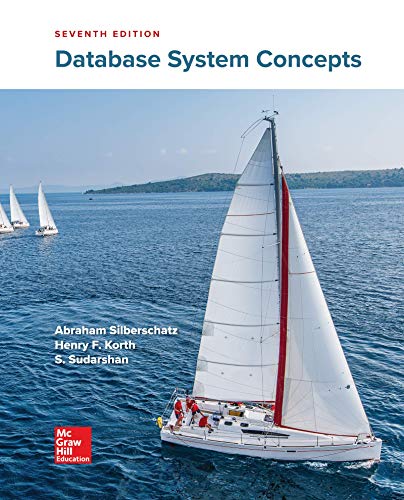
Concept explainers
Please help with the following:
Code in Java keep the code as simple as possible do not use arrays nor parseItnt StringBuilder etc keep it very simple.
Class pizza
This class consists of the following fields.
1. String name
2. double price
3. String topping1
4. String topping2
5. String topping3
In the constructor, you will ask for four variables: String name, String topping1, String topping2, String topping3. These fields will be sat immediately using the proper setter methods. Also, in the constructor, another method will be called. Namely, the private void setPrice() method.
The setPrice method, will check if any of the toppings is equals to the empty string ”” and set the price of the pizza depending on the count of the none empty fields according to the following:
no toppings the price will be sat to 10$.
With 1 topping the price will be 15$
With 2 topping the price will be 17.5$
With 3 topping the price will be 22$
All fields have to be private thus they have the proper setter and getter methods for each.
Class Person
Each person has the following fields:
private String name
private double age
private Pizza pizza
Here, you will only set the name and the age of the person using the constructor. The class should have proper setters and getters for each of the fields. However, you will need to set the values using the this.keyword expression.
This class also has a method called order(). Order is a public method that is responsible for initializing the pizza the person orders. This method works the following way.
When the order method is called, a message will be displayed on the screen asking the person to order a pizza by asking them to input the name of the pizza they want together the name of the three toppings they wish to have on their pizza. These will be stored in four variables. Then a new pizza object in the method will be created using the elements that the user inputted via the scanner. Keep in mind this all happens in the Person class.
However, you keep the field in the global variables. And just do the memory allocation within the method. Like this:
In the global fields you still need to define : Pizza pizza;
then
public void order(){ do a bunch of stuff scanner input....
pizza=new pizza...... do a bunch of stuff
}
There is another thing the order method should do too. All of the things that I described above should be repeated until the user inputs the string ”yes”. So, when the order method is called, the user will be asked to input what they want to eat. Then once the object has been created, the user is asked if they are sure that this is the pizza they want, and until they do not input ”yes”, they will be asked to input their toppings and the pizza object will be
reinitialized.
The main Class Main
Three people decide to go to a restaurant. They all sit around the same table. Initialize these people without using the scanner in the main method (manually the way you like). Then, for each of the people call the order method. Once the order method is called and terminated successfully. You have to print the pizza object that the person ordered. So, you will call person.getPizza() and then print the object. This means you have to have a toString method for the pizza class that displays the name of the pizza and its toppings as one string.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- Cell phone packages please answer the following question in javaarrow_forwardis defined as where a variable is accessible or can be used. Private Class Scope Private Method None of thesearrow_forward1. Dummy GUI Application by Codechum Admin A GUI Application is an application that has a user interface that the user can interact with. For this program, we will be simulating this behavior. First, implement another class called Checkbox which implements the Clickable interface which has only one method: public void click(). The Checkbox will have the following properties: private boolean isChecked (defaults to false upon the creation of object) private String text Additionally, it should have the following methods: the implementation of the click() method If the isChecked is currently false, this will set the isChecked to true and will then print the message "Checkbox is checked". If it is currently true, this will set the isChecked to false and will then print the message "Checkbox is unchecked". Note that the messages to be printed should have also print a new line at the end. an override of the toString() method which returns the message: "Checkbox ({text} - Clicked…arrow_forward
