Please answer in Java, thank you. The purpose of this assignment is to practice your knowledge of how a linked data structure is implemented. This is a more advanced version of a linked list, and will help to give you practice in how to deal with data structures connected through references. This assignment is very important to help you understand the fundamentals of Linked Lists. At the end of this assignment, you should be able to fully understand how any variation of a Linked List is implemented. Instructions: For this project you will be implementing a new data structure called LinkedGrid. This is a linked type data structure version of a 2D array. The LinkedGrid can support any combination of dimensions (n x n, n x m, or m x n, where n > m) This data structure has a singly linked system which allows you to traverse the grid in multiple directions in addition to allowing you to retrieve specific rows and columns. The following diagram gives a rough visual of this data structure. NOTE: Your LinkedGrid MUST work with any number of nodes, the picture only shows twelve as an idea. You may not hardcode only twelve nodes... Complete ALL methods with "TO DO" in LinkedGrid.java import java.util.ArrayList; public class LinkedGrid{ private int numRows; private int numCols; private GridNode head; private GridNode lastRowHead; private GridNode lastColHead; public LinkedGrid() {} /* A constructor which takes the initial number of rows and columns of the LinkedGrid. This constructor needs to initialize the LinkedGrid with grid nodes (which are initially empty) Example: if I invoked the constructor with new LinkedGrid(2, 3), then I would have to initialize my LinkedGrid of 6 empty nodes with 2 rows and 3 nodes per row */ public LinkedGrid(int numRows, int numCols) { // TO DO } /* Constructor which takes a normal 2D array as an argument. This is the ONLY place you can use a 2D array and this 2D array is only used to initialize the values of your LinkedGrid object */ public LinkedGrid(E[][] elements) { // TO DO } /* Get the number of rows */ public int getNumRows() { return numRows; } /* Get the number of columns */ public int getNumCols() { return numCols; } /* Returns the item at the given (row, col). NOTE: You must return the item stored in the GridNode, NOT the GridNode itself */ public E get(int row, int col) { // TO DO return null; } /* Assigns the given item to the GridNode at position (row, col) */ public void set(int row, int col, E value) { // TO DO } /* Get row at index. NOTE: This is not an arraylist of nodes, but of the values in the nodes. */ public ArrayList getRow(int index) { // TO DO return null; } /* Get column at index. NOTE: This is not an arraylist of nodes, but of the values in the nodes. */ public ArrayList getCol(int index) { // TO DO return null; } /* Adds a new row of empty nodes to the beginning of the list */ public void addFirstRow() { // TO DO } /* Adds a new column of empty nodes to the beginning of the list */ public void addFirstCol() { // TO DO } /* Adds a new row of empty nodes to the end of the list */ public void addLastRow() { // TO DO } /* Adds a new column of empty nodes to the end of the list */ public void addLastCol() { // TO DO } /* Inserts a row at the given index. (Insert here means the rows shift over by 1 from the insertion point onward) */ public void insertRow(int index) { // TO DO } /* Inserts a column at the given index. (Insert here means the columns shift over by 1 from the insertion point onward) */ public void insertCol(int index) { // TO DO } /* Removes the first row */ public void deleteFirstRow() { // TO DO } /* Removes the first column */ public void deleteFirstCol() { // TO DO } /* Removes the last row */ public void deleteLastRow() { // TO DO } /* Removes the last column */ public void deleteLastCol() { // TO DO } /* Removes the row at the given index */ public void deleteRow(int index) { // TO DO } /* Removes the column at the given index */ public void deleteCol(int index) { // TO DO } void display() { if (head == null) { System.out.println("This instance of LinkedGrid is empty."); return; } GridNode currRow = head; while(currRow != null) { GridNode curr = currRow; while(curr != null) { System.out.printf("%5s ", curr.getElement().toString()); curr = curr.nextCol; } System.out.println(); currRow = currRow.nextRow; } } }
Please answer in Java, thank you.
The purpose of this assignment is to practice your knowledge of how a linked data structure is implemented. This is a more advanced version of a linked list, and will help to give you practice in how to deal with data structures connected through references. This assignment is very important to help you understand the fundamentals of Linked Lists. At the end of this assignment, you should be able to fully understand how any variation of a Linked List is implemented.
