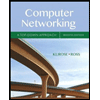
Exercise 2
This exercise is designed to assess student’s skills of writing programs using arrays and loops.
Write a C++ program using array and loop that prompts a user to enter 15 Students’ marks in Program
Design and Implementation (PDI) Module in an array. The program sorts the students’ marks in descending order using a loop and displays the marks of all students as well as lowest, highest, average marks, and the class result based on the average module marks. The result criteria is given below:
Average is less than 40 = Poor Result
Average is between 40 to 49 = Fair Result
Average is less than 50 to 59= Good Result
Average is less than 60 to 69 = Very Good Result
Average is between 70 to 79 = Excellent Result
Average is 80 or greater = Outstanding Result
Note: You must demonstrate the use of arrays and loops. Your code must be properly commented
Sample Output:
Welcome to Result Management System
Enter PDI Marks of Student 1: 50
Enter PDI Marks of Student 2: 35
Enter PDI Marks of Student 3: 30
Enter PDI Marks of Student 4: 65
Enter PDI Marks of Student 5: 70
Enter PDI Marks of Student 6: 73
Enter PDI Marks of Student 7: 55
Enter PDI Marks of Student 8: 48
Enter PDI Marks of Student 9: 20
Enter PDI Marks of Student 10: 45
Enter PDI Marks of Student 11: 75
Enter PDI Marks of Student 12: 35
Enter PDI Marks of Student 13: 45
Enter PDI Marks of Student 14: 24
Enter PDI Marks of Student 15: 41
75, 73, 70, 65, 55, 50, 48, 45, 45, 41, 35, 35, 30, 24, 20
All Students’ Marks in PDI (Highest to Lowest)
Lowest Marks: 20
Highest Marks: 75
Average Marks: 47.4
Fair Result
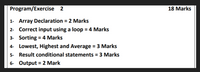

Step by stepSolved in 2 steps with 2 images

- I asked this once before but it came up incorrect somehow so I'll submit this one again. Write a program in C# named ArrayDemo that stores an array of 10 integers. (Note that the array is created for you and does not need to be changed.) Until the user enters a sentinel value, allow the user four options: (1) to view the list in order from the first to last position in the stored array (2) to view the list in order from the last to first position (3) to choose a specific position to view (4) to quit the application. -------- using System; using static System.Console; class ArrayDemo { static void Main() { int[] nums = {7, 6, 3, 2, 10, 8, 4, 5, 9, 1}; // Write your main here } }arrow_forwardIn C/C++, True or False: A function that changes the value of a variable passed by reference also changes the value of the original variable. A variable's type helps define the amount of memory it takes to hold a value of that type.arrow_forwardC# application that will allow users to enter values store all the expenses on that month in an array clothes, food, cool drinks, water, meatarrow_forward
- Please submit a flowchart of your program for your project below. Need a class which will contain: Student Name Student Id Student Grades (an array of 3 grades) A constructor that clears the student data (use -1 for unset grades) Get functions for items a, b, and c, average, and letter grade Set functions for items a, n, and c Note that the get and set functions for Student grades need an argument for the grade index. Need another class which will contain: An Array of Students (1 above) A count of number of students in use You need to create a menu interface that allows you to: Add new students Enter test grades Display all the students with their names, ids, test grades, average, and letter grade Exit the program Add comments and use proper indentation. Nice Features: I would like that system to accept a student with no grades, then later add one or more grades, and when all grades are entered, calculate the final average or grade. I would like the system to display the…arrow_forwardPlease written by computer source (java)arrow_forwardIn this lab, you use what you have learned about searching an array to find an exact match to complete a partially prewritten C++ program. The program uses an array that contains valid names for 10 cities in Michigan. You ask the user to enter a city name; your program then searches the array for that city name. If it is not found, the program should print a message that informs the user the city name is not found in the list of valid cities in Michigan. The file provided for this lab includes the input statements and the necessary variable declarations. You need to use a loop to examine all the items in the array and test for a match. You also need to set a flag if there is a match and then test the flag variable to determine if you should print the the Not a city in Michigan. message. Comments in the code tell you where to write your statements. You can use the previous Mail Order program as a guide. Instructions Ensure the provided code file named MichiganCities.cpp is open. Study…arrow_forward
- 8. Grade Book A teacher has five students who have taken four tests. The teacher uses the following grading scale to assign a letter grade to a student, based on the average of his or her four test scores: Test Score Letter Grade 90–100 A 80-89 B 70-79 C 60-69 D 0-59 Write a class that uses a String array or an ArrayList object to hold the five students' names, an array of five characters to hold the five students' letter grades, and five arrays of four doubles each to hold each student's set of test scores. The class should have methods that return a specific student's name, the average test score, and a letter grade based on the average. Demonstrate the class in a program that allows the user to enter each student's name and his or her four test scores. It should then display each student's average test score and letter grade. Input Validation: Do not accept test scores less than zero or greater than 100.arrow_forwardSee attached images. Please help - C++arrow_forwardc++ code Screenshot and code is mustarrow_forward
- Consider the following program specification: Write a program that will prompt the user for 10 integer values and stores them in an array. The values entered by the user only be between 0 and 100 and the program should validate these appropriately. The program should then: Display the average of the values Display the highest value in the array Display the lowest value in the array Discuss how the values should be validated. What control structures could be used? Using eclipsearrow_forwardc++ language using just one for looparrow_forwardComputer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
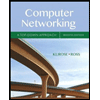
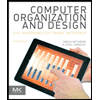
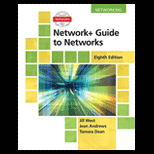
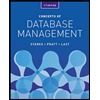
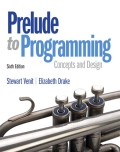
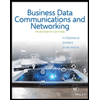