we can represent the numbers -127 through 127 in a single byte (note that we can only go half as high as an unsigned number because half of the possible representations for that byte are being used for the negative numbers). However, there is a flaw with 1's complement: the representation of the number 0.
00000000 -> 0 0000000 -> zero
10000000 -> 1 0000000 -> negative zero?
Because of this, we usually prefer to use 2's complement representation, which cleverly avoids the double 0 issue while still using the first digit of the binary number to show the sign. Even in 2's complement representation, if the first digit is 0 the number is positive (or 0), and if the first digit if 1 the number is negative. You can convert a positive binary number to it's 2's complement negative by following these steps:
- Reverse each bit of the number (0's turn to 1's and 1's turn to 0's)
- Add 1
For example, lets say we want to represent -99 in binary:
99 in binary is 01100011
Flipping all of the bits gets us 10011100
Adding 1 gets us 10011101
Therefore 10011101 is -99 in 2's complement binary.
The process to convert a negative binary number to a positive one is the exact same:
-99 in binary is 10011101
Flipping all of the bits gets us 01100010
Adding 1 gets us 01100011
01100011 is 99 in binary, just like we started with
So far we've only shown negative numbers using 8 digit binary numbers. Like I mentioned above, we usually use this size because that represents a byte in the computer's memory. We could just as easily write our 2's complement binary numbers using any number of digits, and in that case the leftmost digit will always represent the sign of the number.
Exercise 6: How would you represent the number -5 in 2's complement binary? Show your answer as an 8 digit number.
Another convenient aspect of 2's complement binary representation is that it remains consistent with addition, which means we can add positive and negative binary numbers and get the mathematically correct result. This also allows us to easily perform subtraction: to subtract, reverse the sign of the subtracted number by doing the 2's complement conversion, then add that to your other number.
Exercise 7: What is 01010010 - 00001110? Show the result in binary. (You can check your work by converting all of the numbers to decimal and verifying that the result is correct)

Step by step
Solved in 3 steps

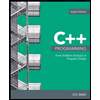
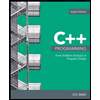