Instructions:
For this project you will be implementing a new data structure called LinkedGrid. This is a linked type data structure version of a 2D array. The LinkedGrid can support any combination of dimensions (n x n, n x m, or m x n, where n > m) This data structure has a singly linked system which allows you to traverse the grid in multiple directions in addition to allowing you to retrieve specific rows and columns. The following diagram gives a rough visual of this data structure. NOTE: Your LinkedGrid MUST work with any number of nodes, the picture only shows twelve as an idea. You may not hardcode only twelve nodes...
Complete ALL methods with "TO DO" in LinkedGrid.java
import java.util.ArrayList;
public class LinkedGrid<E>{
private int numRows;
private int numCols;
private GridNode<E> head;
private GridNode<E> lastRowHead;
private GridNode<E> lastColHead;
public LinkedGrid() {}
/*
A constructor which takes the initial number of rows and columns of
the LinkedGrid. This constructor needs to initialize the LinkedGrid with
grid nodes (which are initially empty)
Example: if I invoked the constructor with new LinkedGrid(2, 3), then I
would have to initialize my LinkedGrid of 6 empty nodes with 2 rows and
3 nodes per row
*/
public LinkedGrid(int numRows, int numCols) {
// TO DO
}
/*
Constructor which takes a normal 2D array as an argument. This is the
ONLY place you can use a 2D array and this 2D array is only used to
initialize the values of your LinkedGrid object
*/
public LinkedGrid(E[][] elements) {
// TO DO
}
/*
Get the number of rows
*/
public int getNumRows() {
return numRows;
}
/*
Get the number of columns
*/
public int getNumCols() {
return numCols;
}
/*
Returns the item at the given (row, col). NOTE: You must return the
item stored in the GridNode, NOT the GridNode itself
*/
public E get(int row, int col) {
// TO DO
return null;
}
/*
Assigns the given item to the GridNode at position (row, col)
*/
public void set(int row, int col, E value) {
// TO DO
}
/*
Get row at index. NOTE: This is not an arraylist of nodes, but of
the values in the nodes.
*/
public ArrayList<E> getRow(int index) {
// TO DO
return null;
}
/*
Get column at index. NOTE: This is not an arraylist of nodes, but of
the values in the nodes.
*/
public ArrayList<E> getCol(int index) {
// TO DO
return null;
}
/*
Adds a new row of empty nodes to the beginning of the list
*/
public void addFirstRow() {
// TO DO
}
/*
Adds a new column of empty nodes to the beginning of the list
*/
public void addFirstCol() {
// TO DO
}
/*
Adds a new row of empty nodes to the end of the list
*/
public void addLastRow() {
// TO DO
}
/*
Adds a new column of empty nodes to the end of the list
*/
public void addLastCol() {
// TO DO
}
/*
Inserts a row at the given index. (Insert here means the rows
shift over by 1 from the insertion point onward)
*/
public void insertRow(int index) {
// TO DO
}
/*
Inserts a column at the given index. (Insert here means the columns
shift over by 1 from the insertion point onward)
*/
public void insertCol(int index) {
// TO DO
}
/*
Removes the first row
*/
public void deleteFirstRow() {
// TO DO
}
/*
Removes the first column
*/
public void deleteFirstCol() {
// TO DO
}
/*
Removes the last row
*/
public void deleteLastRow() {
// TO DO
}
/*
Removes the last column
*/
public void deleteLastCol() {
// TO DO
}
/*
Removes the row at the given index
*/
public void deleteRow(int index) {
// TO DO
}
/*
Removes the column at the given index
*/
public void deleteCol(int index) {
// TO DO
}
void display() {
if (head == null) {
System.out.println("This instance of LinkedGrid is empty.");
return;
}
GridNode currRow = head;
while(currRow != null) {
GridNode curr = currRow;
while(curr != null) {
System.out.printf("%5s ", curr.getElement().toString());
curr = curr.nextCol;
}
System.out.println();
currRow = currRow.nextRow;
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

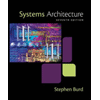
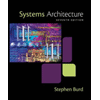