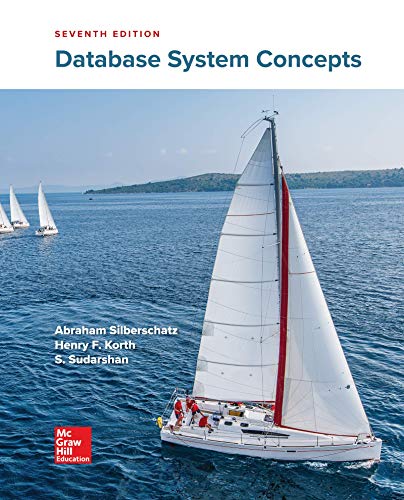
I'm getting error when compiling this code for my rolling dice assignment
Please fix this Java code so it will run successfully.
package dicerollinggame;
import java.util.Scanner;
class SingleDie {
private int sides;
public SingleDie(int sides) {
this.sides = sides;
}
public int roll() {
return (int) (Math.random() * sides) + 1;
}
}
class PairDice {
private SingleDie die1;
private SingleDie die2;
public PairDice(int sides) {
die1 = new SingleDie(sides);
die2 = new SingleDie(sides);
}
public int rollDice() {
int result1 = die1.roll();
int result2 = die2.roll();
return result1 + result2;
}
public boolean isSpecialCombination(int sum) {
return sum == 2 || sum == 7 || sum == 12;
}
}
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Welcome to the Dice Rolling Game!");
int sides;
do {
System.out.print("Enter the number of sides for each die: ");
sides = sc.nextInt();
if (sides <= 1) {
System.out.println("Please enter a valid number of sides for the dice.");
}
} while (sides <= 1);
PairDice dicePair = new PairDice(sides);
boolean playAgain = true;
int gamesWon = 0;
int gamesLost = 0;
while (playAgain) {
int result = dicePair.rollDice();
System.out.println("Result: " + result);
if (dicePair.isSpecialCombination(result)) {
if (result == 2) {
System.out.println("1 + 1 = 2 snake eyes!");
} else if (result == 7) {
System.out.println("7 craps!");
} else if (result == 12) {
System.out.println("6 + 6 = 12 box cars!");
}
playAgain = false;
gamesLost++;
System.out.println("You lost! Games won: " + gamesWon + ", Games lost: " + gamesLost);
} else if (result == 7 || result == 11) {
playAgain = false;
gamesWon++;
System.out.println("You won! Games won: " + gamesWon + ", Games lost: " + gamesLost);
} else {
System.out.println("Roll again!");
}
}
sc.close();
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 5 images

- this does not work in Eclipse Java, please you can fix it? please see below: import java.util.Scanner; public class Assig4 { //email using name and last name public static String generateEmail(String fname, String lname) { String email = fname.substring(0, 1).toLowerCase() + "." + lname.toLowerCase() + "@kean.edu"; return email; } //password using first name, security answer and birth year public static String generatePassword(String firstname, String answer, String birthyear) { String password = firstname.substring(0, 3).toUpperCase() + "" + birthyear + "" + answer.substring(0, 3).toUpperCase(); return password; } public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter first name: "); String firstname = sc.nextLine(); System.out.print("Enter last name: "); String lastname…arrow_forwardPlease help me with the errors I am e having the program below. There are two parts of the program. One is an encryption and decryption java code and the other is the gui for that program Encryption and decryption java code is below import java.util.*; public class Main { public static boolean isALetter(char letter){ if( (letter >= 'a' && letter = 'A' && letter =-32767 && encryptKeyarrow_forwardWhy does the Number Class from this slide need to be abstract?arrow_forward
- Write code that outputs variable numDays as follows. End with a newline. Ex: If the input is: the output is: Days: 3 1 import java.util.Scanner; 2 3 public class OutputTest { public static void main (String [] args) { int numDays; 4 6. // Our tests will run your program with input 3, then run again with input 6. // Your program should work for any input, though. Scanner scnr = new Scanner(System.in); numDays 7 8 9. 10 scnr.nextInt(); 11 12 /* Your code goes here */ 13 } 15 } 14arrow_forwardThis is my program. I need help with the math. import java.util.*;import javax.swing.JOptionPane;public class Craps { public static void main(String[] args) { int Bet, Die; int WinC = 0, LossC = 0, GameC = 0, GameN = 1; double startingBal = 0, curBal = 0; double balD, balDe, balDec, balI, balIn, balInc; char Answer = '\0'; Scanner crapsGame = new Scanner(System.in); System.out.println("Welcome to the Craps Game"); curBal = StartingBal(startingBal); System.out.println("Your starting balance is: $" + curBal); do { System.out.println("Please input your bet for the game>> "); Bet = crapsGame.nextInt(); System.out.println("Game #" + GameN + " Starting with bet of: $" + Bet ); if (Bet < 1 || Bet > curBal) { System.out.println("Invalid bet – Your balance is only: $" + curBal); while (Bet < 1 || Bet > curBal)…arrow_forwardPlease help me comment these lines of code. Please not these are just some lines of code that have been taken from the java program I am creating which is a coin toss program. I do not need you to create one. Just comment what the lines of code below private static String sideUp; } public void toss() { Random in = new Random(); int x = in.nextInt(); if (x % 2 == 0) sideUp = "Heads"; else sideUp = "Tails"; } public static void main(String[] args) { int tail = 0, head = 0; System.out.println("\nTossing coin 20 times:-"); for (int i = 1; i <= 20; i++) { coin.toss(); if (coin.getFace().equals("tails")) tail++; else head++;arrow_forward
- StringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardThere are a few errors in this java code: can you fix it please, its basically a debugging exercise: public static int sum(int n){ int n; for (int i=1; i<=n;i--){ sum++; } return sum; } }arrow_forwardCan you do this please? Thank youarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
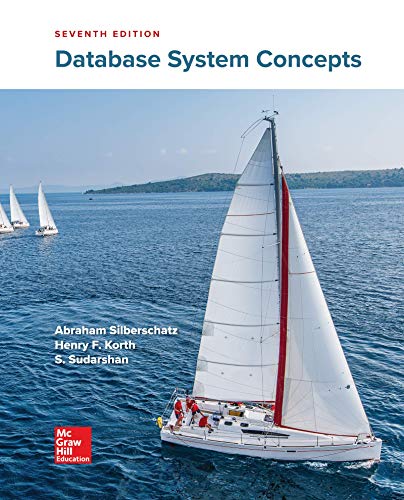
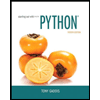
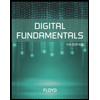
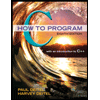
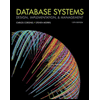
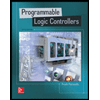