Payroll System In this lab, you're going to be working with partially filled arrays that are parallel with each other. That means that the row index in multiple arrays identifies different pieces of data for the same person. This is a simple payroll system that just calculates gross pay given a set of employees, hours worked for the week and hourly rate. Parallel Arrays First, you have to define several arrays in your main program: 1. employee names for each employee 2 hourly pay rate for each employee 3. total hours worked for each employee 4. gross pay for each employee Second, you will need a two dimension (2-D) array to record the hours worked each day for an employee. The number of rows for the 2-D array should be the same as the arrays above since each row corresponds to an employee. The number of columns represents days of the week (7 last I looked). Functions Needed In this lab, you must read in the employee names first because this identifies how many elements will be in each array. Then you wll have to read in the hourly pay rate for each employee. You must implement two functions: getEmployeeNames and getHourlyPay. /* TODO: Create a function called getEmployeeNames Arguments : Name array(first and last name) Maximum size Return the number of names filled. /* TODO: Create a function called getHourlyPay Arguments NameArray(filled in Step 1) HourlyPayArray(starts empty) NumberOfNamesFilled(from Stepl) Two additional functions: getHighestPay and getlowestPay should use the gross pay array and the number of employees to figure out the highest and lowest pay respectively. HINT: return the index of the highest/lowest gross pay so that when you print the answer in main, you can access the gross pay and the name. Nested Loops for Daily Hours Once you have the names and hourly pay rate, you have to ask the user to enter the hours worked for each employee. Expect to read in hours for 7 days, the user will put 0 for days off. This will require a loop that traverses the rows (each employee) and another loop that traverses the hours worked for the days. Another set of nested loops are needed to sum up the daily hours into total hours worked and use that to calculate the gross pay for the week. Once you have this, you now have everything you need to create the table which contains only the employee names, the total hours worked and the gross pay (no need to traverse the 2-D array again!). Program Output Employee Name, Total Hours and Gross Pay. Use the output information below to space your output in the three arrays that will be printed: employee names, total hours and gross pay. cout <« setw (20) << "Employee Name" << setw (7) << " Hours" << setw (10) << "Gross Pay" << endl; Again, you are working with parallel arrays, so use the same index to access elements in each array for an employee. Employee Name, Total Hours and Gross Pay. Finally, use the funictions that you wrote to calculate the highest and lowest gross pay and print this info according to the test case output. These functions only need the gross pay array and the number of elements in it. Each function should return the index of the target value in the gross pay array.
Payroll System In this lab, you're going to be working with partially filled arrays that are parallel with each other. That means that the row index in multiple arrays identifies different pieces of data for the same person. This is a simple payroll system that just calculates gross pay given a set of employees, hours worked for the week and hourly rate. Parallel Arrays First, you have to define several arrays in your main program: 1. employee names for each employee 2 hourly pay rate for each employee 3. total hours worked for each employee 4. gross pay for each employee Second, you will need a two dimension (2-D) array to record the hours worked each day for an employee. The number of rows for the 2-D array should be the same as the arrays above since each row corresponds to an employee. The number of columns represents days of the week (7 last I looked). Functions Needed In this lab, you must read in the employee names first because this identifies how many elements will be in each array. Then you wll have to read in the hourly pay rate for each employee. You must implement two functions: getEmployeeNames and getHourlyPay. /* TODO: Create a function called getEmployeeNames Arguments : Name array(first and last name) Maximum size Return the number of names filled. /* TODO: Create a function called getHourlyPay Arguments NameArray(filled in Step 1) HourlyPayArray(starts empty) NumberOfNamesFilled(from Stepl) Two additional functions: getHighestPay and getlowestPay should use the gross pay array and the number of employees to figure out the highest and lowest pay respectively. HINT: return the index of the highest/lowest gross pay so that when you print the answer in main, you can access the gross pay and the name. Nested Loops for Daily Hours Once you have the names and hourly pay rate, you have to ask the user to enter the hours worked for each employee. Expect to read in hours for 7 days, the user will put 0 for days off. This will require a loop that traverses the rows (each employee) and another loop that traverses the hours worked for the days. Another set of nested loops are