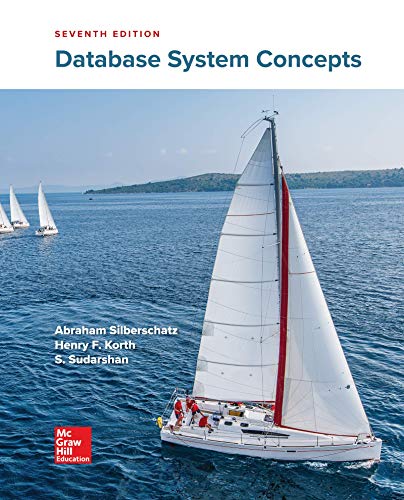
can you explain why the code shown below does not
output:
pineapple
lime
and how to fix it when using the driver
i also attach of the LLNode class
// Note: It is important to implement this file as a circular queue without 'front' pointer
public class CircularLinkedQueue<T> implements QueueInterface<T> {
protected LLNode<T> rear; // reference to the rear of this queue
protected int numElements = 0;
public CircularLinkedQueue() {
rear = null;
}
public void enqueue(T element) {
// Adds element to the rear of this queue.
LLNode<T> newNode = new LLNode<T>(element);
if (isEmpty()) {
rear = newNode;
newNode.setLink(newNode);
numElements++;
}
else {
rear = newNode;
newNode.setLink(rear.getLink());
rear.setLink(newNode);
numElements++;
}
}
public T dequeue() {
// Throws QueueUnderflowException if this queue is empty;
// otherwise, removes front element from this queue and returns it
if (isEmpty())
throw new QueueUnderflowException("Dequeue attempted on empty queue");
else {
T element;
rear = rear.getLink();
element = rear.getInfo();
rear.setInfo(null);
if (rear.getLink() == null)
rear = null;
numElements--;
return element;
}
}
public String toString() {
String result = "\n";
LLNode<T> cursor = rear;
while (cursor != null){
result+=(cursor.getInfo());
cursor = cursor.getLink();
}
return result;
}
public boolean isEmpty() {
// Returns true if this queue is empty; otherwise, returns false.
return (numElements == 0);
}
public boolean isFull() {
// Returns false - a linked queue is never full.
return false;
}
public int size() {
// Returns the number of elements in this queue.
return numElements;
}
}
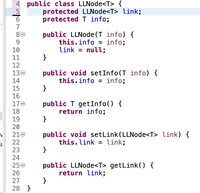
![public class Driver {
public static void main(String[] args) {
CircularLinkedQueue<String> cq = new CircularLinkedQueue<String>();
mit
try {
System.out.println(cq.dequeue());
} catch (Exception e) {
menm
ww vm
wwwww
}
cq.enqueue("Tomato");
cg.enqueue("Grape");
cg.dequeue();
cg.dequeue();
cg.engueue("Pineapple");
cg.enqueue("Lime");
System.out.printIn(ca);
}](https://content.bartleby.com/qna-images/question/1b2c1e36-9974-49eb-8a0c-fe4d3c635f06/bebb7c5b-b032-4ca8-8919-a966cfe365ad/w28nf34_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- Assume class MyStack implements the following StackGen interface. For this question, make no assumptions about the implementation of MyStack except that the following interface methods are implemented and work as documented. Write a public instance method for MyStack, called interchange(T element) to replace the bottom "two" items in the stack with element. If there are fewer than two items on the stack, upon return the stack should contain exactly two items that are element.arrow_forwardImplement a simple linked list in Python (Write source code and show output) with basic linked list operations like: (a) create a sequence of nodes and construct a linear linked list. (b) insert a new node in the linked list. (b) delete a particular node in the linked list. (c) modify the linear linked list into a circular linked list. Use this template: class Node:def __init__(self, val=None):self.val = valself.next = Noneclass LinkedList:"""TODO: Remove the "pass" statements and implement each methodAdd any methods if necessaryDON'T use a builtin list to keep all your nodes."""def __init__(self):self.head = None # The head of your list, don't change its name. It shouldbe "None" when the list is empty.def append(self, num): # append num to the tail of the listpassdef insert(self, index, num): # insert num into the given indexpassdef delete(self, index): # remove the node at the given index and return the deleted value as an integerpassdef circularize(self): # Make your list circular.…arrow_forwardJAVA PROGRAM MODIFY THIS PROGRAM SO IT READS THE TEXT FILES IN HYPERGRADE. I HAVE PROVIDED THE INPUTS AND THE FAILED TEST CASE AS A SCREENSHOT. HERE IS THE WORKING CODE TO MODIFY: import java.io.*;import java.util.*;public class NameSearcher { private static List<String> loadFileToList(String filename) throws FileNotFoundException { List<String> namesList = new ArrayList<>(); File file = new File(filename); if (!file.exists()) { throw new FileNotFoundException(filename); } try (Scanner scanner = new Scanner(file)) { while (scanner.hasNextLine()) { String line = scanner.nextLine().trim(); String[] names = line.split("\\s+"); for (String name : names) { namesList.add(name.toLowerCase()); } } } return namesList; } private static Integer searchNameInList(String name, List<String> namesList) {…arrow_forward
