Please help with the following: Code in Java keep the code as simple as possible do not use arrays nor parseItnt StringBuilder etc keep it very simple. Class pizza This class consists of the following fields. String name double price String topping1 String topping2 String topping3 In the constructor, you will ask for four variables: String name, String topping1, String topping2, String topping3. These fields will be sat immediately using the proper setter methods. Also, in the constructor, another method will be called. Namely, the private void setPrice() method. The setPrice method, will check if any of the toppings is equals to the empty string ”” and set the price of the pizza depending on the count of the none empty fields according to the following: no toppings the price will be sat to 10$. With 1 topping the price will be 15$ With 2 topping the price will be 17.5$ With 3 topping the price will be 22$ All fields have to be private thus they have the proper setter and getter methods for each. Class Person Each person has the following fields: private String name private double age private Pizza pizza Here, you will only set the name and the age of the person using the constructor. The class should have proper setters and getters for each of the fields. However, you will need to set the values using the this.keyword expression. This class also has a method called order(). Order is a public method that is responsible for initializing the pizza the person orders. This method works the following way. When the order method is called, a message will be displayed on the screen asking the person to order a pizza by asking them to input the name of the pizza they want together the name of the three toppings they wish to have on their pizza. These will be stored in four variables. Then a new pizza object in the method will be created using the elements that the user inputted via the scanner. Keep in mind this all happens in the Person class. However, you keep the field in the global variables. And just do the memory allocation within the method. Like this: In the global fields you still need to define : Pizza pizza; then public void order(){ do a bunch of stuff scanner input.... pizza=new pizza...... do a bunch of stuff } There is another thing the order method should do too. All of the things that I described above should be repeated until the user inputs the string ”yes”. So, when the order method is called, the user will be asked to input what they want to eat. Then once the object has been created, the user is asked if they are sure that this is the pizza they want, and until they do not input ”yes”, they will be asked to input their toppings and the pizza object will be reinitialized. The main Class Main Three people decide to go to a restaurant. They all sit around the same table. Initialize these people without using the scanner in the main method (manually the way you like). Then, for each of the people call the order method. Once the order method is called and terminated successfully. You have to print the pizza object that the person ordered. So, you will call person.getPizza() and then print the object. This means you have to have a toString method for the pizza class that displays the name of the pizza and its toppings as one string.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Please help with the following:
Code in Java keep the code as simple as possible do not use arrays nor parseItnt StringBuilder etc keep it very simple.
Class pizza
This class consists of the following fields.
String name
double price
String topping1
String topping2
String topping3
In the constructor, you will ask for four variables: String name, String topping1, String topping2, String topping3. These fields will be sat immediately using the proper setter methods. Also, in the constructor, another method will be called. Namely, the private void setPrice() method.
The setPrice method, will check if any of the toppings is equals to the empty string ”” and set the price of the pizza depending on the count of the none empty fields according to the following:
no toppings the price will be sat to 10$.
With 1 topping the price will be 15$
With 2 topping the price will be 17.5$
With 3 topping the price will be 22$
All fields have to be private thus they have the proper setter and getter methods for each.
Class Person
Each person has the following fields:
private String name
private double age
private Pizza pizza
Here, you will only set the name and the age of the person using the constructor. The class should have proper setters and getters for each of the fields. However, you will need to set the values using the this.keyword expression.
This class also has a method called order(). Order is a public method that is responsible for initializing the pizza the person orders. This method works the following way.
When the order method is called, a message will be displayed on the screen asking the person to order a pizza by asking them to input the name of the pizza they want together the name of the three toppings they wish to have on their pizza. These will be stored in four variables. Then a new pizza object in the method will be created using the elements that the user inputted via the scanner. Keep in mind this all happens in the Person class.
However, you keep the field in the global variables. And just do the memory allocation within the method. Like this:
In the global fields you still need to define : Pizza pizza;
then
public void order(){ do a bunch of stuff scanner input....
pizza=new pizza...... do a bunch of stuff
}
There is another thing the order method should do too. All of the things that I described above should be repeated until the user inputs the string ”yes”. So, when the order method is called, the user will be asked to input what they want to eat. Then once the object has been created, the user is asked if they are sure that this is the pizza they want, and until they do not input ”yes”, they will be asked to input their toppings and the pizza object will be
reinitialized.
The main Class Main
Three people decide to go to a restaurant. They all sit around the same table. Initialize these people without using the scanner in the main method (manually the way you like). Then, for each of the people call the order method. Once the order method is called and terminated successfully. You have to print the pizza object that the person ordered. So, you will call person.getPizza() and then print the object. This means you have to have a toString method for the pizza class that displays the name of the pizza and its toppings as one string.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

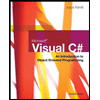
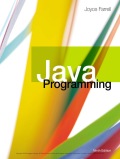
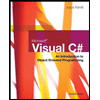
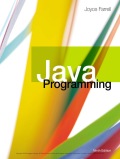