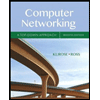
Need help with this Java review
If you can also send a screenshot will be helpful to understan
Objective: The purpose of this lab exercise is to create a Singly Linked List data structure . Please note that the underlying implementation should follow with the descriptions listed below.
Instructions : Create the following Linked List Data Structure in your single package and use "for loops" for your repetitive tasks.
Task Check List
- ONLY "for" loops should be used within the data structure class.
- Names of identifiers MUST match the names listed in the description below. Deductions otherwise.
Description
The internal structure of this Linked List is a singly linked Node data structure and should have at a minimum the following specifications:
data fields: The data fields to declare are private and you will keep track of the size of the list with the variable size and the start of the list with the reference variable data.
- first is a reference variable for the first Node in the list.
- size keeps track of the number of nodes in the list of type int. This will allow you to know the current size of the list without having to traversing the list.
constructors: initializes the data fields size and data.
- A constructor that is a default constructor initializes the starting node location "first" and size to a zero equivalent, that is, constructs an empty list.
public SinglyLinkedList()
methods: manages the behavior of the linked nodes.
Together, the methods below give the illusion of an index or countable location. Implement these methods within your generic Linked List class.
Method |
Description |
Header |
add(item) | uses the append method. This method returns true, if the data was added successfully. |
public boolean add(T item) |
add(index, item) | inserts elements at a given location in the list, shifting subsequent elements to the right. |
public void add(int index, T item) |
append(item) | appends elements to the end of the list. This is a private helper method. |
public void append(T item) |
checkIndex(index) | checks if the given index is valid. Throws an IndexOutOfBoundsException, if invalid. This is a private helper method. |
private void checkIndex(int index) |
detach(index) | detaches the node at the specified index from list. This is a private helper method. |
private T detach(int index) |
get(index) | returns the item at the specified position in the list. This method first checks if the index requested is valid. |
public T get(int index) |
insertBefore(index, item) | inserts an item before the non-null node at the specified index in the list. This is a private helper method. |
private void insertBefore(int index, T item) |
isEmpty() |
returns true, if the list is empty, i.e., the list contains no elements. |
public boolean isEmpty() |
node(index) | returns a reference to the node at the given position in the list. This is a private helper method. |
private Node node(int index) |
remove(index) | removes the item at the given position in the list. Shifts subsequent elements to the left and returns the item removed. |
public T remove(int index) |
set(index, item) | replaces the item at the specified position with the one passed. This method checks if the index requested is valid before it does the replacement and returns the replaced item. |
public T set(int index, T item) |
size() |
returns the number of elements in the list. |
public int size() |
toString() | displays the contents of the list. |
public String toString() |

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- Dont use others answers please!! Write the individual code! follow the directions on the photos will leave you feedback!! Thank you!arrow_forwardIn this task you will work with the linked list of digits we have created in the lessons up to this point. As before you are provided with some code that you should not modify: A structure definition for the storage of each digit's information. A main() function to test your code. The functions createDigit(), append(), printNumber(), freeNumber(), readNumber() and divisibleByThree() (although you may not need to use all of these). Your task is to write a new function changeThrees() which takes as input a pointer that holds the address of the start of a linked list of digits. Your function should change all of those digits in this linked list that equal 3 to the digit 9, and count how many replacements were made. The function should return this number of replacements. Provided codearrow_forwardPlease just use main.cpp,sequence.cpp and sequence.h. It has provided the sample insert()function. No documentation (commenting) is required for this assignment. This assignment is based on an assignment from "Data Structures and Other Objects Using C++" by Michael Main and Walter Savitch. Use linked lists to implement a Sequence class that stores int values. Specification The specification of the class is below. There are 9 member functions (not counting the big 3) and 2 types to define. The idea behind this class is that there will be an internal iterator, i.e., an iterator that the client cannot access, but that the class itself manages. For example, if we have a Sequence object named s, we would use s.start() to set the iterator to the beginning of the list, and s.advance() to move the iterator to the next node in the list. (I'm making the analogy to iterators as a way to help you understand the point of what we are doing. If trying to think in terms of iterators is confusing,…arrow_forward
- I don't get the "//To be implemented" comments, what am I supposed to do?Also, are the header files and header files implementation different? Like is the linkedList.h different than the linkedList.h (implementation)?arrow_forwardHello thank you for the solution. Is there also a way to format the list without using the .join method, and just using f' strings, perhaps in a list comprehension?arrow_forwardWe wish to implement a system that allows the management of Employees and Students. As a first step. you need to implement the above UML diagrams Notes: . ZOOM . PERSON, EMPLOYEE and STUDENT should be public static constants The no-arg constructor will initialize ID, firstName and lastName to their respective null values. It will set personType to PERSON, and sortorder to LAST_NAME The overloaded constructor will assign each parameter to its respective field. sortorder will also be set to LAST_NAME toString() will output type, ID, firstName and lastName, all tab separated. This is an override method equals() will compare if the current object and the parameter contain the same values. This is an override method compareTo() compares the current object and the parameter. This is an override method. It will be used to sort objects Needs the Comparable interface O Will only compare fields depending on the sortorder. For example, if the sortorder is TYPE, it should then compare the…arrow_forward
- Please,help me by providing C++ programing solution. Do not use the LinkedList class or any classes that offers list functions. Implement a LinkList in C++. Pease,implement with an ItemType class and a NodeType structThe program should read a data file,and the data file has two lines of data as follow:100, 110, 120, 130, 140, 150, 160100, 130, 160The program will first add all of the numbers from the file,then display all of them,then delete.arrow_forwardYou will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forwardin this assignment i have to remove vowels from a string using a linked list. the linked list and link code is from a textbook and cannot be changed if the code alters the data structure. for some reason when i implement this code it gives me a logical error where the program only removes all instances of the first vowel in a string instead of moving through all vowels of the string and removing each one with all instances. i would appreciate if you could tell me the problem and solution in words and not in code. The code is in java. output please enter a string.researchhcraeserfalseList (first -->last): researchList (first -->last): researchList (first -->last): rsarch CODE MAIN FUNCTION import java.util.Scanner;/*** Write a description of class test here.** @author (your name)* @version (a version number or a date)*/public class test{// instance variables - replace the example below with your ownpublic static void main(String[] args){Scanner input = new…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
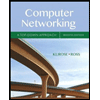
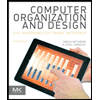
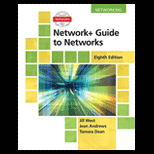
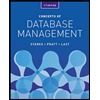
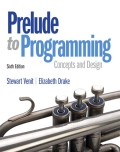
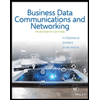