- When you instantiate an object from a class, you give a name to this particular object so that your program can distinguish it from other objects you instantiate from the same class. True Falsearrow_forwardLab 2 – Designing a class This lab requires you to think about the steps that take place in a program by writing pseudocode. Read the following program prior to completing the lab. Design a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field. For the programming problem, create the pseudocode that defines the class and enter it below. Enter pseudocode herearrow_forwardHelp me With this Java Taskarrow_forward
- 9arrow_forwardInstructions: IMPORTANT: This is a continuation of the previous part of the project and assumes that you are starting with code that fulfills all requirements from that part of the project. Modify the your code from the previous part of the project to make it modular. In addition to the main method, your code must include the following static methods: Method 1 - displayTitle A method that creates a String object in memory to hold the text “Computer Hardware Graphics Quality Recommendation Tool” and displays it Method 2 – getResolutionString A method that accepts an integer value (1, 2, 3, or 4) that denotes the monitor resolution. The method should return the appropriate String representation of the monitor resolution. For example, if the method is passed an integer value of 1, it should return a String with a value of “1280 x 720”. (See Step 4 of Project 1) Method 3 – getMultiplierValue A method that accepts an integer value (1, 2, 3, or 4) that denotes the monitor resolution and…arrow_forwarddef area(side1, side2): return side1 * side2s1 = 12s2 = 6Identify the statements that correctly call the area function. Select ALL that apply. Question options: area(s1,s2) answer = area(s1,s2) print(f'The area is {area(s1,s2)}') result = area(side1,side2)arrow_forward
- JAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #2. Word Counter (page 608) Write a method that accepts a String object as an argument and returns the number of words it contains. For instance, if the argument is “Four score and seven years ago” the method should return the number 6. Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The number of words in the string should be displayed on the screen. Test Case 1 Please enter a string or type QUIT to exit:\nHello World!ENTERThere are 2 words in that string.\nPlease enter a string or type QUIT to exit:\nI have a dream.ENTERThere are 4 words in that string.\nPlease enter a string or type QUIT to exit:\nJava is great.ENTERThere are 3 words in that string.\nPlease enter a string or type QUIT to exit:\nquitENTERarrow_forward1. RetailItem Class Write a class named Retailltem that holds data about an item in a retail store. The class should have the following fields: • description. It is a String object that holds a brief description of the item. • unitsOnHand. It is an int variable that holds the number of units currently in inventory. • price. It is a double that holds the item's retail price. Write appropriate mutator methods that store values in these fields and accessor methods that return the values in these fields. Once you have written the class, write a separate program that creates three RetailItem objects and stores the following data in them. Units On Hold Description Designer Jeans Jacket Price 40 34.95 12 59.95 Shirt 20 24.95arrow_forwardTrue or False _(1) The expression n/3*(8+4) evaluates to 16 if n=4 ___(2) x is greater than both y and z can be written as the statement x>y&&z ; in Java. ___(3) In Java a string is automatically an object.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
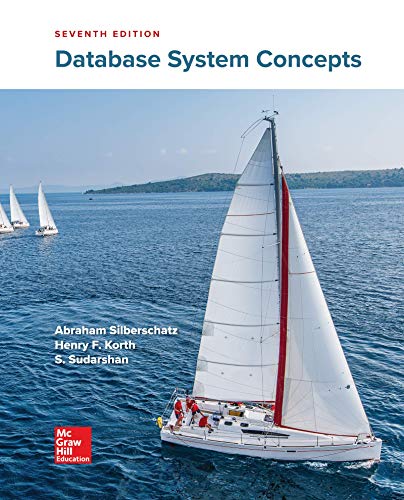
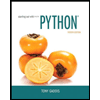
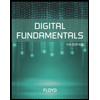
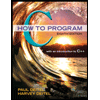
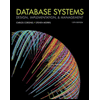
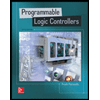