Problem: The Eight Queens problem is to find a solution to place a queen in each row on a chessboard such that no two queens can attack each other. Code below package queenchees; import static java.lang.Byte.SIZE; import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.Pane; import javafx.stage.Stage; import javafx.scene.image.Image; import javafx.scene.image.ImageView; import javafx.scene.shape.Line; public class QueenChees extends Application { // Declare a variable private static final int SIZE = 8; // Declare an array private int[] queens = new int[SIZE]; // Override start method @Override // Start method public void start(Stage primaryStage) { // Call the function search(0); // Create a chess CB ChessBoard CB = new ChessBoard(); // Create a scene Scene scene = new Scene(CB, 250,250); // Create a scene primaryStage.setTitle("Exercise18_34"); // Place the scene on the stage primaryStage.setScene(scene); // Display the stage primaryStage.show(); // Call the function CB.paint(); // SET THE width property scene.widthProperty().addListener(ov -> CB.paint()); } // Set the height property scene.heightProperty().addListener(ov -> CB.paint()); ERROR } // Function definition private boolean is_Valid(int brow, int bcolumn) ERROR { // Loop from 1 through brow for(int i=1; i<=brow; i++) // Check the column, up left diagonal and up right diagonal if(queens[brow-i]==bcolumn|| queens[brow-i]==bcolumn-i|| queens[brow-i]==bcolumn+i) ERROR // Return false return false; ERROR // Return true return true; } // Function defintion private boolean search(int brow) ERROR { // Check if the value of brow is equal to size if (brow==SIZE) // Return true return true; // Loop from 0 through size for (int bcolumn = 0; bcolumn
Problem: The Eight Queens problem is to find a solution to place a queen in each row on a chessboard such that no two queens can attack each other.
Code below
package queenchees;
import static java.lang.Byte.SIZE;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.Pane;
import javafx.stage.Stage;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.shape.Line;
public class QueenChees extends Application {
// Declare a variable
private static final int SIZE = 8;
// Declare an array
private int[] queens = new int[SIZE];
// Override start method
@Override
// Start method
public void start(Stage primaryStage)
{
// Call the function
search(0);
// Create a chess CB
ChessBoard CB = new ChessBoard();
// Create a scene
Scene scene = new Scene(CB, 250,250);
// Create a scene
primaryStage.setTitle("Exercise18_34");
// Place the scene on the stage
primaryStage.setScene(scene);
// Display the stage
primaryStage.show();
// Call the function
CB.paint();
// SET THE width property
scene.widthProperty().addListener(ov -> CB.paint());
}
// Set the height property
scene.heightProperty().addListener(ov -> CB.paint()); ERROR
}
// Function definition
private boolean is_Valid(int brow, int bcolumn) ERROR
{
// Loop from 1 through brow
for(int i=1; i<=brow; i++)
// Check the column, up left diagonal and up right diagonal
if(queens[brow-i]==bcolumn|| queens[brow-i]==bcolumn-i|| queens[brow-i]==bcolumn+i) ERROR
// Return false
return false; ERROR
// Return true
return true;
}
// Function defintion
private boolean search(int brow) ERROR
{
// Check if the value of brow is equal to size
if (brow==SIZE)
// Return true
return true;
// Loop from 0 through size
for (int bcolumn = 0; bcolumn<SIZE; bcolumn++)
{
// Place the queen
queens[brow]=bcolumn;
// Check if the block is valid
if(is_Valid(brow,bcolumn)&&search(brow+1))
// Return true
return true;
}
// Return false
return false;
}
// Class ChessBoard
private class ChessBoard extends Pane
{
// Get the image
Image queen_Image = new Image("queen.jpg");
// Function to paint
public void paint()
{
// Clear the pane
this.getChildren().clear();
// Loop to place the queen
for(int i=0; i<SIZE; i++)
{
// Create a image view
ImageView queen_ImageView = new ImageView(queen_Image);
// Add the image view to the pane
this.getChildren().add(queen_ImageView);
// Set thje position of the queen
int j = queens[i]; ERROR
// Properly position the queen in the blocks
queen_ImageView.setX(j*getWidth()/SIZE);
queen_ImageView.setY(j*getHeight()/SIZE);
queen_ImageView.setFitWidth(getWidth()/SIZE);
queen_ImageView.setFitHeight(getHeight()/SIZE);
}
// Loop to draw the line
for (int i = 1; i<=SIZE; i++)
{
// Create two lines
this.getChildren().add(new Line(0, i*getHeight()/SIZE, getWidth(), i*getHeight()/SIZE));
this.getChildren().add(new Line(i*getWidth()/SIZE,0, getWidth()/SIZE, getHeight()));
}
}
}
public static void main(String[] args) ERROR
{
launch(args); ERROR
} ERROR
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

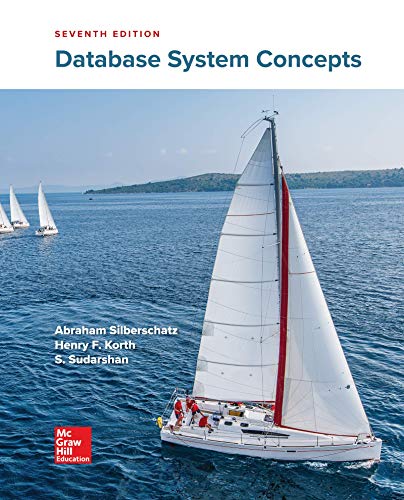
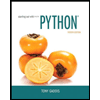
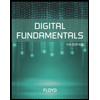
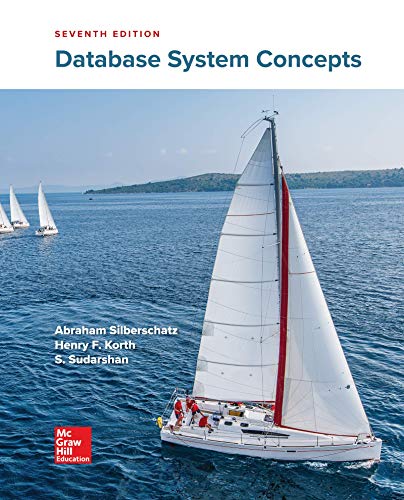
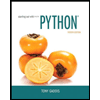
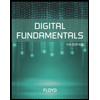
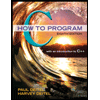
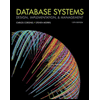
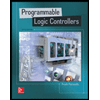