Phase 1: Start by creating a new project in PyCharms and adding a file called final.py In that file add the header information as we do for all assignments as described here: Submission Standards (of course the assignment is Final and include your own information) Then use print and input statements to write a program that will produce the following output: Welcome to the Calculon 5000 What is the path to the bill? C:\Users\0757557\PycharmProjects\GradingCS31\Final\data.txt The path you entered is C:\Users\0757557\PycharmProjects\GradingCS31\Final\data.txt ------------------------------------------------------------------------------------------------------------------------------------ data.txt : prices grapes: 4.00 oranges: 7.50 bread: 3.05 coke: 7.77 beef: 3.75 quantities grapes: 1 bread: 2 coke: 8 beef: 1 ------------------------------------------------------------------------------------------------------------------------------------ Please submit the answer in 'phases' it helps me with understanding what is happening :-) the top one is phase one and the pictures show the rest of the phases of the program after.
Phase 1:
Start by creating a new project in PyCharms and adding a file called final.py
In that file add the header information as we do for all assignments as described here: Submission Standards (of course the assignment is Final and include your own information)
Then use print and input statements to write a program that will produce the following output:
Welcome to the Calculon 5000
What is the path to the bill? C:\Users\0757557\PycharmProjects\GradingCS31\Final\data.txt
The path you entered is C:\Users\0757557\PycharmProjects\GradingCS31\Final\data.txt
------------------------------------------------------------------------------------------------------------------------------------
data.txt :
grapes: 4.00
oranges: 7.50
bread: 3.05
coke: 7.77
beef: 3.75
quantities
grapes: 1
bread: 2
coke: 8
beef: 1



Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 4 images

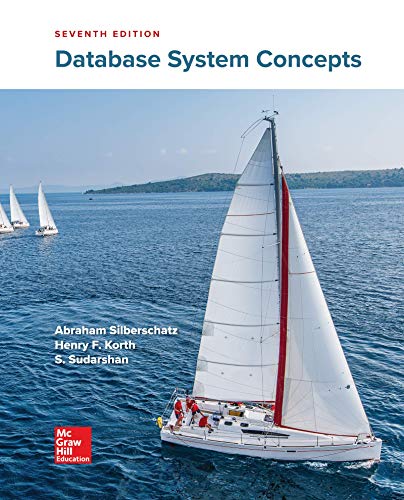
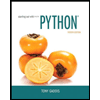
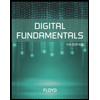
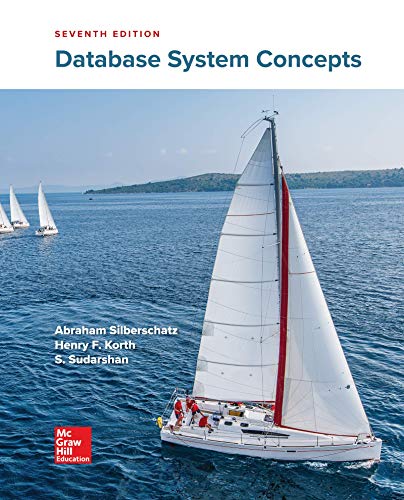
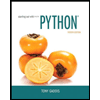
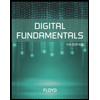
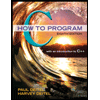
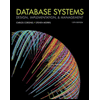
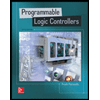