- 1. Show the ListStackADT<T> interface 2. Create a ListStackDataStrucClass<T> with the following methods: defaultconstructor, overloaded constructor, copy constructor, getTop, setTop,isEmpty, ifEmpty (if empty throw the exception), push, peek, pop, toString. 3. Create a private inner class of ListStack<T> called StackNode<T> with thefollowing methods: default constructor, overloaded constructor, copyconstructor, getValue, getNext, setValue, setNext 4. Create a BaseConverter class (non-generic) with the following methods: defaultconstructor, inputPrompt, convert [converts a BaseNumber to a convertedString], convertAll [instantiate a String object] , toString, processAndPrint 5. Create a private inner class BaseNumber. The inner class has the following methods: default constructor, overloaded constructor, getNumber, getBase,setNumber, setBase. [Make your private instance variables in the inner classLong type]. 6. Create a BaseConverterDemo class that only…arrow_forwardThe program inserts a number of values into a priority queue and then removes them. The priority queue treats a larger unsigned integer as a higher priority than a smaller value. The priority queue object keeps a count of the basic operations performed during its lifetime to date, and that count is available via an accessor. In one or two places I have put statements such as op_count++;, but these are incomplete. We only count operations involved in inserting and removing elements, not auxiliary operations such as is_empty() or size() The class implements a priority queue with an unsorted vector. Your assignment is to re-implement the priority queue implementation using a heap built on a vector, exactly as we discussed in class. Leave the framework and public method interfaces completely unchanged. My original test program must work with your new class. You will have to write new private methods bubble_up and percolate_down. You should not implement heapify or heapsort. #include…arrow_forwardExplain why the code below does not run when using the test driver shown in screen shot. i also included the LLNode class and collectioninterface interface public class LinkedCollection<T> implements CollectionInterface<T> { protected LLNode<T> head; // head of the linked list protected int numElements = 0; // number of elements in this collection // set by find method protected boolean found; // true if target found, else false protected LLNode<T> location; // node containing target, if found protected LLNode<T> previous; // node preceding location public LinkedCollection() { numElements = 0; head = null; } public boolean add(T element) // Adds element to this collection. { LLNode<T> newNode = new LLNode<>(element); newNode.setLink(head); head = newNode; numElements++; return true; } protected void find(T target) // Searches the collection for an…arrow_forward
- You will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forwardA) Write a generic Java queue class (a plain queue, not a priority queue). Then, call it GenericQueue, because the JDK already has an interface called Queue. This class must be able to create a queue of objects of any reference type. Consider the GenericStack class shown below for some hints. Like the Stack class below, the GenericQueue should use an underlying ArrayList<E>. Write these methods and any others you find useful: enqueue() adds an E to the queue peek() returns a reference to the object that has been in the queue the longest, without removing it from the queue dequeue() returns the E that has been in the queue the longest, and removes it from the queue contains(T t) returns true if the queue contains at least one object that is equal to t *in the sense that calling .equals() on the object with t the parameter returns true.* Otherwise contains returns false. size() and isEmpty() are obvious.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
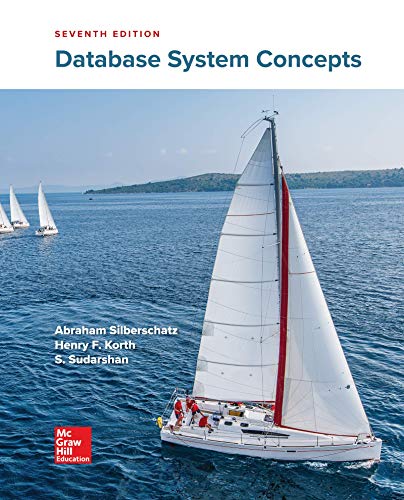
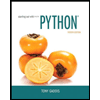
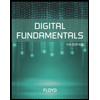
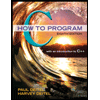
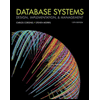
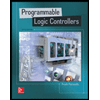