needed to sum up the daily hours into total hours worked and use that to calculate the gross pay for the week. Once you have this, you now have everything you need to create the table which contains only the employee names, the total hours worked and the gross pay (no need to traverse the 2-D array again!). Program Output Employee Name, Total Hours and Gross Pay. Use the output information below to space your output in the three arrays that will be printed: employee names, total hours and gross pay. cout <« setw (20) << "Employee Name" << setw (7) << " Hours" << setw (10) << "Gross Pay" << endl; Again, you are working with parallel arrays, so use the same index to access elements in each array for an employee. Employee Name, Total Hours and Gross Pay. Finally, use the funictions that you wrote to calculate the highest and lowest gross pay and print this info according to the test case output. These functions only need the gross pay array and the number of elements in it. Each function should return the index of the target value in the gross pay array.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter10: Pointers
Section10.3: Pointer Arithmetic
Problem 4E
Related questions
Question
100%
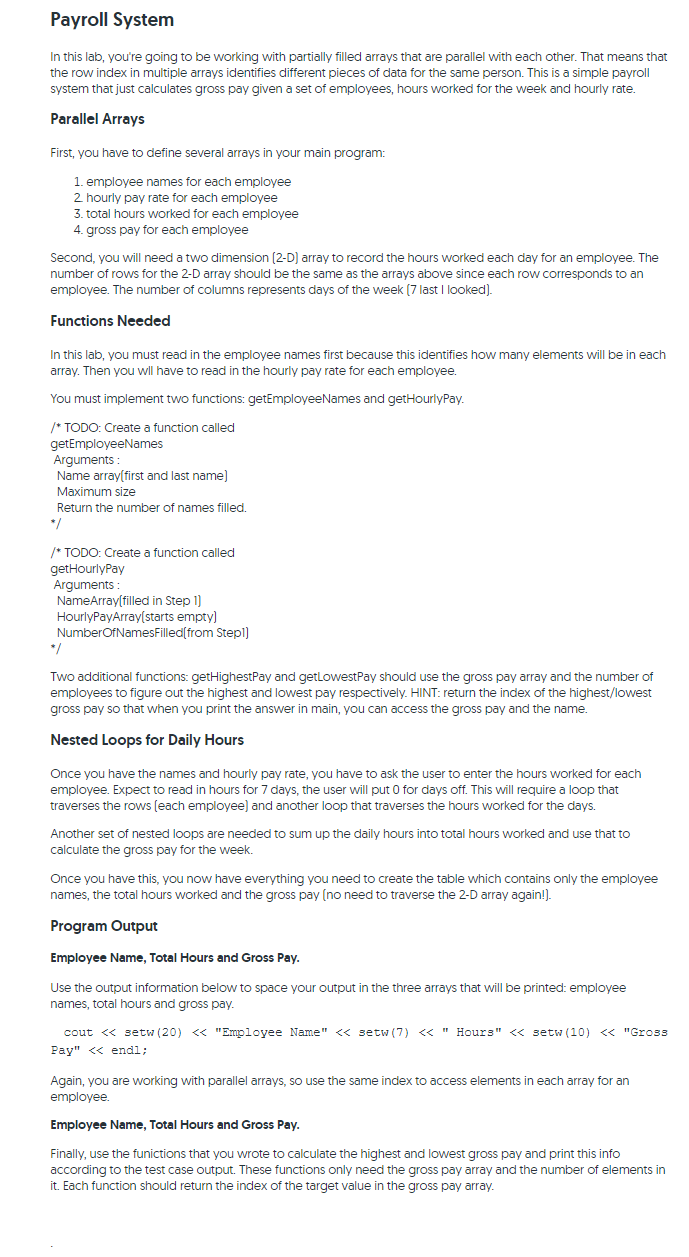
Transcribed Image Text:Payroll System
In this lab, you're going to be working with partially filled arrays that are parallel with each other. That means that
the row index in multiple arrays identifies different pieces of data for the same person. This is a simple payroll
system that just calculates gross pay given a set of employees, hours worked for the week and hourly rate.
Parallel Arrays
First, you have to define several arrays in your main program:
1. employee names for each employee
2 hourly pay rate for each employee
3. total hours worked for each employee
4. gross pay for each employee
Second, you will need a two dimension (2-D) array to record the hours worked each day for an employee. The
number of rows for the 2-D array should be the same as the arrays above since each row corresponds to an
employee. The number of columns represents days of the week (7 last I looked).
Functions Needed
In this lab, you must read in the employee names first because this identifies how many elements will be in each
array. Then you wll have to read in the hourly pay rate for each employee.
You must implement two functions: getEmployeeNames and getHourlyPay.
/* TODO: Create a function called
getEmployeeNames
Arguments :
Name array(first and last name)
Maximum size
Return the number of names filled.
/* TODO: Create a function called
getHourlyPay
Arguments
NameArray(filled in Step 1)
HourlyPayArray(starts empty)
NumberOfNamesFilled(from Stepl)
Two additional functions: getHighestPay and getlowestPay should use the gross pay array and the number of
employees to figure out the highest and lowest pay respectively. HINT: return the index of the highest/lowest
gross pay so that when you print the answer in main, you can access the gross pay and the name.
Nested Loops for Daily Hours
Once you have the names and hourly pay rate, you have to ask the user to enter the hours worked for each
employee. Expect to read in hours for 7 days, the user will put 0 for days off. This will require a loop that
traverses the rows (each employee) and another loop that traverses the hours worked for the days.
Another set of nested loops are needed to sum up the daily hours into total hours worked and use that to
calculate the gross pay for the week.
Once you have this, you now have everything you need to create the table which contains only the employee
names, the total hours worked and the gross pay (no need to traverse the 2-D array again!).
Program Output
Employee Name, Total Hours and Gross Pay.
Use the output information below to space your output in the three arrays that will be printed: employee
names, total hours and gross pay.
cout <« setw (20) << "Employee Name" << setw (7) << " Hours" << setw (10) << "Gross
Pay" << endl;
Again, you are working with parallel arrays, so use the same index to access elements in each array for an
employee.
Employee Name, Total Hours and Gross Pay.
Finally, use the funictions that you wrote to calculate the highest and lowest gross pay and print this info
according to the test case output. These functions only need the gross pay array and the number of elements in
it. Each function should return the index of the target value in the gross pay array.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Recommended textbooks for you
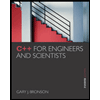
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
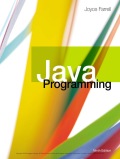
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
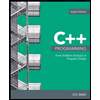
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
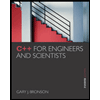
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
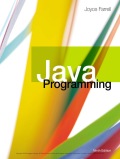
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
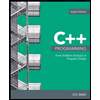
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
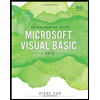
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
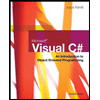
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,