Plz I want you to rewrite this code in a good form // Include all the necessary libraries. #include #include #include #include using namespace std; class Student() { // Considering the max length of data entered (name) to // be 15. char data[15],data1[15]; int n = 0, option = 0, count_n = 0; int m = 0, count_m = 0; // This is the initial mark alloted to a subject. string empty = "00"; // Name of the file in which DB is stored. ifstream f("Example.txt"); string line; // The following for loop counts the total number of // lines in the file. for (int i = 0; std::getline(f, line); ++i) { count_n++; } while (option != 6) { // This prints out all the available options in the cout << "WELCOME TO TECHNOLOGY COLLEGE MUSCAT" cout << "\nAvailable operations: \n1. Add New " "Students\n2." << "Student Show\n3" << "6. Exit\nEnter option: "; cin >> option; if (option == 1) { insert(); } else if (option == 2) { show(); } } } void student::insert() { cout << "Enter the number of students: "; cin >> n; count_n = count_n + n; for (int i = 0; i < n; i++) { ofstream outfile; outfile.open("Example.txt", ios::app); // The entire data of a single student is // stored line-by-line. cout << "Enter your STUDENT ID: "; cin >> data; outfile << data << "\t"; cout << "Enter your STUDENT name: "; cin >> data; int len = strlen(data); cout << "Enter your STUDENT AGE: "; cin >> data; outfile << data << "\t"; while (len < 15) { data[len] = ' '; len = len + 1; } outfile << data << "\t"; outfile << empty << "\t"; outfile << empty << "\t"; } cout<<"**Student Academic Data Entry**" for ( ofstream outfile; outfile.open("Module.txt", ios::app); cout << "Enter the number of modules per student: "; cin >> m; count_m = count_m +m; for (int i = 0; i < m; i++) { cout << "Enter your module name: "; cin >> data1; outfile << data << "\t"; cout << "Enter your module marks: "; cin >> data1; int len = strlen(data1); while (len < 15) { data1[len] = ' '; len = len + 1; } outfile << data1 << "\t"; outfile << empty << "\t"; outfile << empty << "\t"; } } } } void student::display() { char regno[9]; cout << "Enter your registration number: "; cin >> regno; ifstream infile; int check = 0; infile.open("Example.txt", ios::in); // This loop prints out the data according to // the registration number specified. while (infile >> data) { if (strcmp(data, regno) == 0) { cout << "\nRegistration Number: " << data << endl; infile >> data; cout << "Name: " << data << endl; infile >> data; cout << data << endl; infile >> data; cout << data << endl; infile >> data; cout << data << endl; infile.close(); check = 1; } } if (check == 0) { cout << "No such registration number found!" << endl; } } } int main(){ Student s[10]; int n,i; clrscr(); s.insert(); s.display();
Plz I want you to rewrite this code in a good form
// Include all the necessary libraries.
#include <fstream>
#include <iostream>
#include <stdio.h>
#include <string.h>
using namespace std;
class Student()
{
// Considering the max length of data entered (name) to
// be 15.
char data[15],data1[15];
int n = 0, option = 0, count_n = 0;
int m = 0, count_m = 0;
// This is the initial mark alloted to a subject.
string empty = "00";
// Name of the file in which DB is stored.
ifstream f("Example.txt");
string line;
// The following for loop counts the total number of
// lines in the file.
for (int i = 0; std::getline(f, line); ++i) {
count_n++;
}
while (option != 6) {
// This prints out all the available options in the
cout << "WELCOME TO TECHNOLOGY COLLEGE MUSCAT"
cout << "\nAvailable operations: \n1. Add New "
"Students\n2."
<< "Student Show\n3"
<< "6. Exit\nEnter option: ";
cin >> option;
if (option == 1) {
insert();
}
else if (option == 2) {
show();
}
}
}
void student::insert()
{
cout << "Enter the number of students: ";
cin >> n;
count_n = count_n + n;
for (int i = 0; i < n; i++) {
ofstream outfile;
outfile.open("Example.txt", ios::app);
// The entire data of a single student is
// stored line-by-line.
cout << "Enter your STUDENT ID: ";
cin >> data;
outfile << data << "\t";
cout << "Enter your STUDENT name: ";
cin >> data;
int len = strlen(data);
cout << "Enter your STUDENT AGE: ";
cin >> data;
outfile << data << "\t";
while (len < 15) {
data[len] = ' ';
len = len + 1;
}
outfile << data << "\t";
outfile << empty << "\t";
outfile << empty << "\t";
}
cout<<"**Student Academic Data Entry**"
for (
ofstream outfile;
outfile.open("Module.txt", ios::app);
cout << "Enter the number of modules per student: ";
cin >> m;
count_m = count_m +m;
for (int i = 0; i < m; i++) {
cout << "Enter your module name: ";
cin >> data1;
outfile << data << "\t";
cout << "Enter your module marks: ";
cin >> data1;
int len = strlen(data1);
while (len < 15) {
data1[len] = ' ';
len = len + 1;
}
outfile << data1 << "\t";
outfile << empty << "\t";
outfile << empty << "\t";
}
}
}
}
void student::display()
{
char regno[9];
cout << "Enter your registration number: ";
cin >> regno;
ifstream infile;
int check = 0;
infile.open("Example.txt", ios::in);
// This loop prints out the data according to
// the registration number specified.
while (infile >> data) {
if (strcmp(data, regno) == 0) {
cout
<< "\nRegistration Number: " << data
<< endl;
infile >> data;
cout << "Name: " << data << endl;
infile >> data;
cout << data
<< endl;
infile >> data;
cout << data
<< endl;
infile >> data;
cout << data << endl;
infile.close();
check = 1;
}
}
if (check == 0) {
cout << "No such registration number found!"
<< endl;
}
}
}
int main(){
Student s[10];
int n,i;
clrscr();
s.insert();
s.display();

Step by step
Solved in 4 steps with 6 images

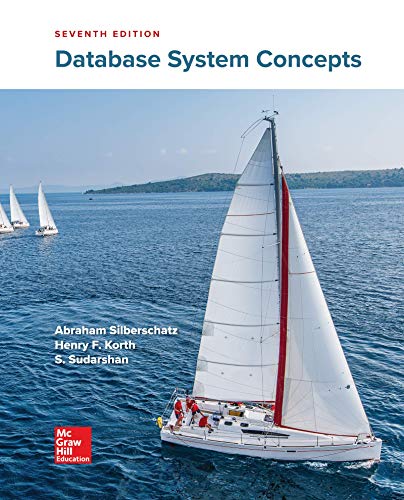
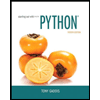
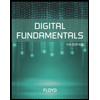
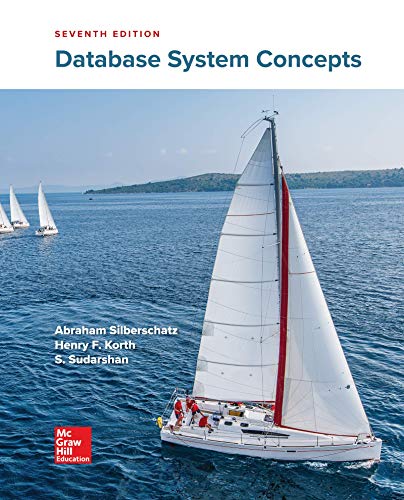
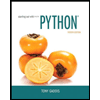
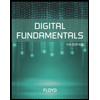
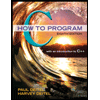
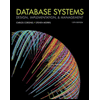
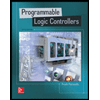